How to Terminate a Script in Windows PowerShell
-
Use the
Exit
Command to Terminate a Script in Windows PowerShell -
Use the
Throw
Command to Terminate a Script in Windows PowerShell -
the
Return
Command -
the
break
Command -
the
continue
Command
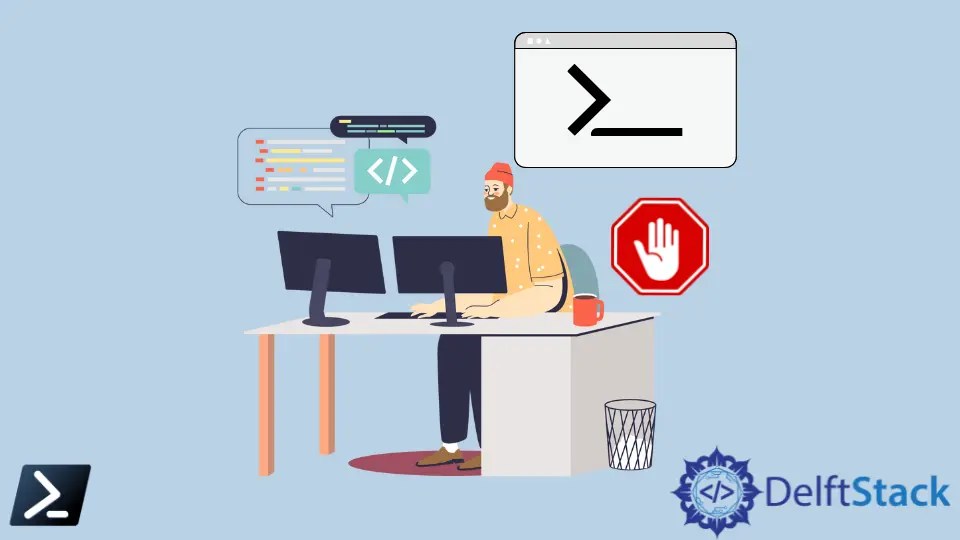
There are many ways to terminate a script in Windows PowerShell. However, some of them may sound similar in terms of context, but their actual purpose is different from each other when it comes to functionality.
This article will enumerate ways to terminate a script in Windows PowerShell and define them one by one.
Use the Exit
Command to Terminate a Script in Windows PowerShell
The Exit
command will exit your script as derived from its name. If you don’t have an open session, this command will also close your shell or scripting window. The Exit
command is also helpful for providing feedback by using exit codes.
exit
Running just the exit
command can have an exit code of 0
(default), which means success or normal termination, or 1
, which means failure or an uncaught throw.
What’s excellent about exit codes is the exit codes are fully customizable. As long as the exit code is an integer, the exit code is valid. In addition, to know the last exit code, you may output the variable $LASTEXITCODE
.
Exit.ps1
Write-Output 'Running sample script.'
exit 200
Example Code:
PS C:\>powershell.exe .\Exit.ps1
PS C:\>Running sample script.
PS C:\>Write-Output $LASTEXITCODE
PS C:\>200
Also, remember that when you call another PowerShell file with an exit
command from a running PowerShell script, the running script will also terminate.
Use the Throw
Command to Terminate a Script in Windows PowerShell
The Throw
command is similar to Exit
command with an exit code but is much more informative. You can use the command and a custom expression to generate terminating errors. Usually, the Throw
command is used inside the Catch
block inside a Try-Catch
expression to describe an exception adequately.
Example Code:
Try {
$divideAnswer = 1 / 0
}
Catch {
Throw "The mathematical expression has a syntax error"
}
Output:
The mathematical expression has a syntax error
At line:4 char:5
+ Throw "The mathematical expression has a syntax error"
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : OperationStopped: (The mathematica... a syntax error:String) [], RuntimeException
+ FullyQualifiedErrorId : The mathematical expression has a syntax error
the Return
Command
Unlike the Exit
command, the Return
command will return to its previous calling point and will not close your scripting window.
Usually, we use the Return
command to return values from an executed function somewhere in the script.
Example Code:
Function sumValues($int1, $int2) {
Return ($int1 + $int2)
}
# The function sumValues is called below, and the script will return to
# the same line with a value and store it in the output variable
$output = sumValues 1 2
Write-Output $output
Output:
3
the break
Command
We use the break
command to terminate loops and cases.
Example Code:
$numList = 1, 2, 3, 4, 5, 6, 7, 8
foreach ($number in $numList) {
if ($number -eq 8) {
#Terminate loop if number variable is equal to 8
break
}
Write-Output $number
}
Output:
1
2
3
4
5
6
7
If we have nested loops, we only break from the loop from where the break
command is called.
Example Code:
While ($true) {
While ($true) {
#The break command will only break out of this while loop
break
}
#The script will continue to run on this line after breaking out of the inner while loop
}
If you want to break out of a specific nested loop, the break
command uses labels as its parameter.
While ($true) {
:thisLoop While ($true) {
While ($true) {
#The break command below will break out of the `thisLoop` while loop.
Break thisLoop
}
}
}
the continue
Command
The continue
command also terminates the script on the loop level. Still, instead of terminating the whole loop instantly, the continue
command only terminates the current iteration and keeps the loop going until all iterations have been processed.
We can say that this is a command to skip something when executing a loop.
Example Code:
$numList = 1, 2, 3, 4, 5, 6, 7, 8
foreach ($number in $numList) {
if ($number -eq 2) {
#The continue command below will skip number 2 and will directly process the next iteration, resetting the process to the top of the loop.
continue
}
Write-Output $number
}
Output:
1
3
4
5
6
7
8
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell Script
- How to Get-Acl for a Specific User in PowerShell
- How to Manually Stop a Long Script Execution in PowerShell
- How to Convert PowerShell File to an Executable File
- How to Get the File System Location of a PowerShell Script
- How to Pass an Argument to a PowerShell Script
- How to Run a PowerShell Script From a Batch File