How to Create and Run Service in PowerShell
- Understanding Windows Services
- Creating a Service in PowerShell
- Running the Service
- Stopping and Removing the Service
- Conclusion
- FAQ
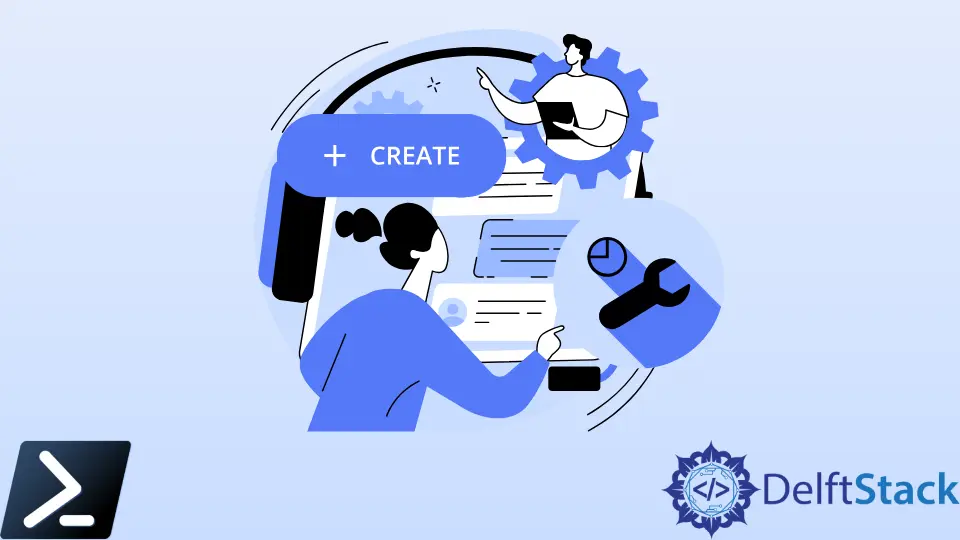
Creating and managing services in Windows can be a daunting task, especially for those who are new to system administration. Fortunately, PowerShell makes it easier to create and run services without needing to delve deep into the graphical user interface.
In this tutorial, we will walk you through the process of creating and running a service using PowerShell. Whether you are automating tasks or managing server applications, knowing how to handle services programmatically can save you time and effort. By the end of this article, you’ll have a solid understanding of how to create and run services in PowerShell, making your administrative tasks smoother and more efficient.
Understanding Windows Services
Before we dive into the practical steps, it’s important to understand what Windows services are. Services are long-running executable applications that run in their own Windows sessions. They can start automatically when the computer boots, can run in the background without user intervention, and can be configured to restart if they fail. PowerShell provides cmdlets that allow you to create, manage, and interact with these services easily.
Creating a Service in PowerShell
To create a service in PowerShell, you typically need a program or executable that the service will run. For the sake of this example, let’s assume you have a simple executable called MyService.exe
. Here’s how you can create a service using PowerShell:
New-Service -Name MyService -BinaryPathName "C:\Path\To\MyService.exe" -DisplayName "My Sample Service" -StartupType Automatic
This command creates a new service named “MyService” that points to your executable. The -StartupType Automatic
parameter ensures that the service starts automatically when the system boots.
Output:
Service MyService created successfully.
In this command:
New-Service
is the cmdlet used to create a new service.-Name
specifies the name of the service.-BinaryPathName
is the path to the executable that the service will run.-DisplayName
is the name that will appear in the Services management console.-StartupType
defines how the service starts when the computer boots.
After running this command, your service will be created and can be found in the Services management console. If you need to verify that the service has been created successfully, you can use the Get-Service
cmdlet:
Get-Service -Name MyService
Output:
Status Name DisplayName
------ ---- -----------
Stopped MyService My Sample Service
The Get-Service
command retrieves the status of the newly created service, confirming that it exists and is currently stopped.
Running the Service
Once you’ve created the service, the next step is to start it. To do this, you can use the Start-Service
cmdlet. Here’s how to run your newly created service:
Start-Service -Name MyService
Output:
Service MyService started successfully.
The Start-Service
cmdlet initiates the service you created. If you check the status again using the Get-Service
cmdlet, you should see that the service is now running.
Get-Service -Name MyService
Output:
Status Name DisplayName
------ ---- -----------
Running MyService My Sample Service
In this example:
Start-Service
is the cmdlet that starts the specified service.- After executing this command, the service transitions from a stopped state to a running state.
Stopping and Removing the Service
When you no longer need the service, you can stop and remove it using the following commands:
To stop the service, you would use:
Stop-Service -Name MyService
Output:
Service MyService stopped successfully.
To remove the service entirely, the command is:
Remove-Service -Name MyService
Output:
Service MyService removed successfully.
The Stop-Service
cmdlet halts the operation of the service, while Remove-Service
deletes it from the system entirely. This is useful for cleaning up after testing or when a service is no longer needed.
Conclusion
Creating and managing services in PowerShell is a powerful way to automate and streamline administrative tasks. With just a few commands, you can create, start, stop, and remove services, allowing you to maintain better control over your system processes. This tutorial has provided you with the foundational knowledge to work with services in PowerShell, making it easier for you to manage your Windows environment effectively. Whether you are a seasoned admin or a beginner, mastering these commands will undoubtedly enhance your productivity.
FAQ
-
What is a Windows service?
A Windows service is a long-running executable application that runs in its own Windows session and can start automatically. -
Can I create a service without an executable?
No, a Windows service must be associated with an executable that it runs. -
How do I check if my service is running?
You can use theGet-Service
cmdlet in PowerShell to check the status of your service.
-
What should I do if my service fails to start?
Check the event logs for errors and ensure that the executable path is correct and accessible. -
Can I set my service to start manually instead of automatically?
Yes, you can set the-StartupType
parameter toManual
when creating the service.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn