Windows PowerShell でスクリプトを終了する方法
-
Windows PowerShell でスクリプトを終了するために
Exit
コマンドを使用する -
Windows PowerShell でスクリプトを終了するために
Throw
コマンドを使用する -
Return
コマンド -
break
コマンド -
continue
コマンド
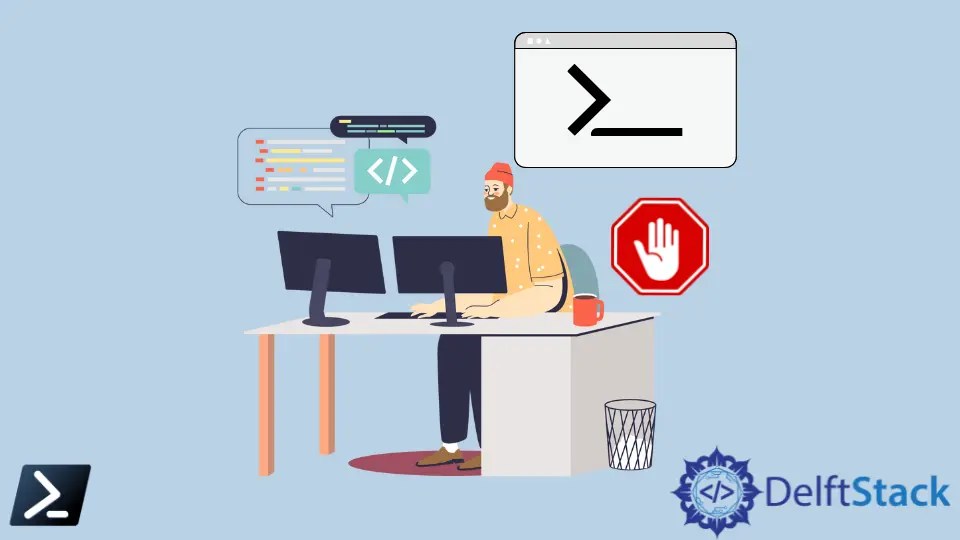
Windows PowerShell でスクリプトを終了する方法はいくつかあります。ただし、文脈においては似ているように聞こえるかもしれませんが、機能に関してはそれぞれ実際の目的が異なります。
この記事では、Windows PowerShell でスクリプトを終了する方法を列挙し、一つずつ定義します。
Windows PowerShell でスクリプトを終了するために Exit
コマンドを使用する
Exit
コマンドは、その名前に由来してスクリプトを終了します。オープンセッションがない場合、このコマンドはシェルまたはスクリプトウィンドウも閉じます。Exit
コマンドは、終了コードを使用してフィードバックを提供するのにも役立ちます。
exit
単に exit
コマンドを実行すると、終了コードは 0
(デフォルト)になります。これは成功または通常の終了を意味し、1
は失敗または未捕捉のスローを意味します。
終了コードの素晴らしい点は、それらが完全にカスタマイズ可能であることです。終了コードが整数である限り、それは有効です。また、最後の終了コードを知るためには、変数 $LASTEXITCODE
を出力することができます。
Exit.ps1
Write-Output 'Running sample script.'
exit 200
例コード:
PS C:\>powershell.exe .\Exit.ps1
PS C:\>Running sample script.
PS C:\>Write-Output $LASTEXITCODE
PS C:\>200
また、実行中の PowerShell スクリプトから exit
コマンドを使用して別の PowerShell ファイルを呼び出すと、実行中のスクリプトも終了することを思い出してください。
Windows PowerShell でスクリプトを終了するために Throw
コマンドを使用する
Throw
コマンドは終了コードを持つ Exit
コマンドに似ていますが、はるかに情報が豊富です。コマンドとカスタム式を使用して、終了エラーを生成できます。通常、Throw
コマンドは Try-Catch
式内の Catch
ブロック内で使用され、例外を適切に説明します。
例コード:
Try {
$divideAnswer = 1 / 0
}
Catch {
Throw "The mathematical expression has a syntax error"
}
出力:
The mathematical expression has a syntax error
At line:4 char:5
+ Throw "The mathematical expression has a syntax error"
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : OperationStopped: (The mathematica... a syntax error:String) [], RuntimeException
+ FullyQualifiedErrorId : The mathematical expression has a syntax error
Return
コマンド
Exit
コマンドとは異なり、Return
コマンドは以前の呼び出しポイントに戻り、スクリプトウィンドウは閉じません。
通常、スクリプト内のどこかで実行された関数から値を返すために Return
コマンドを使用します。
例コード:
Function sumValues($int1, $int2) {
Return ($int1 + $int2)
}
# The function sumValues is called below, and the script will return to
# the same line with a value and store it in the output variable
$output = sumValues 1 2
Write-Output $output
出力:
3
break
コマンド
break
コマンドはループやケースを終了するために使用します。
例コード:
$numList = 1, 2, 3, 4, 5, 6, 7, 8
foreach ($number in $numList) {
if ($number -eq 8) {
#Terminate loop if number variable is equal to 8
break
}
Write-Output $number
}
出力:
1
2
3
4
5
6
7
入れ子のループがある場合、break
コマンドが呼び出されたループからのみ抜けます。
例コード:
While ($true) {
While ($true) {
#The break command will only break out of this while loop
break
}
#The script will continue to run on this line after breaking out of the inner while loop
}
特定の入れ子のループから抜けたい場合、break
コマンドはラベルをパラメーターとして使用します。
While ($true) {
:thisLoop While ($true) {
While ($true) {
#The break command below will break out of the `thisLoop` while loop.
Break thisLoop
}
}
}
continue
コマンド
continue
コマンドもループレベルでスクリプトを終了します。ただし、全ループを即座に終了させるのではなく、continue
コマンドは現在の反復のみを終了させ、すべての反復が処理されるまでループを続けます。
これは、ループを実行する際に何かをスキップするためのコマンドだと言えます。
例コード:
$numList = 1, 2, 3, 4, 5, 6, 7, 8
foreach ($number in $numList) {
if ($number -eq 2) {
#The continue command below will skip number 2 and will directly process the next iteration, resetting the process to the top of the loop.
continue
}
Write-Output $number
}
出力:
1
3
4
5
6
7
8
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn