How to Manage Services in PowerShell
- List Services in PowerShell
- Find Remote Services in PowerShell
- Start and Stop Services in PowerShell
- Restart Service in PowerShell
- Change the Startup Type of a Service in PowerShell
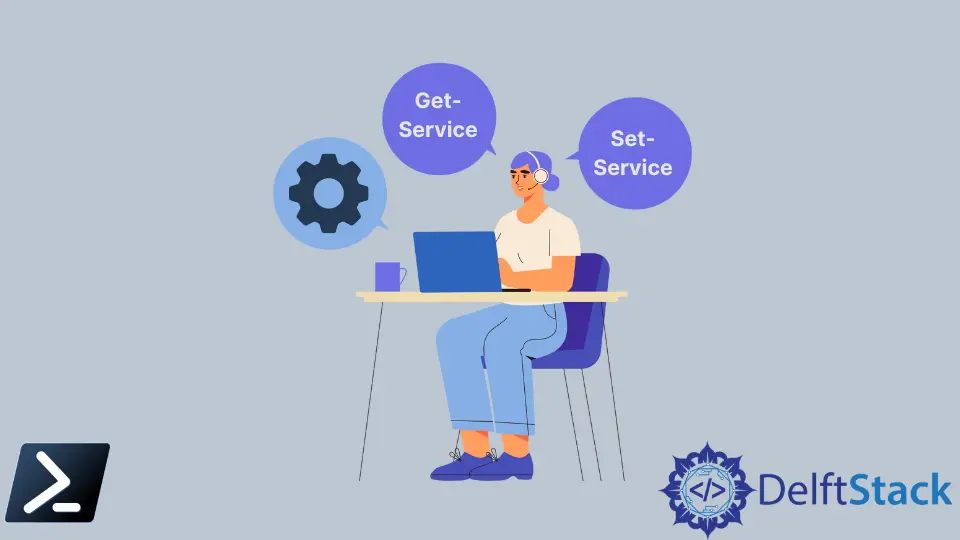
Windows services are one of the topics nearly every Windows sysadmin has to use. Of course, to manage Windows services, we could start the services.msc
MMC snap-in for one-off tasks, but what if we need to build some automation with PowerShell?
This article will discuss all the PowerShell Service cmdlets, use them, and develop our script to manage services on many computers, locally or remotely.
List Services in PowerShell
One of the most basic tasks we can accomplish with Windows PowerShell and services is simply enumerating what services exist on a local or a remote computer. For example, open up PowerShell, run the Get-Service
command, and observe the output.
The Get-Service
command by itself will list all services on the local computer and the Status
, the Name
, and DisplayName
of each service.
Get-Service
Output:
Status Name DisplayName
------ ---- -----------
Stopped AarSvc_55244 AarSvc_55244
Stopped AJRouter AllJoyn Router Service
Stopped ALG Application Layer Gateway Service
Running AMD Crash Defen... AMD Crash Defender Service
<SNIP>
Like many other cmdlets, PowerShell does not return all of the properties for each service.
Although, for example, we would like to see a required service or perhaps the service description, we can find these properties by piping the result to the Select-Object
command and using the *
wildcard to represent all properties.
Get-Service ALG | select *
Output:
Name : ALG
RequiredServices : {}
CanPauseAndContinue : False
CanShutdown : False
CanStop : False
DisplayName : Application Layer Gateway Service
DependentServices : {}
MachineName : .
ServiceName : ALG
ServicesDependedOn : {}
ServiceHandle :
Status : Stopped
ServiceType : Win32OwnProcess
StartType : Manual
Site :
Container :
Find Remote Services in PowerShell
Maybe we are on a network and need to enumerate services across one or more remote Windows computers. We could have done this in the Windows PowerShell days by using the -ComputerName
parameter, but unfortunately, that parameter doesn’t exist anymore.
One way to inspect Windows services on a remote computer is by using Windows PowerShell Remoting (or PS Remoting). Using PS Remoting can encapsulate any local command and invoke it in a remote session just as we were doing it locally.
Assuming we have PowerShell Remoting enabled on a remote computer, we could, for example, use the Invoke-Command
cmdlet to run the Get-Service
command on a remote computer.
$cred = Get-Credential
Invoke-Command -ComputerName SRV1 -ScriptBlock { Get-Service } -Credential $cred
Output:
Status Name DisplayName PSComputerName
------ ---- ----------- --------------
Stopped AarSvc_55244 AarSvc_55244 SRV1
<SNIP>
Once executed, the Invoke-Command
cmdlet passes on all of the information that Get-Service
returned, and services would be returned to you as expected.
Notice the extra PSComputerName
property returned by the Invoke-Command
cmdlet. We can also create a simple script to enumerate services across many remote computers.
Start and Stop Services in PowerShell
We can also start and stop services with Windows PowerShell.
The Start-Service
and Stop-Service
cmdlets precisely do what we would expect. Next, we can use the pipeline or the -Name
parameter below.
## Stop a service
$serviceName = 'wuauserv'
Stop-Service -Name $serviceName
## Stop a service with the pipeline
Get-Service $wuauserv | Stop-Service
All of the *-Service
cmdlets allow us to tab-complete service name values with the -Name
and -DisplayName
parameters. Just type the -Name
parameter followed by a space and hit the Tab
key.
We will see that it cycles through all of the services on the local computer. The same concept applies to starting as a service too.
## Start a service
$serviceName = 'wuauserv'
Start-Service -Name $serviceName
## Start service with the pipeline
Get-Service $wuauserv | Start-Service
Both the Start-Service
and Stop-Service
commands are idempotent, meaning if a service is either started or stopped and we attempt to stop or start the service when they are already in that state, the cmdlets will skip over the service.
To stop and start remote services with PowerShell, again, you’ll need to wrap these commands in a script block and use PowerShell Remoting to invoke them remotely, as shown below.
$cred = Get-Credential
$serviceName = 'wuauserv'
Invoke-Command -ComputerName SRV02 -ScriptBlock { Start-Service -Name $using:serviceName } -Credential $cred
Restart Service in PowerShell
To limit code reuse to restart a service with Windows PowerShell, we would better use the Restart-Service
cmdlet. This cmdlet precisely does what we think and operates similarly to the other service commands.
For example, if we would like to start and stop the wuauserv
service as shown in the previous example above, we could save some code by just piping the output of Get-Service
directly to Restart-Service
, as shown below.
## Restart a service with the Name parameter
$serviceName = 'wuauserv'
Get-Service -Name $serviceName | Restart-Service
Change the Startup Type of a Service in PowerShell
The startup type of a service is the attribute that dictates what the services do when Windows boots up. So again, we have a few options.
Automatic
: The service automatically starts when Windows boots up.Disabled
: The service will never start unless changed.Manual
: The service can be started but must be done manually.Automatic – Delayed
: The service starts automatically but is delayed once Windows boots.
First, we need to know what a startup type is. You can find this with Get-Service
.
If you’re using Get-Service
to find the startup type, you’ll find that Get-Service
calls it Status
and is represented as the Status
property.
(Get-Service -Name wuauserv | select *).StartType
Output:
Manual
Once you know the current startup type, we can change it using Set-Service
. The below example is setting the startup type to Automatic
.
Set-Service -Name <service name> -StartupType Automatic
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn