How to Parse JSON Files Using PowerShell
- Downloading JSON Files in PowerShell
-
Use the
Invoke-Request
Command in PowerShell -
Use the
Invoke-RestMethod
Cmdlet - Parsing JSON Files With PowerShell
-
Use the
Invoke-WebRequest
to Download the Filtered Object in PowerShell
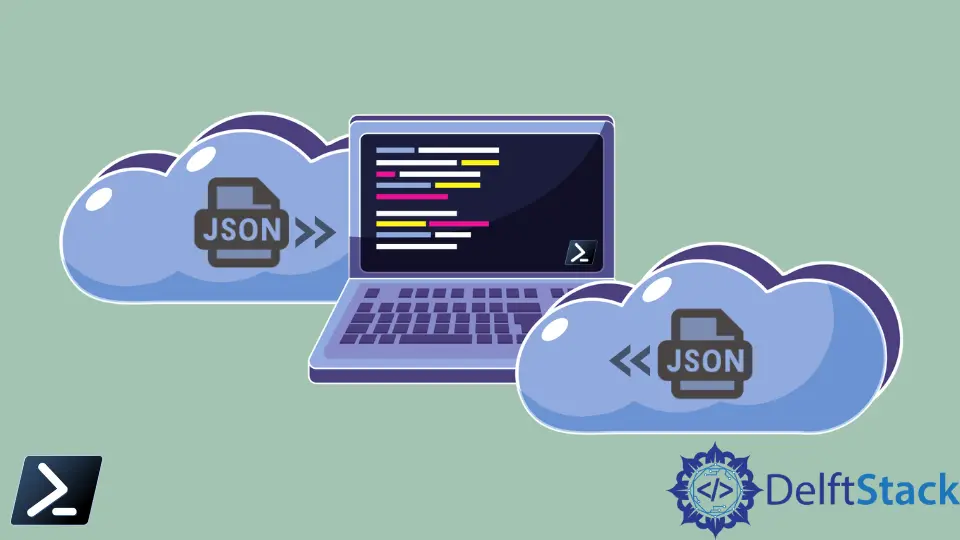
Many APIs use a protocol called REST. REST APIs
talk primarily in one language through Javascript Object Notation or simply JSON.
This article shows how to use Windows PowerShell to speak directly to a REST API
and translate the data into something useful.
JSON is a way to close the gap between human-readable and machine-readable data.
Before structured data objects, most operating systems relied on text parsing tools that used complex like regular expressions regex
to bring structure to simple text.
We can now create a string that can be read by both humans and programs with relative ease using JSON.
{
"string": "this is a string. backslashes (\\) have to be escaped",
"object": {
"description": "This is a sample object nested inside our javascript object. Description is a string subproperty"
},
"numbers": 12345,
"array": ["a string", "another string"]
}
JSON is easy to read and write, albeit with many quotations, commas, parenthesis, and escaped characters.
Downloading JSON Files in PowerShell
First, get an example JSON from GitHub into PowerShell that uses the Invoke-RestMethod
command.
However, it’s still important to know how to convert JSON strings.
Use the Invoke-Request
Command in PowerShell
To query an API with Windows PowerShell and get some JSON in return is to use the Invoke-WebRequest
command.
This command can query any web service or site over HTTP and return information (not just JSON). We will use this example to get some JSON to work with.
- Open up the PowerShell console.
- Run the
Invoke-WebRequest
command to query the GitHub API, as shown below. The code snippet below assigns all of the output the cmdlet returns to the$webData
variable for processing.
$webJSONData = Invoke-WebRequest -Uri "https://api.github.com/repos/PowerShell/PowerShell/releases/latest"
Execute the ConvertFrom-Json
command to convert the JSON string stored in the content property to a PowerShell object.
$releases = ConvertFrom-Json $webJSONData.content
Pipe the Windows PowerShell object to the Get-Member
command. When you do, we will see that the object is a System.Management.Automation.PSCustomObject
type; not just a simple string.
$releases | Get-Member
Use the Invoke-RestMethod
Cmdlet
Using the Invoke-RestMethod
command is very similar to Invoke-WebRequest
. It only eliminates a single line of code.
The cmdlet will automatically assume a JSON response and convert it for you.
It clarifies in the code that you are expecting to be using an API, and you are also expecting a JSON response.
- Open up your PowerShell Console
- Run
Invoke-RestMethod
to GET the release information
$releases_2 = Invoke-RestMethod -Uri "https://api.github.com/repos/PowerShell/PowerShell/releases/latest"
Pipe the Windows PowerShell object to the Get-Member
command. When you do, we will see that the object is a System.Management.Automation.PSCustomObject
type; not just a simple string.
$releases_2 | Get-Member
Parsing JSON Files With PowerShell
Let us look through the $releases
variable defined in the last section. Two properties that stand out are the browser_download_url
and the name
properties.
The browser_download_url
property relates to the URL, and the name
property probably relates to the release names.
Now output all of the name
properties as shown below.
$releases_2.assets.name
Use the Invoke-WebRequest
to Download the Filtered Object in PowerShell
## Using Invoke-RestMethod
$webAPIData = Invoke-RestMethod -Uri "https://api.github.com/repos/PowerShell/PowerShell/releases/latest"
## Using Invoke-WebRequest
$webAPIData = ConvertFrom-Json (Invoke-WebRequest -Uri "https://api.github.com/repos/PowerShell/PowerShell/releases/latest")
## The download information is stored in the "assets" section of the data
$assets = $webAPIData.assets
## The pipeline operator is used to filter the assets object to find the release version
$asset = $assets | Where-Object { $_.name -match "win-x64" -and $_.name -match ".zip" }
## Download the latest version
Write-Output "Downloading $($asset.name)"
Invoke-WebRequest $asset.browser_download_url -OutFile "$pwd\$($asset.name)"
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn