How to Read Files Line by Line in Windows PowerShell
-
Read File Line by Line in PowerShell Using
Get-Content
with aForEach
Loop -
Read File Line by Line in PowerShell Using
Get-Content
withForeach-Object
-
Using the
[System.IO.File]
Class in PowerShell to Read File Line by Line -
Using the
System.IO.StreamReader
Class in PowerShell to Read File Line by Line - Conclusion
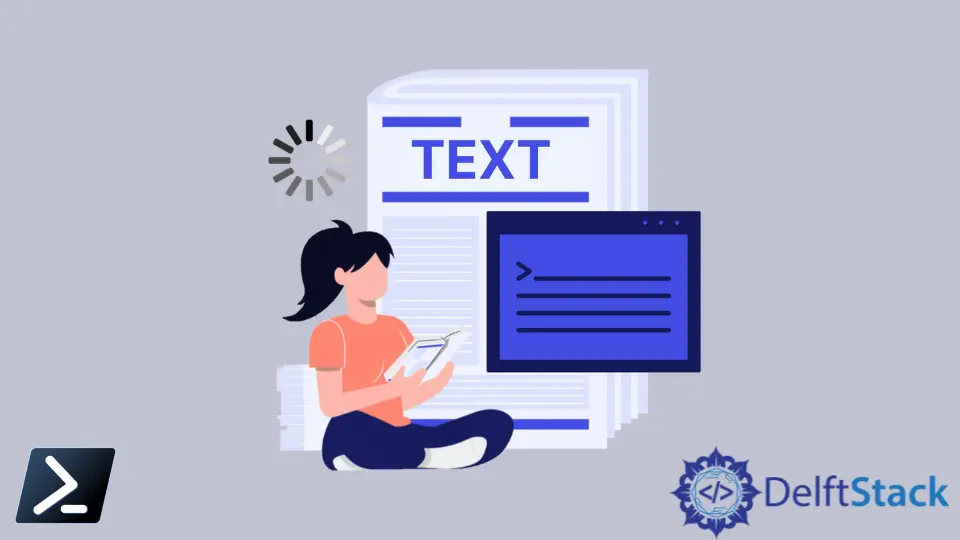
In Windows PowerShell, we can use the Get-Content
cmdlet to read files from a file. However, the cmdlet will load the entire file contents to memory at once, which will fail or freeze on large files.
To solve this problem, what we can do is we can read the files line by line, and this article will show you how.
Before we start, it is better if we create a sample text file with multiple lines. For example, I have created a file.txt
containing the following lines.
file.txt
:
one
two
three
four
five
six
seven
eight
nine
ten
According to Microsoft, a regular expression is a pattern used to match the text. It can be made up of literal characters, operators, and other constructs. This article will try different scripts involving a standard variable called Regular Expressions, denoted by $regex
.
Read File Line by Line in PowerShell Using Get-Content
with a ForEach
Loop
We have initially discussed that the Get-Content
cmdlet has its flaws when we are loading a large file as it will load the contents of the file into memory all at once. However, we can improve it by loading the lines one by one using the foreach
loop.
Example Code:
foreach ($line in Get-Content .\file.txt) {
if ($line -match $regex) {
Write-Output $line
}
}
Here’s a step-by-step explanation of the process:
-
Get the File Content:
We start by using the
Get-Content
cmdlet to read the contents of the file.Get-Content .\file.txt
Replace
"file.txt"
with the path to your file. -
Iterate Over Each Line:
Next, we use a
ForEach
loop to iterate over each line in the file. The loop assigns each line to a variable,$line
, one at a time.Inside the loop, you can replace
Write-Host $line
with the code that processes each line as per your requirements. In this example, we are simply displaying each line usingWrite-Host
, but you can perform any desired actions.
Read File Line by Line in PowerShell Using Get-Content
with Foreach-Object
To read a file line by line in PowerShell using Get-Content
with Foreach-Object
, follow these steps:
-
Use the
Get-Content
Cmdlet:We start by using the
Get-Content
cmdlet to read the contents of the file. TheGet-Content
cmdlet reads each line of the file and outputs them as objects in a pipeline.powershellCopy codeGet-Content -Path "example.txt" | ForEach-Object { # Process each line here $_ # $_ represents the current line }
Replace "example.txt"
with the path to your file.
-
Foreach-Object Loop:
Next, we use the
Foreach-Object
cmdlet (%
is an alias forForeach-Object
) to iterate over each line in the pipeline. Inside the script block (the code within{}
), you can process each line using$_
, which represents the current line being processed.powershellCopy codeGet-Content -Path "example.txt" | ForEach-Object { # Process each line here $_ # $_ represents the current line }
Within this loop, you can perform any actions you need on each line.
Complete Working Code Example
Here’s a complete working code example that reads a file line by line and displays each line:
powershellCopy code# Specify the path to the file
$filePath = "example.txt"
# Check if the file exists
if (Test-Path $filePath) {
# Read the file line by line and process each line
Get-Content -Path $filePath | ForEach-Object {
# Process each line here
Write-Host $_
}
} else {
Write-Host "File not found: $filePath"
}
In this code:
- We specify the path to the file using the
$filePath
variable. - We use the
Test-Path
cmdlet to check if the file exists. - If the file exists, we use
Get-Content
to read its content and pass it toForeach-Object
for processing. - Inside the
Foreach-Object
script block, we useWrite-Host $_
to display each line.
Using the [System.IO.File]
Class in PowerShell to Read File Line by Line
To read a file line by line in PowerShell using the [System.IO.File]
class, follow these steps:
-
Specify the File Path:
Start by specifying the path to the file you want to read. Replace
"example.txt"
with the actual path to your file.powershellCopy code $filePath = "example.txt"
-
Check If the File Exists:
Before attempting to read the file, it’s a good practice to check if the file exists using the
Test-Path
cmdlet:powershellCopy codeif (Test-Path $filePath) { # File exists, proceed with reading } else { Write-Host "File not found: $filePath" }
-
Read the File Line by Line:
Once you’ve verified that the file exists, you can use the
[System.IO.File]
class to read the file line by line. Here’s the code to do that:powershellCopy codetry { $lines = [System.IO.File]::ReadLines($filePath) foreach ($line in $lines) { # Process each line here Write-Host $line } } catch { Write-Host "Error reading the file: $_" }
In this code:
- We use the
ReadLines
method of the[System.IO.File]
class to read the file line by line, and we store the lines in the$lines
variable. - We then use a
foreach
loop to iterate over each line in$lines
and process it. In this example, we are simply displaying each line usingWrite-Host
, but you can perform any desired actions.
- We use the
Complete Working Code Example
Here’s a complete working code example that reads a file line by line and displays each line:
powershellCopy code# Specify the path to the file
$filePath = "example.txt"
# Check if the file exists
if (Test-Path $filePath) {
try {
# Read the file line by line
$lines = [System.IO.File]::ReadLines($filePath)
foreach ($line in $lines) {
# Process each line here
Write-Host $line
}
} catch {
Write-Host "Error reading the file: $_"
}
} else {
Write-Host "File not found: $filePath"
}
In this code:
- We specify the path to the file using the
$filePath
variable. - We check if the file exists using
Test-Path
. - If the file exists, we use the
[System.IO.File]::ReadLines()
method to read the file line by line and process each line using aforeach
loop. - We include error handling to catch any exceptions that might occur during file reading.
Using the System.IO.StreamReader
Class in PowerShell to Read File Line by Line
To read a file line by line in PowerShell using the System.IO.StreamReader
class, follow these steps:
-
Specify the File Path:
Begin by specifying the path to the file you want to read. Replace
"example.txt"
with the actual path to your file.powershellCopy code $filePath = "example.txt"
-
Check If the File Exists:
Before attempting to read the file, it’s a good practice to check if the file exists using the
Test-Path
cmdlet:powershellCopy codeif (Test-Path $filePath) { # File exists, proceed with reading } else { Write-Host "File not found: $filePath" }
-
Read the File Line by Line:
Once you’ve verified that the file exists, you can use the
System.IO.StreamReader
class to read the file line by line. Here’s the code to do that:
powershellCopy codetry {
$reader = [System.IO.StreamReader]::new($filePath)
while ($reader.Peek() -ge 0) {
$line = $reader.ReadLine()
# Process each line here
Write-Host $line
}
$reader.Close()
} catch {
Write-Host "Error reading the file: $_"
}
In this code:
- We create a new instance of
System.IO.StreamReader
using[System.IO.StreamReader]::new($filePath)
, where$filePath
is the path to the file. - We use a
while
loop to read the file line by line. The$reader.Peek() -ge 0
condition ensures that we continue reading until the end of the file is reached. - Inside the loop, we use
$reader.ReadLine()
to read each line and process it as needed. In this example, we are simply displaying each line usingWrite-Host
, but you can perform any desired actions. - Finally, we close the
StreamReader
using$reader.Close()
to release the file resources.
Complete Working Code Example
Here’s a complete working code example that reads a file line by line and displays each line:
powershellCopy code# Specify the path to the file
$filePath = "example.txt"
# Check if the file exists
if (Test-Path $filePath) {
try {
# Create a StreamReader to read the file
$reader = [System.IO.StreamReader]::new($filePath)
# Read the file line by line
while ($reader.Peek() -ge 0) {
$line = $reader.ReadLine()
# Process each line here
Write-Host $line
}
# Close the StreamReader
$reader.Close()
} catch {
Write-Host "Error reading the file: $_"
}
} else {
Write-Host "File not found: $filePath"
}
In this code:
- We specify the path to the file using the
$filePath
variable. - We check if the file exists using
Test-Path
. - If the file exists, we create a
System.IO.StreamReader
instance to read the file line by line and process each line using awhile
loop. - We include error handling to catch any exceptions that might occur during file reading.
Conclusion
In summary, PowerShell provides several versatile methods for reading files line by line, catering to diverse scripting and automation needs. Get-Content with a ForEach Loop is a user-friendly choice for straightforward tasks, offering simplicity and readability. Get-Content with Foreach-Object enhances flexibility, making it suitable for more intricate operations and filtering tasks within the pipeline.
For scenarios requiring greater control and comprehensive error handling, the [System.IO.File]
class offers a powerful solution. This method allows you to fine-tune file operations and gracefully handle exceptions, making it well-suited for robust and error-resistant scripts.
In situations where you’re dealing with large files and memory efficiency is crucial, the System.IO.StreamReader class shines. It allows you to read files efficiently while managing memory resources more effectively, ensuring smooth processing of substantial datasets.
Ultimately, the choice of method depends on your specific script requirements, file size, and complexity of processing. By leveraging these methods, you can confidently read files line by line in PowerShell, effectively addressing a wide range of file-related tasks in your automation workflows.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn