How to Explode Multiple Columns in Pandas
-
Use the
explode()
Function to Explode Multiple Columns in Pandas -
Use
Series.explode
to Explode Multiple Columns in Pandas
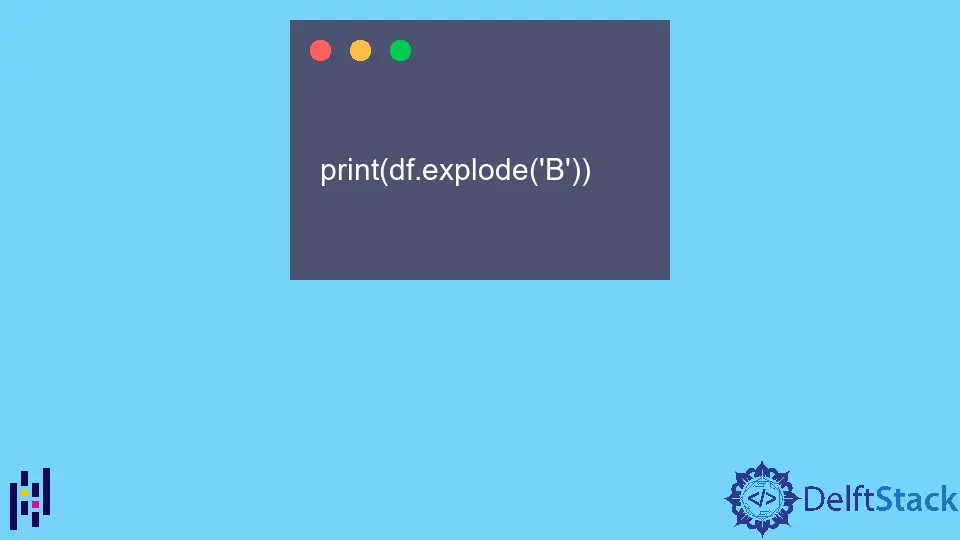
The data we have access to can contain different datatypes, from strings to arrays or lists. Typically we prefer numbers (integers and floats) and strings as there are easier to manipulate.
If we have a list within our dataframe cells, how do we work with them? We can use the explode()
function with the Pandas library, which will be explained in this topic.
Use the explode()
Function to Explode Multiple Columns in Pandas
With the explode()
function, Dataframe cells with list elements are transformed to rows while replicating the index values and returning a Dataframe with the exploded lists. Before we use the explode()
function, let’s create a Dataframe containing list elements.
Code Example:
import pandas as pd
import numpy as np
df = pd.DataFrame(
{
"A": ["1", "2", "3", "4"],
"B": [["11", "12"], ["13", "14"], ["15", "16"], ["17", "18"]],
"C": [["31", "32"], ["33", "43"], ["56", "67"], ["78", "87"]],
"D": [["41", "42"], ["34", "47"], ["55", "66"], ["77", "88"]],
"E": [["51", "52"], ["35", "45"], ["56", "76"], ["97", "68"]],
}
)
print(df)
Output:
A B C D E
0 1 [11, 12] [31, 32] [41, 42] [51, 52]
1 2 [13, 14] [33, 43] [34, 47] [35, 45]
2 3 [15, 16] [56, 67] [55, 66] [56, 76]
3 4 [17, 18] [78, 87] [77, 88] [97, 68]
To explode the Dataframe, we must pass the column
we need to explode. We can explode multiple columns, but let’s start with one column - B
.
print(df.explode("B"))
Output:
A B C D E
0 1 11 [31, 32] [41, 42] [51, 52]
0 1 12 [31, 32] [41, 42] [51, 52]
1 2 13 [33, 43] [34, 47] [35, 45]
1 2 14 [33, 43] [34, 47] [35, 45]
2 3 15 [56, 67] [55, 66] [56, 76]
2 3 16 [56, 67] [55, 66] [56, 76]
3 4 17 [78, 87] [77, 88] [97, 68]
3 4 18 [78, 87] [77, 88] [97, 68]
As you can see, it replicates the indexes and separates the list elements to have their cell. If we don’t want to use the original indexes, we can replace them with new indexes by using the ignore_index
parameter.
By setting it to True
, we replace the original indexes with new ones.
A B C D E
0 1 11 [31, 32] [41, 42] [51, 52]
1 1 12 [31, 32] [41, 42] [51, 52]
2 2 13 [33, 43] [34, 47] [35, 45]
3 2 14 [33, 43] [34, 47] [35, 45]
4 3 15 [56, 67] [55, 66] [56, 76]
5 3 16 [56, 67] [55, 66] [56, 76]
6 4 17 [78, 87] [77, 88] [97, 68]
7 4 18 [78, 87] [77, 88] [97, 68]
We can also explode the Dataframe based on multiple columns.
print(df.explode(["B", "C", "D", "E"], ignore_index=True))
Output:
A B C D E
0 1 11 31 41 51
1 1 12 32 42 52
2 2 13 33 34 35
3 2 14 43 47 45
4 3 15 56 55 56
5 3 16 67 66 76
6 4 17 78 77 97
7 4 18 87 88 68
Use Series.explode
to Explode Multiple Columns in Pandas
The Series.explode
function does the same thing that pandas explode()
function does, and we can make use of the apply()
function alongside the function to explode the entire Dataframe. We can set the index based on a column and apply the explode()
function, then reset the index using the reset_index()
function.
print(df.set_index(["A"]).apply(pd.Series.explode).reset_index())
Output:
A B C D E
0 1 11 31 41 51
1 1 12 32 42 52
2 2 13 33 34 35
3 2 14 43 47 45
4 3 15 56 55 56
5 3 16 67 66 76
6 4 17 78 77 97
7 4 18 87 88 68
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn