How to Create JSON Array in PowerShell
-
Use
ConvertTo-Json
to Create a JSON Array in PowerShell -
Use
ConvertTo-Json
to Create a JSON Array and Save It Into a File in PowerShell - Use Here-Strings to Create a JSON Array in PowerShell
- Create an Array of Custom Objects to Create a JSON Array in PowerShell
- Conclusion
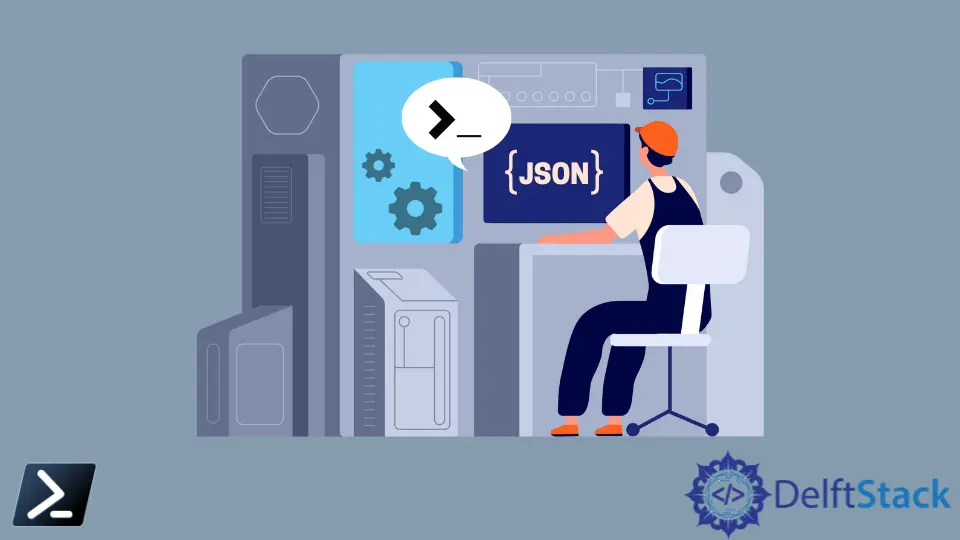
In PowerShell, JSON (JavaScript Object Notation) is a common data interchange format used for communication between various systems and applications. A JSON array containing key-value pairs is a structured way to organize data.
In this article, we’ll explore different methods to create a JSON array in PowerShell, along with detailed code examples and explanations.
Use ConvertTo-Json
to Create a JSON Array in PowerShell
In this example, we demonstrate how to create a simple JSON array using PowerShell. We first organize the JSON array inside a variable called $Body
and then convert it to a JSON array using the ConvertTo-Json
cmdlet.
Example 1:
$Body = @(
@{
FirstName ='Alen'
LastName ='Walker'
}
)
ConvertTo-Json -InputObject $Body
In this code snippet, we define an array using @()
, and within the array, we create a hashtable (@{}
) with key-value pairs representing the data elements of our JSON array. The keys in the hashtable correspond to the field names in our JSON, such as FirstName
and LastName
.
We then use ConvertTo-Json
to convert this structure into a JSON array.
The resulting JSON output can be seen below.
Output:
[
{
"LastName": "Walker",
"FirstName": "Alen"
}
]
In our next example, we showcase creating a JSON object that contains sub-arrays.
The $Body
variable is defined to hold a JSON object with two properties: My Items
and My details
. Each property contains an array of items.
Example 2:
$body = @{
'My Items' = @(
"Item 1",
"Item 2",
"Item 3",
"Item 4"
)
'My details' =@(
@{
"Name" = "Alen"
"Id" = "0001"
},
@{
"Name" = "Mycle"
"Id" = "0002"
}
)
}
ConvertTo-Json -InputObject $Body
In this script, we define a hashtable (@{}
) and specify two keys: 'My Items'
and 'My details'
. The value associated with 'My Items'
is an array of item names, while the value associated with 'My details'
is an array of hashtables representing details about each item.
When we run ConvertTo-Json
on the $Body
variable, it converts the structure into a JSON object.
The resulting JSON output can be seen below.
Output:
{
"My details": [
{
"Id": "0001",
"Name": "Alen"
},
{
"Id": "0002",
"Name": "Mycle"
}
],
"My Items": [
"Item 1",
"Item 2",
"Item 3",
"Item 4"
]
}
In this JSON object, "My details"
and "My Items"
are the keys, each associated with its respective array of data. The structure allows for organizing related data in a hierarchical manner, which is a common use case when working with JSON in PowerShell.
We can also create a PowerShell object and then convert it directly to a JSON array using the ConvertTo-Json
cmdlet.
Take a look at the example below:
$jsonArray = @(
@{
FirstName = 'John'
LastName = 'Doe'
},
@{
FirstName = 'Jane'
LastName = 'Smith'
}
)
$jsonOutput = $jsonArray | ConvertTo-Json
Write-Output $jsonOutput
Output:
[
{
"LastName": "Doe",
"FirstName": "John"
},
{
"LastName": "Smith",
"FirstName": "Jane"
}
]
Here, the example script begins by defining a variable named $jsonArray
as an array of PowerShell hash tables. Each hash table represents a person with attributes for first name (FirstName
) and last name (LastName
).
The script then converts this PowerShell array of hash tables into a JSON array using the ConvertTo-Json
cmdlet and assigns it to the $jsonOutput
variable. Finally, it uses Write-Output
to display the resulting JSON array.
In summary, the script creates a JSON array representation of two individuals with their respective first and last names using PowerShell hash tables, and then it outputs this JSON array to the console.
Use ConvertTo-Json
to Create a JSON Array and Save It Into a File in PowerShell
This method is a bit complex. We will create the JSON array step by step using more than one variable.
Please take a look at the following example to understand this method better.
Example:
$jsonBase = @{}
$array = @{}
$data = @{"Name" = "Mycle"; "Colour" = "Alen"; }
$array.Add("User", $data)
$jsonBase.Add("UserData", $array)
$jsonBase | ConvertTo-Json -Depth 10 | Out-File ".\sample.json"
At the start of the script, an empty hash table named $jsonBase
is created. This will serve as the fundamental structure for the JSON object that we will build.
Following that, another hash table named $array
is initialized to represent the array object. This $array
variable will hold the data that constitutes the array within our JSON structure.
Then, sample user data is defined using a hash table named $data
, containing key-value pairs such as the user’s name and color. This data will later be encapsulated within the array.
Next, the script adds the $data
hash table to the $array
hash table, associating it with the key User
. This establishes the representation of user-specific data within the array.
Continuing, the $array
, which now contains the user data, is added to the $jsonBase
hash table using the key UserData
. This step encapsulates the array of user data within the larger JSON structure.
Towards the end, the script converts the $jsonBase
hash table to JSON format using ConvertTo-Json
. Note that the -Depth
parameter controls the level of recursion for converting nested structures.
Finally, the Out-File
cmdlet saves the JSON data to a file named sample.json
in the current directory.
After executing, a JSON file named sample.json
will be generated, containing the user data under the key UserData
and presenting the user’s name and color within a nested structure under the key User
.
Output (sample.json
):
{
"UserData": {
"User": {
"Name": "Mycle",
"Colour": "Alen"
}
}
}
Use Here-Strings to Create a JSON Array in PowerShell
Using here-strings in PowerShell is another handy technique to construct a well-formatted JSON array while maintaining the desired layout. A here-string, denoted by @" ... "@
, allows us to define a block of text in PowerShell while preserving its formatting, including line breaks and indentation.
We can employ here-strings to create a JSON array directly as a string.
Example:
$jsonArray = @"
[
{
"FirstName": "John",
"LastName": "Doe"
},
{
"FirstName": "Jane",
"LastName": "Smith"
}
]
"@
Write-Output $jsonArray
Output:
[
{
"FirstName": "John",
"LastName": "Doe"
},
{
"FirstName": "Jane",
"LastName": "Smith"
}
]
In this example, we used a here-string (@" ... "@
), which enables the direct creation of a JSON array as a string.
Within this here-string, we defined the JSON array using the appropriate syntax:
- We used square brackets
[...]
to encapsulate the entire array. - Then, we used curly brackets
{...}
to enclose each individual JSON object.
Lastly, we displayed the JSON array using Write-Output
.
This approach streamlines the process of constructing a JSON array with the desired structure and format. As we can see, the output shows a neatly formatted JSON array that aligns with the defined structure.
Create an Array of Custom Objects to Create a JSON Array in PowerShell
Another method we can use to create a JSON array involves creating an array of custom objects, each representing an element, and then converting it to a JSON array. Custom objects are instances of the [PSCustomObject]
type that allow us to define and structure data.
[PSCustomObject]
allows us to create objects with custom properties. It’s a fundamental feature in PowerShell that facilitates the creation and manipulation of structured data within scripts and functions.
The custom properties are defined using a hashtable-like syntax where we specify property names and their corresponding values, such as the following:
[PSCustomObject]@{
Property1 = 'Value1'
Property2 = 'Value2'
}
Here’s an example:
$jsonArray = @(
[PSCustomObject]@{
FirstName = 'John'
LastName = 'Doe'
},
[PSCustomObject]@{
FirstName = 'Jane'
LastName = 'Smith'
}
)
$jsonOutput = $jsonArray | ConvertTo-Json
Write-Output $jsonOutput
Output:
[
{
"FirstName": "John",
"LastName": "Doe"
},
{
"FirstName": "Jane",
"LastName": "Smith"
}
]
In the provided example, an array named $jsonArray
is created, containing custom objects defined using the @{}
syntax. We have the properties FirstName
and LastName
and their respective values.
Then, the ConvertTo-Json
cmdlet is used to convert the array of custom objects ($jsonArray
) into a JSON array format. The resulting JSON array ($jsonOutput
) now contains each custom object as an element, with the defined properties and values represented in JSON syntax.
Finally, the JSON array is displayed using Write-Output
.
Conclusion
In conclusion, PowerShell provides multiple methods to create a JSON array, each catering to specific needs and preferences.
The ConvertTo-Json
cmdlet is a versatile tool, allowing straightforward conversion of PowerShell objects or arrays into JSON representations. Whether structuring JSON directly, using here-strings, or leveraging custom objects, PowerShell offers flexibility in generating JSON arrays for efficient data interchange and organization across various applications and systems.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn