How to Store Text File Contents in Variable Using PowerShell
-
Use
Get-Content
to Store an Entire Text File Contents in a Variable in PowerShell -
Use
[IO.File]::ReadAllText
to Store an Entire Text File Contents in a Variable in PowerShell -
Use
[IO.File]::ReadAllLines
to Store an Entire Text File Contents in a Variable in PowerShell -
Use
StreamReader
to Store an Entire Text File Contents in a Variable in PowerShell - Conclusion
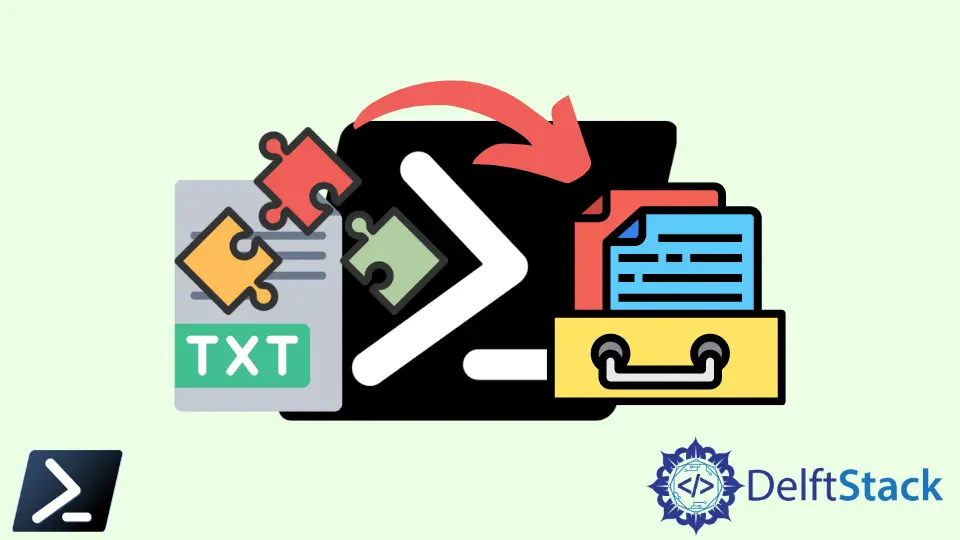
This comprehensive guide delves into various methodologies for storing the entire contents of a text file into a PowerShell variable. Each section of the article unfolds a different method, ranging from the straightforward Get-Content
cmdlet to more advanced techniques like using the .NET
Framework’s StreamReader
and File
class methods.
By exploring these diverse approaches, this article aims not only to educate beginners in the art of PowerShell scripting but also to offer seasoned scripters a deeper understanding of the versatility and power at their disposal.
We’ll dissect each method with a practical example, providing a clear understanding of the syntax, usage, and scenarios where each technique shines. This guide is a testament to PowerShell’s flexibility in handling text files, a crucial skill for any automation or scripting task in Windows environments.
Use Get-Content
to Store an Entire Text File Contents in a Variable in PowerShell
The primary purpose of using Get-Content
to read a file into a variable is to enable manipulation and analysis of the file’s contents within a PowerShell script. Applications range from simple data retrieval and display to more complex scenarios like data parsing, search, and conditional processing based on the file’s content.
Command:
# Reading content from the file
$fileContent = Get-Content -Path "example.txt"
# Displaying the content on the console
Write-Output "The content of the file is:"
Write-Output $fileContent
In this script, we begin by using Get-Content
to read the content of example.txt
. The content is then stored in the variable $fileContent
.
Following this, we use Write-Output
to display a message and the content of the file. Throughout this process, we engage in a straightforward yet effective method of file content retrieval, showcasing the simplicity and power of PowerShell scripting.
Output:
Use [IO.File]::ReadAllText
to Store an Entire Text File Contents in a Variable in PowerShell
While PowerShell’s Get-Content
cmdlet is frequently used for this purpose, another efficient approach is utilizing the [System.IO.File]::ReadAllText
method from the .NET
Framework. This method is particularly beneficial for reading the entire content of a file into a single string, making it a preferred choice for certain applications.
# Define the file path
$filePath = "example.txt"
# Use [IO.File]::ReadAllText to read the file contents
$fileContent = [System.IO.File]::ReadAllText($filePath)
# Display the content on the console
Write-Host "The content of the file is:"
Write-Host $fileContent
In our script, we start by defining the file path in the $filePath
variable. We then use [System.IO.File]::ReadAllText
to read the entire content of the file specified by $filePath
into the $fileContent
variable.
Finally, we use Write-Host
to display a message and the content of the file on the console. This script exemplifies how to efficiently and simply read file content, making it accessible for both beginners and experienced PowerShell users.
Output:
Use [IO.File]::ReadAllLines
to Store an Entire Text File Contents in a Variable in PowerShell
The [System.IO.File]::ReadAllLines
method is designed to read all lines of a text file and store them as an array of strings, with each line of the file becoming an element in the array. This approach is particularly useful when each line represents a discrete data record or entry, such as in CSV files, logs, or any structured text format where line-by-line processing is necessary.
# Define the file path
$filePath = "example.txt"
# Read all lines of the file
$lines = [System.IO.File]::ReadAllLines($filePath)
# Display each line of the file
Write-Host "The content of the file is:"
foreach ($line in $lines) {
Write-Host $line
}
In our script, we begin by specifying the path to our file in $filePath
. We then employ [System.IO.File]::ReadAllLines
to read the file’s content, storing each line as an element in the $lines
array.
Following this, we iterate over the array with a foreach
loop, using Write-Host
to display each line. This approach illustrates how to effectively read and process a text file line by line, a method that’s both efficient and easy to understand, making it suitable for a wide range of scripting scenarios.
Output:
Use StreamReader
to Store an Entire Text File Contents in a Variable in PowerShell
One sophisticated method to achieve this is by using the StreamReader
class from the .NET
Framework. The StreamReader
is particularly useful in scenarios where you need to read large files or when you want more control over the file reading process, such as customizing buffer size or handling different character encodings.
# Initialize a StreamReader for the file
$streamReader = [System.IO.StreamReader]::new("example.txt")
# Read the entire content
$fileContent = $streamReader.ReadToEnd()
# Close the StreamReader
$streamReader.Close()
# Display the content
Write-Host "The content of the file is:"
Write-Host $fileContent
In our script, we start by creating a new instance of StreamReader
for example.txt
. We then use the ReadToEnd
method to read the entire content of the file, which is stored in the $fileContent
variable.
It is crucial to close the StreamReader
using the Close
method to release the file resource. Finally, we display the file content using Write-Host
.
This script demonstrates an effective approach to reading file content, particularly useful for large files while offering an insight into handling .NET
classes within PowerShell.
Output:
Conclusion
This article has journeyed through the various methods of reading and storing text file contents in a PowerShell variable, providing a rich tableau of options for scripters of all levels. From the simplicity of Get-Content
to the robustness of [System.IO.File]::ReadAllText
, [System.IO.File]::ReadAllLines
, and the StreamReader
class, we have seen how each method serves different needs and scenarios.
Whether dealing with large files, requiring efficient memory management, or needing to process each line individually, PowerShell offers a solution. These methods highlight PowerShell’s adaptability and its seamless integration with the .NET
Framework, making it an indispensable tool for system administrators, developers, and IT professionals.