How to Query for File's Last Write Time in PowerShell
- Query for File Properties in PowerShell
-
Convert Values to
DateTime
Formats in PowerShell - Find the Difference Between Two Dates in PowerShell
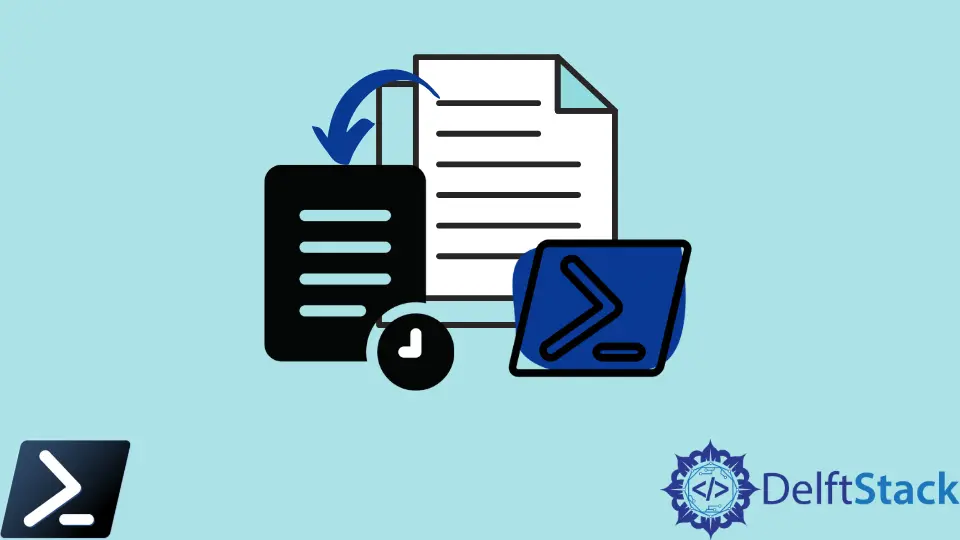
When managing files, one of our most sought properties to check is when the file was last modified. We can quickly check this conveniently through File Explorer.
However, there will be times when we want to check the properties of a file programmatically using PowerShell.
In this article, we will learn and discuss how to fetch the properties of a file, query for its last write time, and perform additional commands to get its date different from the current date.
Query for File Properties in PowerShell
With Windows PowerShell, we can easily query file properties using the Get-ItemProperty
command. Let’s use one file as an example.
Example Code:
$filepath = "C:\Temp\Login.log"
Get-ItemProperty -Path $filepath
In the example above, we have queried for the basic properties of Login.log
. The snippet above mimics how we look at file properties using the File Explorer.
Output:
Directory: C:\Temp
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 6/7/2022 4:33 AM 4944 Login.log
We could even see the Mode
property or the file permissions, the Length
or file size, and LastWriteTime
or the date and time when the file was last modified.
Now for this article, we wanted to focus more on the last write time of the file, so let’s try and isolate this property by running the updated snippet of code below.
Example Code:
$filepath = "C:\Temp\Login.log"
(Get-ItemProperty -Path $filepath -Name LastWriteTime).LastWriteTime
Output:
Tuesday, June 7, 2022 4:33:02 AM
As we can see, we have now correctly isolated the last modified time of the file; however, if we wanted to get the difference between the current time and date and the file’s previous write time, we would have an error because of their difference in data type.
Convert Values to DateTime
Formats in PowerShell
In PowerShell, we have a specific data type called DateTime
that symbolizes the system’s date and time format. Our previous output is in String
format, so let’s convert this into DateTime
format by running the code below.
Example Code:
$filepath = "C:\Temp\Login.log"
$lastWrite = [DateTime](Get-ItemProperty -Path $filepath -Name LastWriteTime).LastWriteTime
Notice that we have assigned the DateTime
value to a result. We have done this to add convenience to our script later on.
Find the Difference Between Two Dates in PowerShell
Now that our output is in DateTime
format, we can conveniently find the difference between the current and last write times by performing a simple mathematical expression.
Example Code:
$currDate = Get-Date
$currDate - $lastWrite
Output:
Days : 0
Hours : 17
Minutes : 24
Seconds : 57
Milliseconds : 757
Ticks : 626977577652
TotalDays : 0.725668492652778
TotalHours : 17.4160438236667
TotalMinutes : 1044.96262942
TotalSeconds : 62697.7577652
TotalMilliseconds : 62697757.7652
The Get-Date
command queries the current date and time during the command’s execution. The command is saved with a DateTime
data type, thus making it similar to our prepared last write time value.
With two variables with a DateTime
data type, we can find the total time and the difference using a mathematical operator like a plus (+
) and minus (-
).
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn