Pandas Series Series.map() Function
-
Syntax of
pandas.Series.map()
-
Example Codes:
Series.map()
-
Example Codes:
Series.map()
to Pass a Dictionary asarg
Parameter -
Example Codes:
Series.map()
to Pass a Function asarg
Parameter -
Example Codes:
Series.map()
to Apply It on a DataFrame
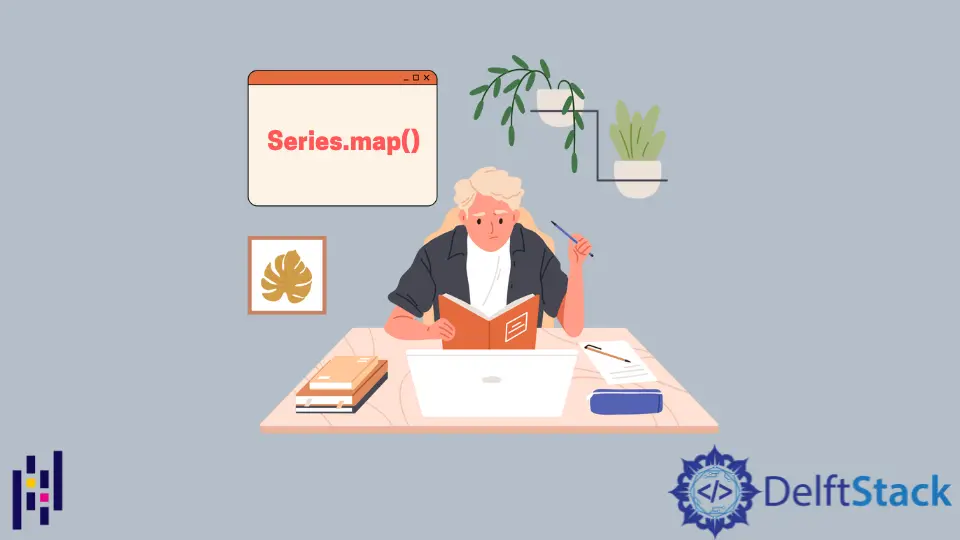
Python Pandas Series.map()
function substitutes the values of a Series
. The substituted values may be derived from a Series
, dictionary, or a function. This function works only for a Series
. If we apply this function to a DataFrame
then it will generate an AttributeError
.
Syntax of pandas.Series.map()
Series.map(arg, na_action=None)
Parameters
arg |
It is a function, dictionary, or a Series . The values to be substituted are derived from this function, dictionary, or Series . |
na_action |
This parameter accepts two values: None and ignore . Its default value is None . If its value is ignore then it does not map the derived values to NaN values. It ignores NaN values. |
Return
It returns a Series
with the same index as the caller.
Example Codes: Series.map()
We will generate a Series
that contains the NaN
values to check the output after passing the na_action
parameter.
import pandas as pd
import numpy as np
series = pd.Series(['Rose',
'Lili',
'Tulip',
np.NaN,
'Orchid',
'Hibiscus',
'Jasmine',
'Daffodil',
np.NaN ,
'SunFlower',
'Daisy'])
print(series)
The example Series
is,
0 Rose
1 Lili
2 Tulip
3 NaN
4 Orchid
5 Hibiscus
6 Jasmine
7 Daffodil
8 NaN
9 SunFlower
10 Daisy
dtype: object
We use NumPy
to generate NaN
values.
The parameter arg
is a mandatory parameter. If it is not passed then the function generates a TypeError
. We will first pass a Series as arg
parameter.
To map two Series the last column of the first Series should be the same as the index of the second Series.
import pandas as pd
import numpy as np
first_series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
second_series = pd.Series(
[23, 34, 67, 90, 21, 45, 29, 70, 56],
index=[
"Rose",
"Lili",
"Tulip",
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
"SunFlower",
"Daisy",
],
)
series1 = first_series.map(second_series)
print(series1)
Output:
0 23.0
1 34.0
2 67.0
3 NaN
4 90.0
5 21.0
6 45.0
7 29.0
8 NaN
9 70.0
10 56.0
dtype: float64
Note that the function has substituted the values after comparing the two Series
.
Example Codes: Series.map()
to Pass a Dictionary as arg
Parameter
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
dictionary = {
"Rose": "One",
"Lili": "Two",
"Orchid": "Three",
"Jasmine": "Four",
"Daisy": "Five",
}
series1 = series.map(dictionary)
print(series1)
Output:
0 One
1 Two
2 NaN
3 NaN
4 Three
5 NaN
6 Four
7 NaN
8 NaN
9 NaN
10 Five
dtype: object
The values in the Series
that are not in the dictionary are replaced with a NaN
value.
Example Codes: Series.map()
to Pass a Function as arg
Parameter
Now we will pass a function as a parameter.
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format)
print(series1)
Output:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 The name of the flower is nan.
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 The name of the flower is nan.
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
Here, we have passed string.format()
function as a parameter. Note that the function has been applied to the NaN
values as well. If we do not want to apply this function to the NaN values then we will pass ignore
value to the na_action
parameter.
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format, na_action="ignore")
print(series1)
Output:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 NaN
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 NaN
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
The above example code has ignored the NaN
values.
Example Codes: Series.map()
to Apply It on a DataFrame
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.map("The flower name is {}.".format)
print(dataframe1)
Output:
AttributeError: 'DataFrame' object has no attribute 'map'
The function has generated the AttributeError
.