Funzione Pandas Series.map()
-
Sintassi di
pandas.Series.map()
-
Codici di esempio:
Series.map()
-
Codici di esempio:
Series.map()
per passare un dizionario come parametroarg
-
Codici di esempio:
Series.map()
per passare una funzione come parametroarg
-
Codici di esempio:
Series.map()
per applicarlo su un DataFrame
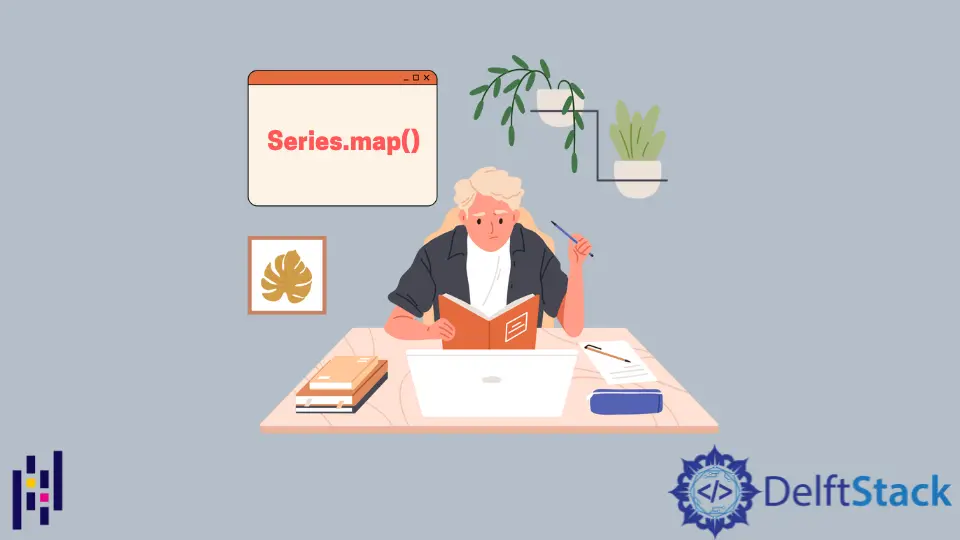
La funzione Python Pandas Series.map()
sostituisce i valori di una Series
. I valori sostituiti possono essere derivati da una Series
, da un dizionario o da una funzione. Questa funzione funziona solo per una serie
. Se applichiamo questa funzione a un DataFrame
, verrà generato un AttributeError
.
Sintassi di pandas.Series.map()
Series.map(arg, na_action=None)
Parametri
arg |
È una funzione, un dizionario o una Series . I valori da sostituire derivano da questa funzione, dizionario o Series . |
na_action |
Questo parametro accetta due valori: None e ignore . Il suo valore predefinito è None . Se il suo valore è ignore , non mappa i valori derivati ai valori NaN. Ignora i valori NaN . |
Ritorno
Restituisce una Series
con lo stesso indice del chiamante.
Codici di esempio: Series.map()
Genereremo una Series
che contiene i valori NaN
per controllare l’output dopo aver passato il parametro na_action
.
import pandas as pd
import numpy as np
series = pd.Series(['Rose',
'Lili',
'Tulip',
np.NaN,
'Orchid',
'Hibiscus',
'Jasmine',
'Daffodil',
np.NaN ,
'SunFlower',
'Daisy'])
print(series)
L’esempio “Serie” è,
0 Rose
1 Lili
2 Tulip
3 NaN
4 Orchid
5 Hibiscus
6 Jasmine
7 Daffodil
8 NaN
9 SunFlower
10 Daisy
dtype: object
Usiamo NumPy
per generare valori NaN
.
Il parametro arg
è un parametro obbligatorio. Se non viene passato, la funzione genera un TypeError
. Per prima cosa passeremo una serie come parametro arg
.
Per mappare due serie, l’ultima colonna della prima serie dovrebbe essere uguale all’indice della seconda serie.
import pandas as pd
import numpy as np
first_series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
second_series = pd.Series(
[23, 34, 67, 90, 21, 45, 29, 70, 56],
index=[
"Rose",
"Lili",
"Tulip",
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
"SunFlower",
"Daisy",
],
)
series1 = first_series.map(second_series)
print(series1)
Produzione:
0 23.0
1 34.0
2 67.0
3 NaN
4 90.0
5 21.0
6 45.0
7 29.0
8 NaN
9 70.0
10 56.0
dtype: float64
Notare che la funzione ha sostituito i valori dopo aver confrontato le due Series
.
Codici di esempio: Series.map()
per passare un dizionario come parametro arg
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
dictionary = {
"Rose": "One",
"Lili": "Two",
"Orchid": "Three",
"Jasmine": "Four",
"Daisy": "Five",
}
series1 = series.map(dictionary)
print(series1)
Produzione:
0 One
1 Two
2 NaN
3 NaN
4 Three
5 NaN
6 Four
7 NaN
8 NaN
9 NaN
10 Five
dtype: object
I valori nella Series
che non sono nel dizionario vengono sostituiti con un valore NaN
.
Codici di esempio: Series.map()
per passare una funzione come parametro arg
Ora passeremo una funzione come parametro.
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format)
print(series1)
Produzione:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 The name of the flower is nan.
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 The name of the flower is nan.
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
Qui, abbiamo passato la funzione string.format()
come parametro. Notare che la funzione è stata applicata anche ai valori NaN
. Se non vogliamo applicare questa funzione ai valori NaN, passeremo il valore ignore
al parametro na_action
.
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format, na_action="ignore")
print(series1)
Produzione:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 NaN
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 NaN
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
Il codice di esempio sopra ha ignorato i valori NaN
.
Codici di esempio: Series.map()
per applicarlo su un DataFrame
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.map("The flower name is {}.".format)
print(dataframe1)
Produzione:
AttributeError: 'DataFrame' object has no attribute 'map'
La funzione ha generato l ‘AttributeError
.