How to Drop Last Row and Column in Pandas
-
Use the
drop()
Method to Drop Rows and Columns in Pandas -
Use the
drop()
Method to Drop Last Row in a Multi-Indexed Dataframe in Pandas -
Use the
drop()
Method to Delete Last Column in Pandas
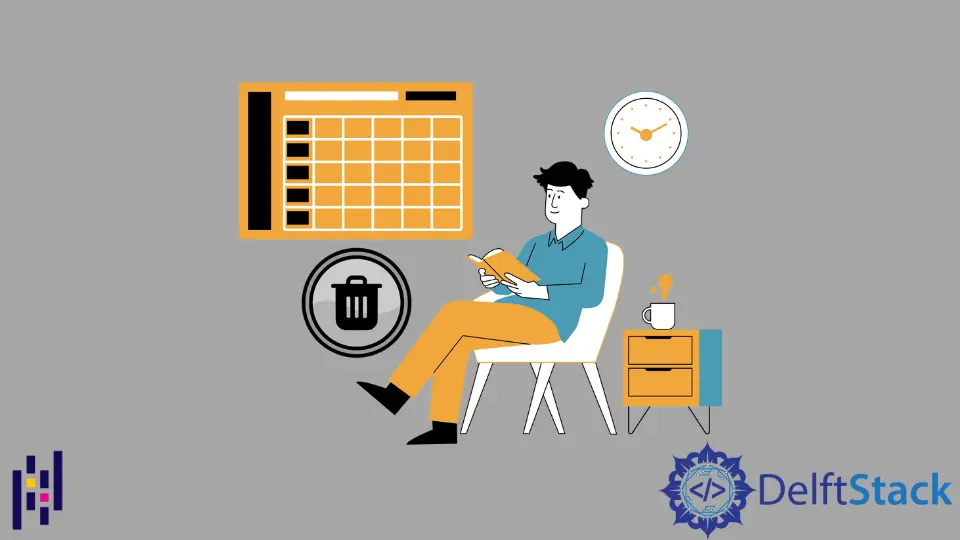
This article explores different methods to delete specific rows in Pandas data frame using Python.
Most data engineers and data analysts use Python because of its amazing ecosystem of data concentrated packages. A few of them are Pandas, Matplotlib, SciPy, etc.
Pandas is highly capable of importing various file types and exploring the data efficiently. Analysts can use the .drop()
method to delete various elements within a row and a column.
Use the drop()
Method to Drop Rows and Columns in Pandas
Syntax for drop()
:
DataFrame.drop(
labels=None,
axis=0,
index=None,
columns=None,
level=None,
inplace=False,
errors="raise",
)
Different parameters that can be used for dropping rows and columns are below.
label
- refers to thename
of a row or column.axis
- mostly integer or string value that begins from 0.index
- used as an alternative toaxis
.level
- used when the data is in multiple levels for specifying the level.inplace
- can change the data if the condition isTrue
.errors
- if the value is set toignore
, the program will ignore that specific error and execute without interruption. Also,raise
can be used as an alternative toignore
.
Let us initially make a dummy data frame in Pandas so that we use our tricks to manipulate the data and explore.
import numpy as np
import pandas as pd
df = pd.DataFrame(np.arange(12).reshape(3, 4), columns=["P", "Q", "R", "S"])
print(df)
The output for the above code is below.
P Q R S
0 0 1 2 3
1 4 5 6 7
2 8 9 10 11
As you can see, the data frame is ready to work on.
The index begins from 0, and the columns are named P
, Q
, R
, and S
. The reshape(x,y)
refers to the rows as x and columns as y.
Let us delete columns from the table using the code below.
print(df.drop(["Q", "R"], axis=1))
The output for the above code is below.
P S
0 0 3
1 4 7
2 8 11
As we can observe, columns Q
and R
have been dropped from the dataframe. The newly formed dataframe only consists of P
and S
.
Use the drop()
Method to Drop Last Row in a Multi-Indexed Dataframe in Pandas
Let us make a multi-index data frame to see how we can perform different operations on that data. Below is a code for generating dummy data that is multi-indexed.
midex = pd.MultiIndex(
levels=[["deer", "dog", "eagle"], ["speed", "weight", "length"]],
codes=[[0, 0, 0, 1, 1, 1, 2, 2, 2], [0, 1, 2, 0, 1, 2, 0, 1, 2]],
)
df = pd.DataFrame(
index=midex,
columns=["big", "small"],
data=[
[61, 36],
[29, 14],
[5.6, 2],
[43, 24],
[27, 11],
[4.5, 0.8],
[300, 250],
[3, 0.9],
[2.3, 0.3],
],
)
print(df)
The output for the code above is below.
big small
deer speed 61.0 36.0
weight 29.0 14.0
length 5.6 2.0
dog speed 43.0 24.0
weight 27.0 11.0
length 4.5 0.8
eagle speed 300.0 250.0
weight 3.0 0.9
length 2.3 0.3
As we can observe, the index for each feature here, deer
, dog
and eagle
, starts from 0, irrespective of its overall index in the table. We call it a second-level index, and the first-level index remains deer
, dog
and eagle
.
We can mention the last n
number of rows and columns that we want to delete simultaneously. For example, we can mention that we want to delete the last 2 rows or last 3 columns, and the program will do it for us instantly.
Here is an example of how we can drop the last row from the above data frame in Pandas. We will now be deleting the last 3 rows from the dummy data frame that we have created.
df.drop(df.tail(3).index, inplace=True) # drop last n rows
print(df)
Here, we have given 3
as the last n
number of rows to be deleted.
The output for the above code is below.
big small
deer speed 61.0 36.0
weight 29.0 14.0
length 5.6 2.0
dog speed 43.0 24.0
weight 27.0 11.0
length 4.5 0.8
Similarly, we can delete the columns from the data frame in the same manner.
Use the drop()
Method to Delete Last Column in Pandas
The syntax for deleting the last n
number of columns is below.
df.drop(
df.columns[
[
-n,
]
],
axis=1,
inplace=True,
)
We must replace the number of columns we need to delete with the n
given in the code above. If we desire to delete the right-most column of the data frame, we need to replace n
by 1.
The output for the above code is below.
big
deer speed 61.0
weight 29.0
length 5.6
dog speed 43.0
weight 27.0
length 4.5
So, in this way, you can perform different operations with ease by just mentioning the labels correctly or by mentioning the index of the column or row you like to delete.
Thus, using the above techniques, we can efficiently find ways to delete rows and columns from a Pandas data frame in Python.