How to Get First Row of Given Column Dataframe Pandas
-
Get the First Row of a Particular Column in DataFrame Using
Series.loc()
-
Get the First Row of a Particular Column in DataFrame Using
Series.loc()
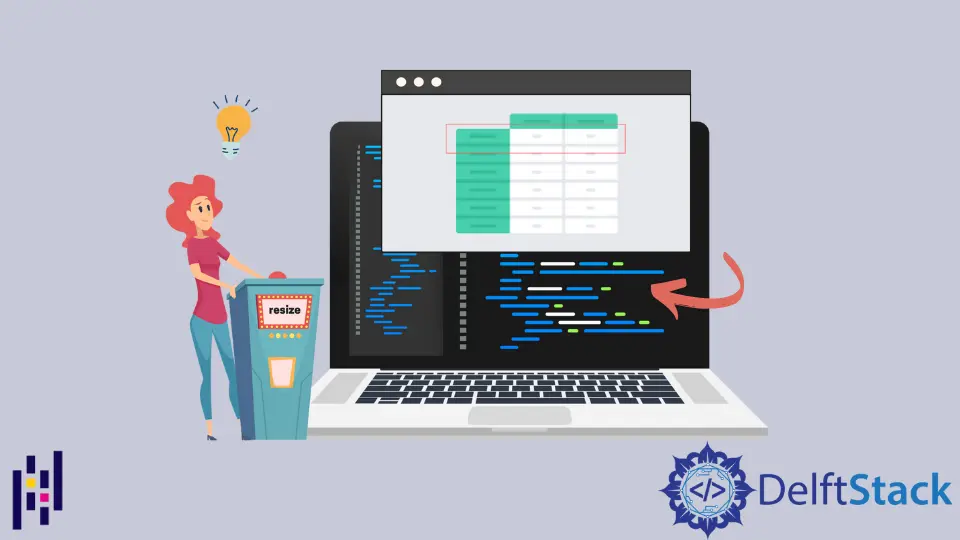
This tutorial explains how we can get the first row of a given column in DataFrame using the Series.loc()
and Series.iloc()
method.
We will use the below example DataFrame in this article.
import pandas as pd
roll_no = [501, 502, 503, 504, 505]
student_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Gender": ["Female", "Male", "Male", "Female", "Female", "Male"],
"Age": [17, 18, 17, 16, 18, 16],
},
index=roll_no,
)
print(student_df)
Output:
Name Gender Age
501 Jennifer Female 17
502 Travis Male 18
503 Bob Male 17
504 Emma Female 16
505 Luna Female 18
506 Anish Male 16
Get the First Row of a Particular Column in DataFrame Using Series.loc()
To get a particular row from a Series object using Series.loc()
, we simply pass the row’s index name as an argument to the Series.loc()
method.
Each column of DataFrame is a Series object, and we can use the .loc()
method to select any entry of the given column.
import pandas as pd
roll_no = [501, 502, 503, 504, 505, 506]
student_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Gender": ["Female", "Male", "Male", "Female", "Female", "Male"],
"Age": [17, 18, 17, 16, 18, 16],
},
index=roll_no,
)
print("The DataFrame is:")
print(student_df, "\n")
first_row = student_df["Name"].loc[501]
print("First row from Name column is:")
print(first_row)
Output:
The DataFrame is:
Name Gender Age
501 Jennifer Female 17
502 Travis Male 18
503 Bob Male 17
504 Emma Female 16
505 Luna Female 18
506 Anish Male 16
First row from Name column is:
Jennifer
It selects the first row from the Name
column of the DataFrame student_df
and prints it. We pass the index of the first row i.e. 501
to select the first row.
Alternatively, we can pass both index of the first row and the specified column’s name as arguments to the loc()
method to extract the entry at the first row of the specified column in the DataFrame.
import pandas as pd
roll_no = [501, 502, 503, 504, 505, 506]
student_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Gender": ["Female", "Male", "Male", "Female", "Female", "Male"],
"Age": [17, 18, 17, 16, 18, 16],
},
index=roll_no,
)
print("The DataFrame is:")
print(student_df, "\n")
first_name = student_df.loc[501, "Name"]
print("First row from Name column is:")
print(first_name)
Output:
The DataFrame is:
Name Gender Age
501 Jennifer Female 17
502 Travis Male 18
503 Bob Male 17
504 Emma Female 16
505 Luna Female 18
506 Anish Male 16
First row from Name column is:
Jennifer
It selects the value from the Name
column and first row with index value 503
.
Get the First Row of a Particular Column in DataFrame Using Series.loc()
To get a particular row from DataFrame using Series.iloc()
, we pass the row’s integer index as an argument to the Series.iloc()
method.
import pandas as pd
roll_no = [501, 502, 503, 504, 505, 506]
student_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Gender": ["Female", "Male", "Male", "Female", "Female", "Male"],
"Age": [17, 18, 17, 16, 18, 16],
},
index=roll_no,
)
print("The DataFrame is:")
print(student_df, "\n")
first_row = student_df["Name"].iloc[0]
print("First row from Name column is:")
print(first_row)
Output:
The DataFrame is:
Name Gender Age
501 Jennifer Female 17
502 Travis Male 18
503 Bob Male 17
504 Emma Female 16
505 Luna Female 18
506 Anish Male 16
First row from Name column is:
Jennifer
It selects the first row from the Name
column of the DataFrame student_df
and prints it. We pass the integer index of the first row i.e. 0
, since the index starts from 0
.
Alternatively, we can pass both the integer index of the first row and index of the specified column as arguments to the iloc()
method to extract the entry at the first row of the specified column in the DataFrame.
import pandas as pd
roll_no = [501, 502, 503, 504, 505, 506]
student_df = pd.DataFrame(
{
"Name": ["Jennifer", "Travis", "Bob", "Emma", "Luna", "Anish"],
"Gender": ["Female", "Male", "Male", "Female", "Female", "Male"],
"Age": [17, 18, 17, 16, 18, 16],
},
index=roll_no,
)
print("The DataFrame is:")
print(student_df, "\n")
first_name = student_df.iloc[0, 0]
print("Name of student at first row is:")
print(first_name)
Output:
The DataFrame is:
Name Gender Age
501 Jennifer Female 17
502 Travis Male 18
503 Bob Male 17
504 Emma Female 16
505 Luna Female 18
506 Anish Male 16
Name of student at first row is:
Jennifer
It selects the value from the first row and first column of the DataFrame.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn