Pandas Series Series.map() 함수
Minahil Noor
2023년1월30일
Pandas
Pandas Series
-
pandas.Series.map()
구문 -
예제 코드:
Series.map()
-
예제 코드: 사전을
arg
매개 변수로 전달하는Series.map()
-
예제 코드: 함수를
arg
매개 변수로 전달하는Series.map()
-
예제 코드:
Series.map()
을 DataFrame에 적용
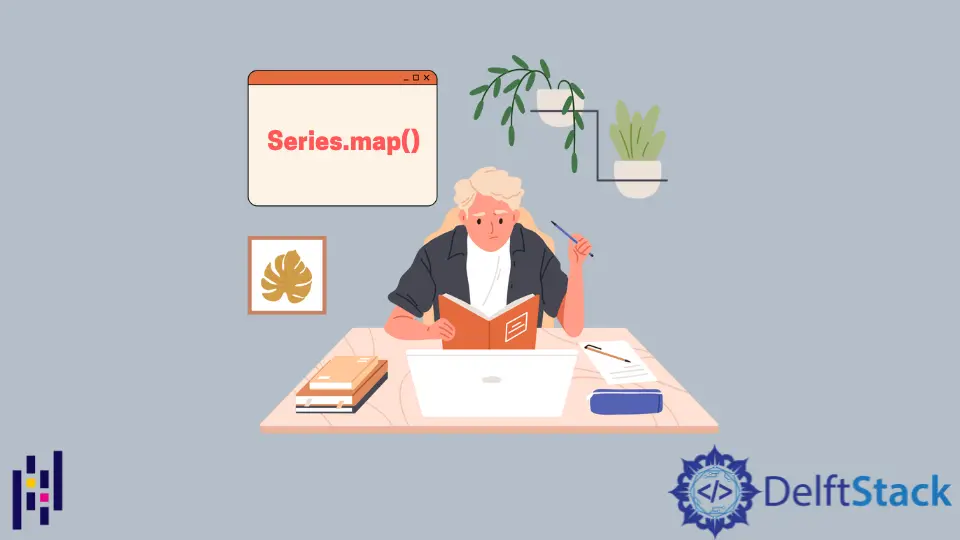
Python Pandas Series.map()
함수는Series
의 값을 대체합니다. 대체 값은 Series
, 사전 또는 함수에서 파생 될 수 있습니다. 이 기능은 Series
에서만 작동합니다. 이 함수를DataFrame
에 적용하면AttributeError
가 생성됩니다.
pandas.Series.map()
구문
Series.map(arg, na_action=None)
매개 변수
arg |
함수, 사전또는 Series 입니다. 대체 할 값은이 함수, 사전 또는 Series 에서 파생됩니다. |
na_action |
이 매개 변수는 None 및 ignore 의 두 가지 값을 허용합니다. 기본값은 None 입니다. 값이 ignore 이면 파생 된 값을 NaN 값에 매핑하지 않습니다. NaN 값을 무시합니다. |
반환
호출자와 동일한 인덱스를 가진Series
를 반환합니다.
예제 코드: Series.map()
na_action
매개 변수를 전달한 후 출력을 확인하기 위해NaN
값을 포함하는Series
를 생성합니다.
import pandas as pd
import numpy as np
series = pd.Series(['Rose',
'Lili',
'Tulip',
np.NaN,
'Orchid',
'Hibiscus',
'Jasmine',
'Daffodil',
np.NaN ,
'SunFlower',
'Daisy'])
print(series)
Series
의 예는 다음과 같습니다.
0 Rose
1 Lili
2 Tulip
3 NaN
4 Orchid
5 Hibiscus
6 Jasmine
7 Daffodil
8 NaN
9 SunFlower
10 Daisy
dtype: object
NumPy
를 사용하여NaN
값을 생성합니다.
매개 변수 arg
는 필수 매개 변수입니다. 전달되지 않으면 함수는TypeError
를 생성합니다. 먼저 Series를arg
매개 변수로 전달합니다.
두 시리즈를 매핑하려면 첫 번째 시리즈의 마지막 열이 두 번째 시리즈의 색인과 동일해야합니다.
import pandas as pd
import numpy as np
first_series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
second_series = pd.Series(
[23, 34, 67, 90, 21, 45, 29, 70, 56],
index=[
"Rose",
"Lili",
"Tulip",
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
"SunFlower",
"Daisy",
],
)
series1 = first_series.map(second_series)
print(series1)
출력:
0 23.0
1 34.0
2 67.0
3 NaN
4 90.0
5 21.0
6 45.0
7 29.0
8 NaN
9 70.0
10 56.0
dtype: float64
이 함수는 두Series
를 비교 한 후 값을 대체했습니다.
예제 코드: 사전을arg
매개 변수로 전달하는Series.map()
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
dictionary = {
"Rose": "One",
"Lili": "Two",
"Orchid": "Three",
"Jasmine": "Four",
"Daisy": "Five",
}
series1 = series.map(dictionary)
print(series1)
출력:
0 One
1 Two
2 NaN
3 NaN
4 Three
5 NaN
6 Four
7 NaN
8 NaN
9 NaN
10 Five
dtype: object
사전에없는Series
의 값은NaN
값으로 대체됩니다.
예제 코드: 함수를arg
매개 변수로 전달하는Series.map()
이제 함수를 매개 변수로 전달합니다.
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format)
print(series1)
출력:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 The name of the flower is nan.
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 The name of the flower is nan.
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
여기에서는 string.format()
함수를 매개 변수로 전달했습니다. 이 함수는NaN
값에도 적용되었습니다. 이 함수를 NaN 값에 적용하지 않으려면ignore
값을na_action
매개 변수에 전달합니다.
import pandas as pd
import numpy as np
series = pd.Series(
[
"Rose",
"Lili",
"Tulip",
np.NaN,
"Orchid",
"Hibiscus",
"Jasmine",
"Daffodil",
np.NaN,
"SunFlower",
"Daisy",
]
)
series1 = series.map("The name of the flower is {}.".format, na_action="ignore")
print(series1)
출력:
0 The name of the flower is Rose.
1 The name of the flower is Lili.
2 The name of the flower is Tulip.
3 NaN
4 The name of the flower is Orchid.
5 The name of the flower is Hibiscus.
6 The name of the flower is Jasmine.
7 The name of the flower is Daffodil.
8 NaN
9 The name of the flower is SunFlower.
10 The name of the flower is Daisy.
dtype: object
위의 예제 코드는NaN
값을 무시했습니다.
예제 코드: Series.map()
을 DataFrame에 적용
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": {0: 60, 1: 100, 2: 80, 3: 75, 4: 95},
"Name": {0: "Olivia", 1: "John", 2: "Laura", 3: "Ben", 4: "Kevin"},
"Obtained Marks": {0: 56, 1: 75, 2: 82, 3: 64, 4: 67},
}
)
dataframe1 = dataframe.map("The flower name is {}.".format)
print(dataframe1)
출력:
AttributeError: 'DataFrame' object has no attribute 'map'
이 함수는AttributeError
를 생성했습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다