How to Check if a Service Is Running Using PowerShell
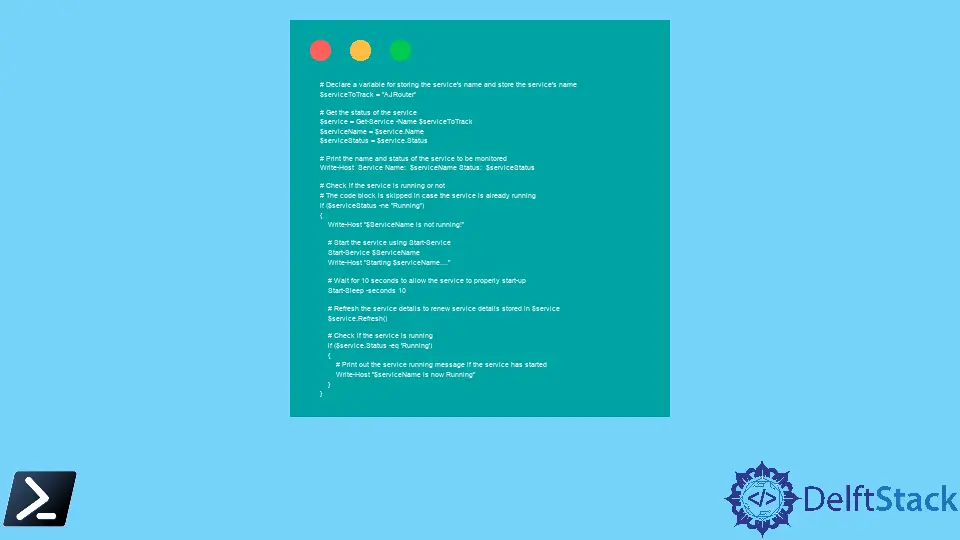
This article aims to demonstrate how to see whether a Windows Service is currently running or not using PowerShell scripting and if the service is not running, turn the service on.
Check if a Service Is Running Using PowerShell
As one of the core components of Microsoft Windows, Services are vital in ensuring that long-running processes are created and managed smoothly. Based on its importance in core execution, one may want to continuously monitor critical systems or custom-made services, track their behavior, check if they are running, and take appropriate measures if any abnormal measure is encountered.
Consider the following cmdlet used to print all available services present:
sc queryex type=service state=all
This shows the following output:
.
.
< Output Redacted >
.
SERVICE_NAME: AJRouter
DISPLAY_NAME: AllJoyn Router Service
TYPE : 20 WIN32_SHARE_PROCESS
STATE : 1 STOPPED
WIN32_EXIT_CODE : 1077 (0x435)
SERVICE_EXIT_CODE : 0 (0x0)
CHECKPOINT : 0x0
WAIT_HINT : 0x0
PID : 0
FLAGS :
SERVICE_NAME: ALG
DISPLAY_NAME: Application Layer Gateway Service
TYPE : 10 WIN32_OWN_PROCESS
STATE : 1 STOPPED
WIN32_EXIT_CODE : 1077 (0x435)
SERVICE_EXIT_CODE : 0 (0x0)
CHECKPOINT : 0x0
WAIT_HINT : 0x0
PID : 0
FLAGS :
SERVICE_NAME: ApHidMonitorService
DISPLAY_NAME: AlpsAlpine HID Monitor Service
TYPE : 10 WIN32_OWN_PROCESS
STATE : 4 RUNNING
(STOPPABLE, NOT_PAUSABLE, ACCEPTS_SHUTDOWN)
WIN32_EXIT_CODE : 0 (0x0)
SERVICE_EXIT_CODE : 0 (0x0)
CHECKPOINT : 0x0
WAIT_HINT : 0x0
PID : 4464
FLAGS :
.
.
.
< Output Redacted >
From the output above, it can be seen that many services are running and stopped. Among this large list of services, our important service will also be present, which we want to keep track of for any abnormal behavior or to see if it is running.
Let’s see how we can monitor our activity using PowerShell scripting.
Consider the following snippet:
# Declare a variable for storing the service's name and store the service's name
$serviceToTrack = "AJRouter"
# Get the status of the service
$service = Get-Service -Name $serviceToTrack
$serviceName = $service.Name
$serviceStatus = $service.Status
# Print the name and status of the service to be monitored
Write-Host Service Name: $serviceName Status: $serviceStatus
# Check if the service is running or not
# The code block is skipped in case the service is already running
if ($serviceStatus -ne "Running") {
Write-Host "$ServiceName is not running!"
# Start the service using Start-Service
Start-Service $ServiceName
Write-Host "Starting $serviceName...."
# Wait for 10 seconds to allow the service to properly start-up
Start-Sleep -Seconds 10
# Refresh the service details to renew service details stored in $service
$service.Refresh()
# Check if the service is running
if ($service.Status -eq 'Running') {
# Print out the service running message if the service has started
Write-Host "$serviceName is now Running"
}
}
As seen in the code above, any desired service can easily be tracked via PowerShell scripting.
First, the name of the service is stored in the variable named $serviceToTrack
. After which, the name is passed to the Get-Service
cmdlet as a -Name
parameter.
The Get-Service
fetches detailed information about the activity passed to it, including but not limited to its name and status. Both of which are required in our case.
After acquiring the service, we can check its name and status using the Name
and Status
members of the recently fetched service, done mostly to confirm that the service we fetched is indeed the one we want to monitor.
Before starting the service, it is necessary to check whether the service is running. Again the status member is used here.
If it is set to Running
, the service is already active or running and does not need to be started. On the other hand, if the service is not running, we can use the Start-Service
cmdlet, passing the name of the service to start as one of its parameters.
To ensure that the service is properly started and running, we must wait for some time, using the Start-Sleep
cmdlet, and the time to sleep passed.
After we have waited for enough time, we can check the status of the service. To update the service to its current state, we can use the Refresh()
method to get the latest state of the service.
A simple comparison check after that can confirm whether the service is running or not.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn