How to Get File Size in KB Using PowerShell
-
Use the
Get-Item
Method and theLength
Property to Get File Size in PowerShell -
Use the
Get-Item
andSelect-Object
to Get File Size in PowerShell -
Use the
Get-ChildItem
andForEach-Object
to Get File Sizes in PowerShell -
Use the
Get-ChildItem
andForEach-Object
to Get File Sizes in PowerShell - Conclusion
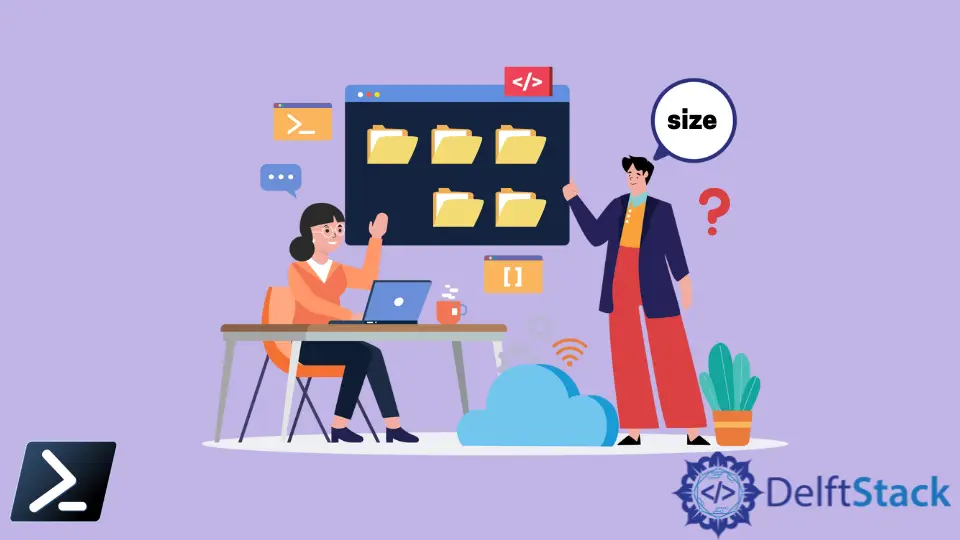
PowerShell is a powerful tool that enables you to perform different file operations in the system. You can do file tasks like creating, copying, moving, removing, reading, renaming, and checking the existence of files in PowerShell.
In Windows, you can check the file size through the GUI by right-clicking on the file icon and selecting the Properties
option. But, sometimes, you might need to check the file size from PowerShell.
This tutorial will teach you how to get the size of the file using PowerShell.
Use the Get-Item
Method and the Length
Property to Get File Size in PowerShell
The file objects have the Length
property in PowerShell. It represents the size of the file in bytes.
The following command gets the size of a train.csv
file in bytes.
# Getting the size of a file
$file = Get-Item "sample.txt"
$fileSize = $file.Length
Write-Host "The size of the file is $fileSize bytes"
Replace sample.txt
with the path to your desired file.
In our code, we start by using Get-Item
to fetch the file object for sample.txt
. Once we have the file object in $file
, we access its .Length
property to get the size of the file in bytes.
Finally, we use Write-Host
to print the size to the console. This approach is effective because it leverages the power of PowerShell’s object-oriented nature, allowing us to easily access file properties.
Output:
When working with file systems in PowerShell, it is often necessary not only to obtain the size of a file but also to present this information in a more human-readable format such as kilobytes (KB), megabytes (MB), or gigabytes (GB). PowerShell simplifies this process by allowing us to retrieve the file size in bytes using Get-Item
and then converting this value to larger units.
# Getting and converting the size of a file
$file = Get-Item "sample.txt"
$fileSizeBytes = $file.Length
$fileSizeKB = $fileSizeBytes / 1KB
$fileSizeMB = $fileSizeBytes / 1MB
$fileSizeGB = $fileSizeBytes / 1GB
Write-Host "File size: $fileSizeBytes bytes, $fileSizeKB KB, $fileSizeMB MB, $fileSizeGB GB"
Replace sample.txt
with the path to your desired file.
In this script, we begin by obtaining the file object using Get-Item
. We then access the .Length
property of this object to get the file size in bytes.
Next, we perform simple arithmetic to convert this byte value into KB, MB, and GB. Finally, we use Write-Host
to print out all these sizes in a user-friendly manner.
Output:
Use the Get-Item
and Select-Object
to Get File Size in PowerShell
One such task is retrieving the size of a file. PowerShell offers a flexible way to do this by combining Get-Item
with Select-Object
.
The combination of Get-Item
and Select-Object
is used to get file properties, including the file size. This approach is particularly useful when you need to extract specific information (like file size) in a clean, readable format.
# Getting the size of a file using Select-Object
$fileSize = (Get-Item "sample.txt" | Select-Object -ExpandProperty Length)
Write-Host "The size of the file is $fileSize bytes"
Replace sample.txt
with your file path.
In our script, we first use Get-Item
to retrieve the file object for sample.txt
. We then pipe (|
) this object to Select-Object
, specifying the -ExpandProperty Length
parameter to directly extract the file size in bytes.
By using Write-Host
, we output this information to the console. This method is streamlined and effective, especially when we’re interested in only one piece of information about the file.
Output:
Use the Get-ChildItem
and ForEach-Object
to Get File Sizes in PowerShell
The use of Get-ChildItem
in conjunction with ForEach-Object
serves to enumerate files in a directory and process each file individually to obtain its size. This approach is widely applicable in system administration for monitoring directory sizes, in data management for assessing storage requirements, and in creating detailed reports of file sizes for analysis or auditing purposes.
# Getting sizes of all files in a directory
Get-ChildItem "Directory\" | ForEach-Object {
$fileSize = $_.Length
Write-Host "$($_.Name) size: $fileSize bytes"
}
Replace Directory\
with the directory path of your choice.
In our script, Get-ChildItem
is used to retrieve all files in the Directory\
directory. Each file retrieved is then piped into ForEach-Object
.
Inside the ForEach-Object
script block, we access the Length
property of each file object ($_
) to get its size in bytes. The Write-Host
cmdlet is then used to print the name and size of each file.
This method is efficient for iterating through multiple files and obtaining their sizes individually, allowing for detailed and customizable output.
Output:
Use the Get-ChildItem
and ForEach-Object
to Get File Sizes in PowerShell
By combining PowerShell’s file manipulation cmdlets with ForEach-Object
, we can efficiently calculate the sizes of files grouped by their type. This method is especially beneficial in scenarios like storage analysis, data organization, and reporting where file types and their corresponding sizes are critical.
The primary purpose of this technique is to iterate over files in a directory, group them by their extension, and calculate the total size for each file type.
# Calculating total file sizes by file type
Get-ChildItem "Directory" -Recurse | Group-Object Extension | ForEach-Object {
$totalSize = ($_.Group | Measure-Object -Property Length -Sum).Sum
Write-Host "Total size for $($_.Name) files: $totalSize bytes"
}
Replace Directory
with the directory path of your choice.
In this script, we start by using Get-ChildItem
to get all files in the Directory
directory, including subdirectories (-Recurse
). These files are then grouped by their file extension using Group-Object
.
The ForEach-Object
cmdlet processes each group, where we calculate the total size of all files in each group using Measure-Object -Property Length -Sum
.
Finally, the total size for each file type is displayed using Write-Host
. This method is efficient for summarizing data and provides a clear insight into the distribution of file sizes by type.
Output:
Conclusion
In conclusion, this article has provided a comprehensive guide on leveraging PowerShell to perform various file size operations. We’ve explored different methods: using Get-Item
and the Length
property, Get-Item
combined with Select-Object
, and Get-ChildItem
with ForEach-Object
for more advanced scenarios like grouping file sizes by type.
Each method serves a specific purpose, from obtaining the size of a single file in a readable format to analyzing the storage distribution across different file types in a directory.
For those new to PowerShell, this tutorial offers a clear starting point for understanding file manipulation and size calculation techniques. Experienced users will appreciate the detailed examples and practical applications in system administration and data management.