How to Create Text File Using Windows PowerShell
-
Use
New-Item
to Create a New Text File Through Windows PowerShell -
Use the
ni
Command to Create a New Text File Through Windows PowerShell -
Use
fsutil
to Create a New Text File Through Windows PowerShell -
Use the
echo
Command and>
Operator to Create a New Text File Through Windows PowerShell - Use Text Editor to Create a New Text File Through Windows PowerShell
-
Use the
Set-Content
to Create a New Text File Through Windows PowerShell -
Use the
StreamWriter
to Create a New Text File Through Windows PowerShell -
Use the
Out-File
to Create a New Text File Through Windows PowerShell -
Use the
Add-Content
to Create a New Text File Through Windows PowerShell - Conclusion
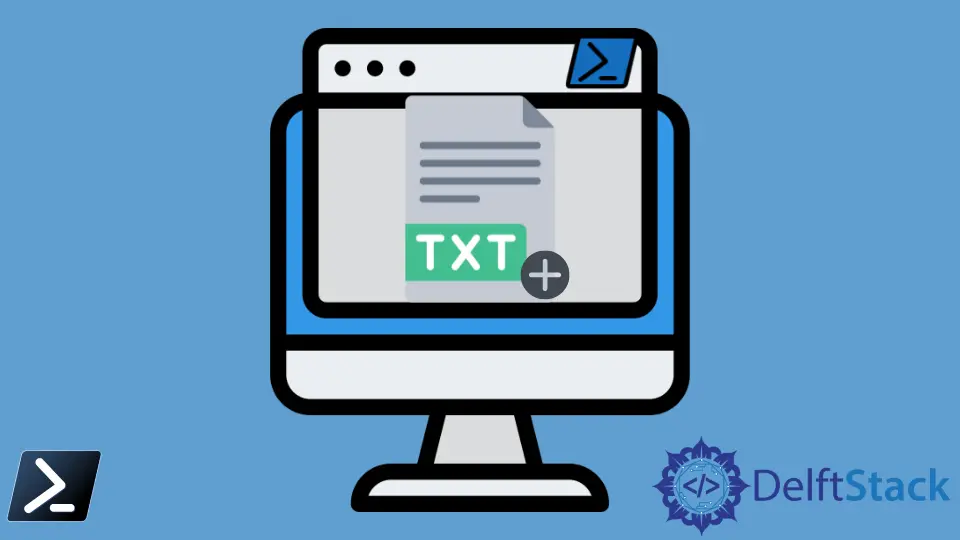
Creating text files in PowerShell is a fundamental aspect of scripting and automation. Whether you need to generate configuration files, log data, or simply store information, PowerShell offers several methods to accomplish this task efficiently.
Among these methods are native cmdlets like New-Item
, Set-Content
, and Out-File
, as well as command-line utilities like fsutil
and text editor commands like notepad
. Additionally, leveraging .NET
classes such as StreamWriter
provides fine-grained control over file creation and content writing.
In this article, we’ll explore each of these methods, providing syntax, examples, and insights into their usage, allowing you to choose the most suitable approach for your specific requirements.
Use New-Item
to Create a New Text File Through Windows PowerShell
In PowerShell, the New-Item
cmdlet is a versatile tool for creating various types of items in the file system. When it comes to text files, New-Item
allows us to effortlessly generate new files with specified content or simply create an empty file.
This method is particularly useful when you need to automate the creation of text files as part of a script or routine task.
New-Item -Path "test.txt" -ItemType "file" -Value "Hello, PowerShell!" -Force
In this example, we utilize the New-Item
cmdlet to create a new text file named test.txt
within the working directory. The -Path
parameter specifies the location and filename, while -ItemType "file"
declares the item type as a file.
The -Value
parameter is employed to set the content of the file, which in this case is "Hello, PowerShell!"
. Lastly, the -Force
flag ensures that the command can overwrite the file if it already exists.
The -Force
parameter is used to overwrite the file if it already exists.
Output:
Use the ni
Command to Create a New Text File Through Windows PowerShell
In PowerShell, the ni
alias stands for New-Item
, which is a versatile cmdlet used to create various types of items in the file system. When it comes to text files, the ni
method allows us to effortlessly generate new files with specified content or simply create an empty file.
ni "test.txt" -ItemType "file" -Force
In this example, we employ the ni
alias to execute the New-Item
cmdlet, facilitating the creation of a new text file named test.txt
within the working directory. The -ItemType "file"
parameter explicitly designates the item type as a file, ensuring the creation of a text file specifically.
Furthermore, the -Force
parameter is utilized to enable the command to overwrite the file if it already exists, ensuring seamless execution even in scenarios where the file may need to be replaced.
Output:
Use fsutil
to Create a New Text File Through Windows PowerShell
In PowerShell, the fsutil
command-line utility is a powerful tool for managing various aspects of the file system. Among its functionalities is the ability to create empty files, which is particularly useful when working with text files.
While fsutil
is primarily a command-line tool and not a native PowerShell cmdlet, it can still be invoked from within PowerShell scripts to accomplish tasks such as creating text files.
fsutil file createnew "test.txt" 0
In this example, we utilize the fsutil
command-line utility to create a new text file named test.txt
within the working directory. By employing the file createnew
subcommand, we instruct fsutil
to generate an empty file of size 0
, denoting a text file devoid of initial content.
This approach provides a straightforward means of creating text files directly from PowerShell, leveraging the functionality of fsutil
to handle file system operations.
Output:
Use the echo
Command and >
Operator to Create a New Text File Through Windows PowerShell
The echo
command is a built-in alias for PowerShell’s Write-Output
cmdlet. It is similar to other shells that use echo
.
Both commands write the output to the pipeline and redirect the output to a new file. It will create a new file with the same output as its content.
echo "Hello, PowerShell!" > "test.txt"
Get-Content test.txt
In this example, we utilize the echo
command to generate a new text file named test.txt
with the content "Hello, PowerShell!"
. By redirecting (>
) the output of echo
to the specified file path, we efficiently create the text file in a single command.
Subsequently, we use the Get-Content
cmdlet to retrieve the content of the test.txt
file, confirming that the specified text has been successfully written to the file.
Output:
Use Text Editor to Create a New Text File Through Windows PowerShell
You can use different text editors in PowerShell to create a new file, such as nano
, notepad
, vim
, emacs
, etc.
The notepad
command is commonly associated with opening the Notepad
application. However, it can also be used to create text files directly from the command line.
notepad test.txt
In this example, we utilize the notepad
command to open the Notepad
application and create a new text file named test.txt
in the working directory. Once Notepad
opens, we can manually enter the desired text content into the file.
After saving the file from within Notepad
, the new text file will be created at the specified location.
Output:
Similarly, you can install other text editors and create a new file with some content.
Use the Set-Content
to Create a New Text File Through Windows PowerShell
In PowerShell, the Set-Content
cmdlet is a powerful tool for writing data to a file. It can be used to create new text files or overwrite existing ones with specified content.
This method offers flexibility and control over the content written to the file, making it a valuable tool for text file manipulation in PowerShell scripts.
Set-Content -Path "test.txt" -Value "Hello, PowerShell!"
Get-Content test.txt
In this code snippet, we utilize the Set-Content
cmdlet to create a new text file named test.txt
with the content "Hello, PowerShell!"
. By specifying the file path and the desired content using the -Path
and -Value
parameters, respectively, we efficiently generate the text file within a single command.
Subsequently, we use the Get-Content
cmdlet to retrieve the content of the test.txt
file, confirming that the specified text has been successfully written to the file.
Output:
Use the StreamWriter
to Create a New Text File Through Windows PowerShell
In PowerShell, the StreamWriter
class from the .NET
framework provides a powerful way to create and write content to text files programmatically. This method offers fine-grained control over the file-writing process, allowing users to specify various parameters such as encoding, append mode, and whether to create the file if it doesn’t exist.
Using StreamWriter
, users can efficiently generate text files with custom content, making it a valuable tool for file manipulation tasks in PowerShell scripts.
$stream = [System.IO.StreamWriter]::new("test.txt")
$stream.WriteLine("Hello, PowerShell!")
$stream.Close()
Get-Content test.txt
In this example, we utilize the StreamWriter
class to create a new text file named test.txt
in the current directory. We initialize a new instance of StreamWriter
with the desired file path and then use the WriteLine
method to write the text content "Hello, PowerShell!"
to the file.
Finally, we close the StreamWriter
object to ensure that all resources are properly released.
Output:
Use the Out-File
to Create a New Text File Through Windows PowerShell
The Out-File
cmdlet provides a straightforward way to create and write content to text files. This method is particularly useful when you need to redirect output from PowerShell commands or scripts directly to a text file.
Out-File
allows users to specify various parameters such as the file path, encoding, and whether to append to an existing file. With its flexibility and ease of use, Out-File
is a versatile tool for generating and managing text files within PowerShell.
"Hello, PowerShell!" | Out-File -FilePath "test.txt"
Get-Content test.txt
In this example, we use the Out-File
cmdlet to create a new text file named test.txt
in the working directory. We provide the text content "Hello, PowerShell!"
as output from the preceding command, which is then redirected (|
) to Out-File
.
The -FilePath
parameter specifies the path where the new file will be created. Subsequently, we use the Get-Content
cmdlet to retrieve the content of the test.txt
file, confirming that the specified text has been successfully written to the file.
Output:
Use the Add-Content
to Create a New Text File Through Windows PowerShell
In PowerShell, the Add-Content
cmdlet is commonly used to append content to existing files. However, it can also be utilized to create new text files if the specified file does not already exist.
This method offers a convenient way to generate text files and add content to them in a single command. Add-Content
allows users to specify various parameters such as the file path, content to be added, and encoding.
Add-Content -Path "test.txt" -Value "Hello, PowerShell!"
Get-Content test.txt
In this example, we use the Add-Content
cmdlet to create a new text file named test.txt
in the working directory. We specify the text content "Hello, PowerShell!"
using the -Value
parameter.
Since the file does not exist yet, Add-Content
will create it and add the specified content. Subsequently, we use the Get-Content
cmdlet to retrieve the content of the test.txt
file, confirming that the specified text has been successfully written to the file.
Output:
Conclusion
PowerShell offers a myriad of methods to create text files, each catering to different scenarios and preferences. Whether you prefer the simplicity of native cmdlets like New-Item
and Out-File
, the flexibility of .NET
classes like StreamWriter
, or the versatility of command-line utilities like fsutil
, PowerShell provides the tools to meet your needs.
By understanding the strengths and capabilities of each method, you can streamline your file creation processes and enhance your scripting workflows. Whether you’re a novice PowerShell user or an experienced scripter, mastering these techniques empowers you to efficiently manage text files and automate tasks with confidence.