如何在 Windows PowerShell 中终止脚本
-
使用
Exit
命令终止 Windows PowerShell 中的脚本 -
使用
Throw
命令终止 Windows PowerShell 中的脚本 -
Return
命令 -
break
命令 -
continue
命令
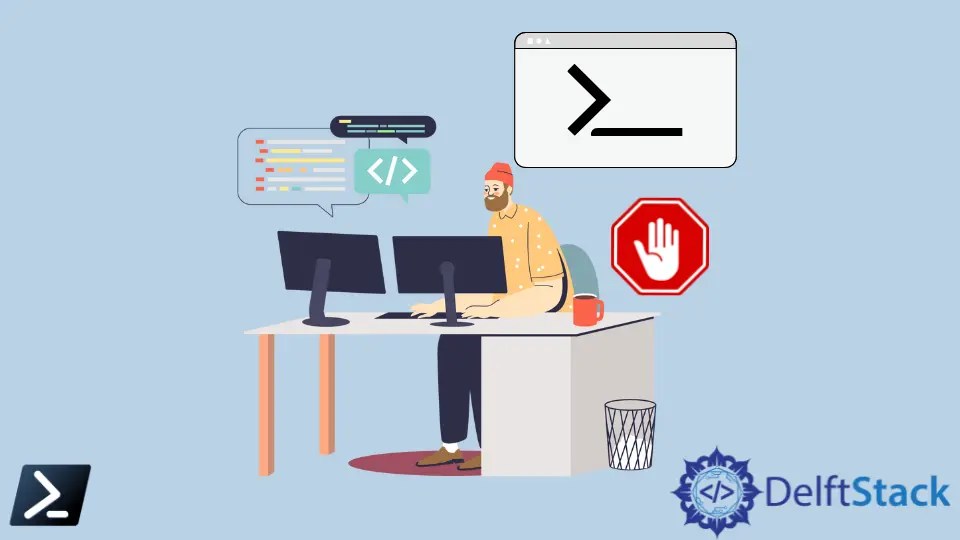
在 Windows PowerShell 中终止脚本有很多种方法。然而,尽管它们在上下文上听起来相似,但在功能上它们的实际目的却相互不同。
本文将列举在 Windows PowerShell 中终止脚本的方法,并逐一定义它们。
使用 Exit
命令终止 Windows PowerShell 中的脚本
Exit
命令将根据其名称退出您的脚本。如果您没有打开的会话,此命令还将关闭您的控制台或脚本窗口。Exit
命令对于通过使用退出代码提供反馈也很有用。
exit
仅运行 exit
命令的退出代码可以是 0
(默认),表示成功或正常终止,或 1
,表示失败或未捕获的抛出。
关于退出代码的一个优点是退出代码是完全可定制的。只要退出代码是一个整数,退出代码就是有效的。此外,要知道最后的退出代码,您可以输出变量 $LASTEXITCODE
。
Exit.ps1
Write-Output 'Running sample script.'
exit 200
示例代码:
PS C:\>powershell.exe .\Exit.ps1
PS C:\>Running sample script.
PS C:\>Write-Output $LASTEXITCODE
PS C:\>200
此外,请记住,当您从正在运行的 PowerShell 脚本中调用另一个 PowerShell 文件并使用 exit
命令时,正在运行的脚本也会终止。
使用 Throw
命令终止 Windows PowerShell 中的脚本
Throw
命令与 Exit
命令类似,带有退出代码,但信息量更大。您可以使用该命令和自定义表达式生成终止错误。通常,Throw
命令在 Try-Catch
表达式中的 Catch
块内使用,以适当地描述异常。
示例代码:
Try {
$divideAnswer = 1 / 0
}
Catch {
Throw "The mathematical expression has a syntax error"
}
输出:
The mathematical expression has a syntax error
At line:4 char:5
+ Throw "The mathematical expression has a syntax error"
+ ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : OperationStopped: (The mathematica... a syntax error:String) [], RuntimeException
+ FullyQualifiedErrorId : The mathematical expression has a syntax error
Return
命令
与 Exit
命令不同,Return
命令将返回到其先前的调用点,并不会关闭您的脚本窗口。
通常,我们使用 Return
命令从脚本中执行的某个函数返回值。
示例代码:
Function sumValues($int1, $int2) {
Return ($int1 + $int2)
}
# The function sumValues is called below, and the script will return to
# the same line with a value and store it in the output variable
$output = sumValues 1 2
Write-Output $output
输出:
3
break
命令
我们使用 break
命令终止循环和情况。
示例代码:
$numList = 1, 2, 3, 4, 5, 6, 7, 8
foreach ($number in $numList) {
if ($number -eq 8) {
#Terminate loop if number variable is equal to 8
break
}
Write-Output $number
}
输出:
1
2
3
4
5
6
7
如果我们有嵌套循环,只会从调用 break
命令的循环中跳出。
示例代码:
While ($true) {
While ($true) {
#The break command will only break out of this while loop
break
}
#The script will continue to run on this line after breaking out of the inner while loop
}
如果您想跳出特定的嵌套循环,break
命令使用标签作为参数。
While ($true) {
:thisLoop While ($true) {
While ($true) {
#The break command below will break out of the `thisLoop` while loop.
Break thisLoop
}
}
}
continue
命令
continue
命令也在循环级别终止脚本。不过,continue
命令并不是立即终止整个循环,而是仅终止当前迭代,并保持循环继续,直到所有迭代都被处理。
我们可以说这是在执行循环时跳过某些内容的命令。
示例代码:
$numList = 1, 2, 3, 4, 5, 6, 7, 8
foreach ($number in $numList) {
if ($number -eq 2) {
#The continue command below will skip number 2 and will directly process the next iteration, resetting the process to the top of the loop.
continue
}
Write-Output $number
}
输出:
1
3
4
5
6
7
8
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn