How to Loop Through a JSON File in PowerShell
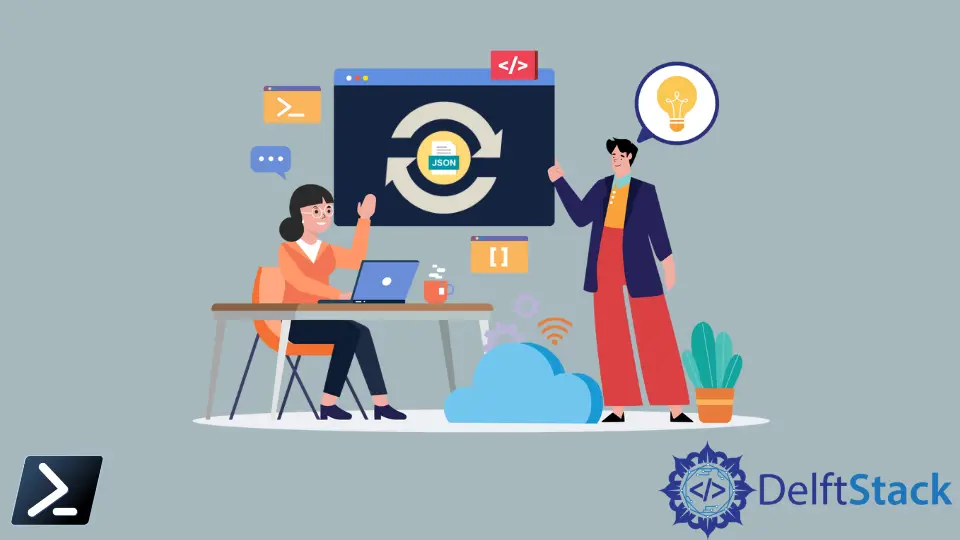
The main aim of this article is to demonstrate how to loop and iterate through a JSON file in PowerShell scripting.
PowerShell Loop Through JSON
While dealing with JSON objects while scripting in PowerShell, it can occur that to access a certain property, one may want to iterate through it.
Consider the following JSON Object:
[
{
"id": 1,
"first_name": "Twila",
"last_name": "Witcherley",
"email": "twitcherley0@issuu.com",
"gender": "Female",
"ip_address": "129.230.255.192"
}, {
"id": 2,
"first_name": "Rafaelita",
"last_name": "Fearnehough",
"email": "rfearnehough1@nsw.gov.au",
"gender": "Polygender",
"ip_address": "247.204.187.100"
}, {
"id": 3,
"first_name": "Eimile",
"last_name": "Denyer",
"email": "edenyer2@nps.gov",
"gender": "Female",
"ip_address": "125.244.213.155"
}, {
"id": 4,
"first_name": "Sly",
"last_name": "Conman",
"email": "sconman3@meetup.com",
"gender": "Polygender",
"ip_address": "194.50.217.42"
}, {
"id": 5,
"first_name": "Augustine",
"last_name": "Ciccotti",
"email": "aciccotti4@google.ca",
"gender": "Male",
"ip_address": "192.158.101.100"
}
]
In the context of the JSON mentioned above, it may be required to access the id
, first_name
, last_name
, email
, gender
and ip_address
.
Use ConvertFrom-Json
The solution can be solved in two ways, mainly based on the version of PowerShell.
Consider the following snippet:
$j =
@'
[{"id":1,"first_name":"Twila","last_name":"Witcherley","email":"twitcherley0@issuu.com","gender":"Female","ip_address":"129.230.255.192"},{"id":2,"first_name":"Rafaelita","last_name":"Fearnehough","email":"rfearnehough1@nsw.gov.au","gender":"Polygender","ip_address":"247.204.187.100"},{"id":3,"first_name":"Eimile","last_name":"Denyer","email":"edenyer2@nps.gov","gender":"Female","ip_address":"125.244.213.155"},{"id":4,"first_name":"Sly","last_name":"Conman","email":"sconman3@meetup.com","gender":"Polygender","ip_address":"194.50.217.42"},{"id":5,"first_name":"Augustine","last_name":"Ciccotti","email":"aciccotti4@google.ca","gender":"Male","ip_address":"192.158.101.100"}]
'@
foreach ($record in ($j | ConvertFrom-Json)) {
Write-Host "$($record.id) | $($record.first_name) | $($record.last_name) | $($record.email) | $($record.gender) | $($record.ip_address)"
}
Which gives the following output:
1 | Twila | Witcherley | twitcherley0@issuu.com | Female | 129.230.255.192
2 | Rafaelita | Fearnehough | rfearnehough1@nsw.gov.au | Polygender | 247.204.187.100
3 | Eimile | Denyer | edenyer2@nps.gov | Female | 125.244.213.155
4 | Sly | Conman | sconman3@meetup.com | Polygender | 194.50.217.42
5 | Augustine | Ciccotti | aciccotti4@google.ca | Male | 192.158.101.100
We can use the ConvertFrom-Json
cmdlet to convert a JSON string into a PowerShell data structure.
The reason for using ConvertFrom-JSON
is that PowerShell does not support iterating over JSON objects without first converting them to something suitable. In this case, ConvertFrom-Json
is used to convert the JSON string to a PSCustomObject
, with each of its properties representing a JSON field.
Use Deserialize Object
Consider the following snippet:
Add-Type -AssemblyName System.Web.Extensions
$JS = New-Object System.Web.Script.Serialization.JavaScriptSerializer
$j =
@'
[{"id":1,"first_name":"Twila","last_name":"Witcherley","email":"twitcherley0@issuu.com","gender":"Female","ip_address":"129.230.255.192"},{"id":2,"first_name":"Rafaelita","last_name":"Fearnehough","email":"rfearnehough1@nsw.gov.au","gender":"Polygender","ip_address":"247.204.187.100"},{"id":3,"first_name":"Eimile","last_name":"Denyer","email":"edenyer2@nps.gov","gender":"Female","ip_address":"125.244.213.155"},{"id":4,"first_name":"Sly","last_name":"Conman","email":"sconman3@meetup.com","gender":"Polygender","ip_address":"194.50.217.42"},{"id":5,"first_name":"Augustine","last_name":"Ciccotti","email":"aciccotti4@google.ca","gender":"Male","ip_address":"192.158.101.100"}]
'@
$data = $JS.DeserializeObject($j)
$data.GetEnumerator() | ForEach-Object {
foreach ($key in $_.Keys) {
Write-Host "$key : $($_[$key])"
}
}
Output:
id : 1
first_name : Twila
last_name : Witcherley
email : twitcherley0@issuu.com
gender : Female
ip_address : 129.230.255.192
id : 2
first_name : Rafaelita
last_name : Fearnehough
email : rfearnehough1@nsw.gov.au
gender : Polygender
ip_address : 247.204.187.100
id : 3
first_name : Eimile
last_name : Denyer
email : edenyer2@nps.gov
gender : Female
ip_address : 125.244.213.155
id : 4
first_name : Sly
last_name : Conman
email : sconman3@meetup.com
gender : Polygender
ip_address : 194.50.217.42
id : 5
first_name : Augustine
last_name : Ciccotti
email : aciccotti4@google.ca
gender : Male
ip_address : 192.158.101.100
To overcome this problem, we can use the .NET JavaScriptSerializer
to handle our JSON file. As the JavaScriptSerializer
returns a Dictionary
, the iteration is relatively simple using the GetEnumerator()
and foreach-Object
methods.
Remember that PowerShell 2.0 and .NET Framework 2 - 4.8
are required to reference the System.Web.UI.WebResourceAttribute
dll.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn