How to Merge Two Pandas Series Into a DataFrame
-
Merge Two Pandas Series Into a
DataFrame
Using thepandas.concat()
Method -
Merge Two Pandas Series Into a
DataFrame
Using thepandas.merge()
Method -
Merge Two Pandas Series Into a
DataFrame
Using theSeries.append()
Method -
Merge Two Pandas Series Into a
DataFrame
Using theDataFrame.join()
Method - Conclusion
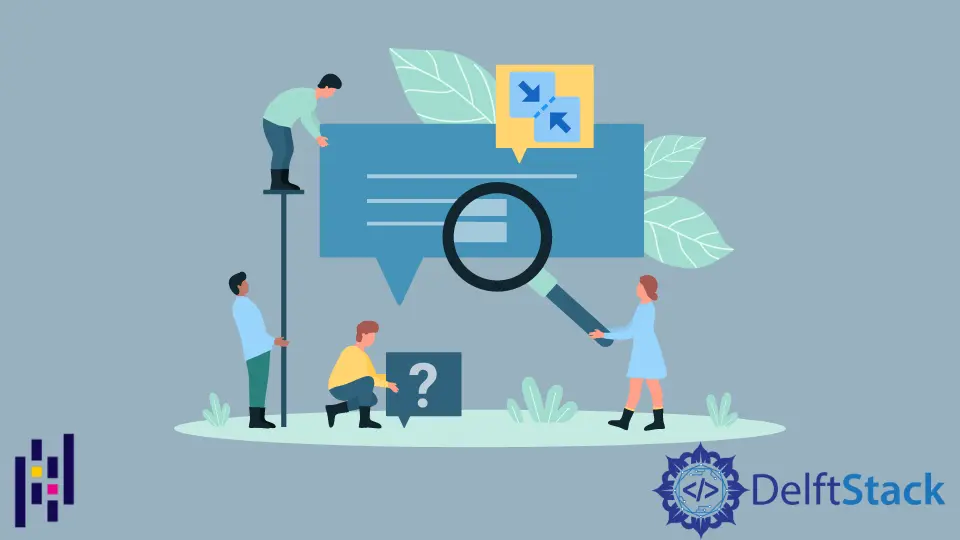
Pandas is a very popular and open-source python library that offers various functionalities or methods to merge or combine two pandas series in a DataFrame
. In pandas, a series is a single one-dimensional labeled array that can handle any data type such as integer, float, string, python objects, etc. In simple words, the pandas series is a column in an excel sheet. Series stores the data in sequential order.
This tutorial will teach us how to merge or combine two or multiple pandas series into a DataFrame
.
There are several methods available to merge two or multiple pandas series into a DataFrame
such as pandas.concat()
, Series.append()
, pandas.merge()
, and DataFrame.join()
. We will explain each method in brief detail with the help of some examples in this article.
Merge Two Pandas Series Into a DataFrame
Using the pandas.concat()
Method
The pandas.concat()
method performs all concatenations operations along an axis (either row wise or column wise). We can merge two or more pandas objects or series along a particular axis to create a DataFrame
. The concat()
method takes various parameters.
In the following example, we will pass pandas series
to merge and axis=1
as parameters. The axis=1
means the series will merge as a column instead of rows. If we use axis=0
, it will append pandas series as a row.
Example Code:
import pandas as pd
# Create Series by assigning names
products = pd.Series(
["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
name="Products",
)
dollar_price = pd.Series([350, 300, 400, 250], name="Price")
percentage_sale = pd.Series([83, 99, 84, 76], name="Sale")
# merge two pandas series using the pandas.concat() method
df = pd.concat([products, dollar_price, percentage_sale], axis=1)
print(df)
Output:
Products Price Sale
0 Intel Dell Laptops 350 83
1 HP Laptops 300 99
2 Lenavo Laptops 400 84
3 Acer Laptops 250 76
Merge Two Pandas Series Into a DataFrame
Using the pandas.merge()
Method
The pandas.merge()
is used to merge the complex column-wise combinations of DataFrame
similar to SQL join
or a merge
operation. The merge()
method can perform all database join operations between the named series objects or DataFrame
. We have to pass an extra parameter name
to the series when using the pandas.merge()
method.
See the following example.
Example Code:
import pandas as pd
# Create Series by assigning names
products = pd.Series(
["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
name="Products",
)
dollar_price = pd.Series([350, 300, 400, 250], name="Price")
# using pandas series merge()
df = pd.merge(products, dollar_price, right_index=True, left_index=True)
print(df)
Output:
Products Price
0 Intel Dell Laptops 350
1 HP Laptops 300
2 Lenavo Laptops 400
3 Acer Laptops 250
Merge Two Pandas Series Into a DataFrame
Using the Series.append()
Method
The Series.append()
method is a shortcut to the concat()
method. This method appends the series along axis=0
or rows. Using this method, we can create a DataFrame
by appending the series to another series as a row instead of columns.
We used the series.append()
method in our source code in the following way:
Example Code:
import pandas as pd
# Using Series.append()
technical = pd.Series(["Pandas", "Python", "Scala", "Hadoop"])
non_technical = pd.Series(["SEO", "Graphic design", "Content writing", "Marketing"])
# Using the `appen()` method merge series and create dataframe
df = pd.DataFrame(
technical.append(non_technical, ignore_index=True), columns=["Skills"]
)
print(df)
Output:
Skills
0 Pandas
1 Python
2 Scala
3 Hadoop
4 SEO
5 Graphic design
6 Content writing
7 Marketing
Merge Two Pandas Series Into a DataFrame
Using the DataFrame.join()
Method
Using the DataFrame.join()
method, we can join two series. When we use this method, we have to convert one series into the DataFrame
object. Then we will use the result to combine with another series.
In the following example, we have converted the first series into a DataFrame
object. Then, we used this DataFrame
to merge with another series.
Example Code:
import pandas as pd
# Create Series by assigning names
products = pd.Series(
["Intel Dell Laptops", "HP Laptops", "Lenavo Laptops", "Acer Laptops"],
name="Products",
)
dollar_price = pd.Series([350, 300, 400, 250], name="Price")
# Merge series using DataFrame.join() method
df = pd.DataFrame(products).join(dollar_price)
print(df)
Output:
Products Price
0 Intel Dell Laptops 350
1 HP Laptops 300
2 Lenavo Laptops 400
3 Acer Laptops 250
Conclusion
We learned in this tutorial how to merge two Pandas series into a DataFrame
by using the four different ways. Moreover, we explored how these four methods pandas.concat()
, Series.append()
, pandas.merge()
, and DataFrame.join()
that facilitate us to solve the Pandas merge series task.
Related Article - Pandas DataFrame
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Convert a Float to an Integer in Pandas DataFrame
- How to Sort Pandas DataFrame by One Column's Values
- How to Get the Aggregate of Pandas Group-By and Sum