How to Remove-Item if File Exists in PowerShell
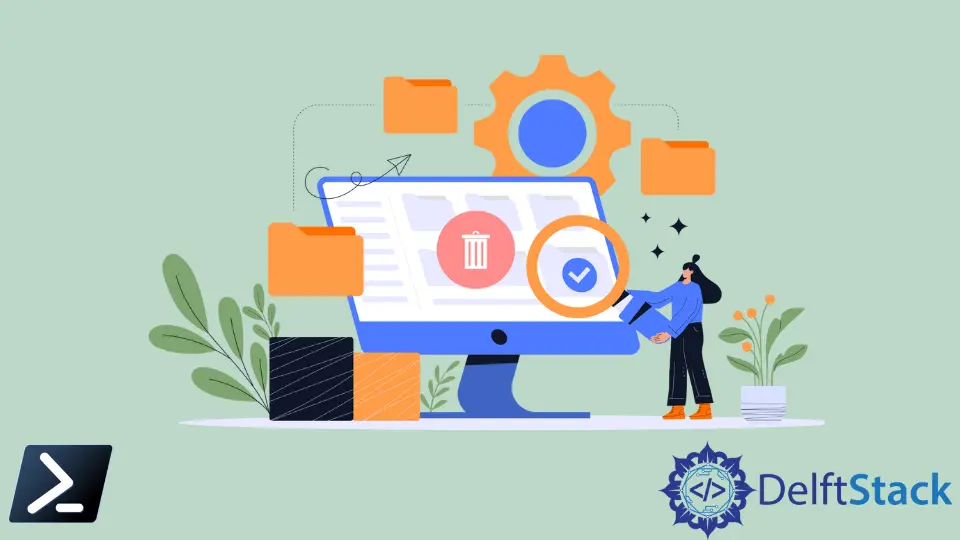
As an administrator, one of our possible duties would probably be managing files. This situation usually happens if we are managing multiple file servers.
One specific task we can do inside the server is copying files into a different location to make perhaps a copy or mirror of a company’s file.
However, what if we need to perform bulk copies at a time? There is a possibility that we can also encounter existing files on the destination location while copying in bulk.
This article will explain how to copy files in bulk, check if they exist in the destination folder, and remove them using PowerShell.
Remove-Item
if File Exists After Copy-Item
in PowerShell
For this article, we will focus on and use the commands Get-ChildItem
, Remove-Item
, and Copy-Item
. We will be sharing the script block where we can copy files in bulk and check if they exist on the destination folder, and if it exists, the file will be removed and overwritten.
So, declare your source and destination folders like the below, and let’s drill down and discuss the script block in detail.
Example Code:
$sourceFolder = "C:\Temp"
$destFolder = "C:\PS"
Get-ChildItem $sourceFolder\Scripts -Recurse | % {
Remove-Item $_ -ErrorAction Ignore
Copy-Item -Path $_.FullName -Destination $destFolder
}
-
First, declare your source and destination folders inside each variable like our
$sourceFolder
and$destFolder
variables. -
Next, we will use the
Get-ChildItem
command to get all the files in the folder and place them in an array list. TheGet-ChildItem
command can also cater to multiple folders.To check for multiple folders, insert a comma (
,
) between destinations like the snippet below.Get-ChildItem $sourceFolder\Scripts, $sourceFolder\Test
The `-Recurse` parameter is used to check for files that are also inside the subfolder of the parent source folder.
-
We then use the
Remove-Item
command to remove the already existing file. As you can see, we have used a parameter called-ErrorAction
that will set our action preference if an error has occurred.In this case, the file does not exist in the destination file if an error occurs. Our action preference is set to
Ignore
, which will ignore theRemove-Item
command if an error has occurred and will continue script execution on that line.Alternatively, we can shorten this command line using aliases like the example snippet below.
rm yourfile.zip -ea ig
Copy Files With the Move-Item
Command
We can also use the Move-Item
command as an alternative to Copy-Item
. The Move-Item
command automatically checks if the file exists in the destination folder.
The command will skip the file if the value is true
. This means we can delete the Remove-Item
command line and have a shorter script that will look something like the snippet of code below.
$sourceFolder = "C:\Temp"
$destFolder = "C:\PS"
Get-ChildItem $sourceFolder\Scripts -Recurse | % {
Move-Item -Path $_.FullName -Destination $destFolder
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn