How to Check if Folder Exists in PowerShell
-
Use the
Test-Path
Cmdlet to Check if a Folder Exists in PowerShell -
Use the
System.IO.Directory
to Check if a Folder Exists in PowerShell -
Use the
Get-Item
Cmdlet to Check if a Folder Exists in PowerShell - Conclusion
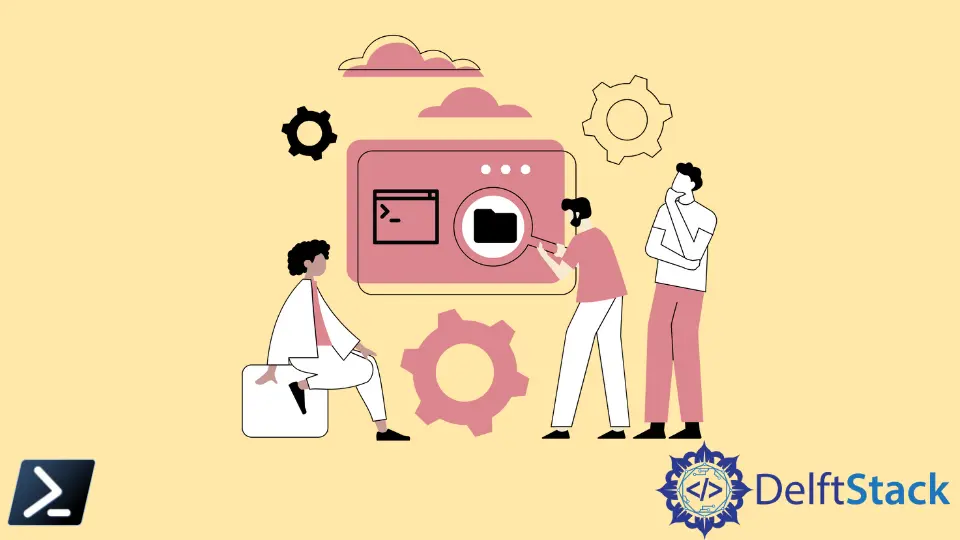
PowerShell is a powerful tool that can perform different file and folder operations. It allows you to create, copy, move, rename, delete, and view files and folders on the system.
File and folder management is a feature of PowerShell, allowing users to not only manipulate these entities but also verify their existence in the system. In this tutorial, we’ll explore various methods to check for the existence of a folder in your system using PowerShell.
Use the Test-Path
Cmdlet to Check if a Folder Exists in PowerShell
The Test-Path
cmdlet determines whether all path elements exist or not in PowerShell. It returns a Boolean value, True
if all elements exist, and False
if any are missing.
Syntax:
Test-Path -Path "C:\Path\to\Folder"
Parameter:
-Path
: This parameter indicates the path to the folder or file you want to check for existence. In this case, it’s set to"C:\Path\to\Folder"
, indicating that you want to check if the folder located on that path exists.
For example, the following command checks whether all elements of the path C:\New\complex
exist or not.
Test-Path -Path "C:\New\complex"
Output:
True
It means the complex
folder exists in the C:\New
directory.
This following command checks if the Documents
folder exists in the C:\New
directory.
Test-Path -Path "C:\New\Documents"
Output:
False
Hence, the Documents
folder is not present in the C:\New
directory.
If you want to return verbose information instead of True
/False
, you can use the if
statement like this.
if (Test-Path -Path "C:\New\Documents") {
Write-Host "The given folder exists."
}
else {
Write-Host "The given folder does not exist."
}
In this code, we use the Test-Path
cmdlet to check if a folder, "C:\New\Documents"
, exists in the system. If the folder exists, it prints "The given folder exists"
using Write-Host
.
If the folder does not exist, it prints "The given folder does not exist"
in the console.
Output:
The given folder does not exist.
As it turns out, the "C:\New\Documents"
folder does not exist in the system, leading the Test-Path
cmdlet to return False
. Our code triggers the else block, displaying the "The given folder does not exist."
message on the console.
This output accurately signifies that the specified folder was not located at the provided path within the system.
Use the System.IO.Directory
to Check if a Folder Exists in PowerShell
The System.IO.Directory
class from the .NET
Framework provides static methods for creating, moving, deleting, and enumerating through directories and subdirectories. You can use its Exists()
method to see if the provided path refers to a directory that already exists on the system.
It also returns True
if the path exists and False
if it does not exist.
[System.IO.Directory]::Exists("C:\New\complex")
Output:
True
The [System.IO.Directory]::Exists("C:\New\complex")
has no parameter being used. Instead, it is directly calling a method to check if a folder exists in PowerShell.
Now, let’s check if the Documents
folder exists in the C:\New
directory.
[System.IO.Directory]::Exists("C:\New\Documents")
In the command above, we’re using the .NET Framework called "System.IO.Directory"
to check if a folder exists. This tool has a feature called "Exists()"
that helps us with this task.
We tell it to look at the "C:\New\Documents"
location and tell us if there’s a folder there. If it finds the folder, it will return True
, but if the folder does not exist, it says False
.
Output:
False
The output is "False"
. This means that the System.IO.Directory::Exists()
indicates that the folder "C:\New\Documents"
does not exist at the specified path.
Use the Get-Item
Cmdlet to Check if a Folder Exists in PowerShell
The Get-Item
gets the item at the given path. If the path exists on the system, it prints the Mode
, LastWriteTime
, Length
, and Name
of the directory.
Get-Item C:\Users\Public
In this command, we’re retrieving information about the Public
folder situated at C:\Users\Public
. When we execute this command in PowerShell, the console will display various details regarding the folder.
Output:
Directory: C:\Users
Mode LastWriteTime Length Name
---- ------------- ------ ----
d-r--- 10/15/2023 6:35 PM Public
If the specified path does not exist, you will get an error message saying it does not exist, like in the following example.
Get-Item C:\Users\Publics
Output:
Get-Item : Cannot find path 'C:\Users\Publics' because it does not exist.
At line:1 char:1
+ Get-Item C:\Users\Publics
+ ~~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : ObjectNotFound: (C:\Users\Publics:String) [Get-Item], ItemNotFoundException
+ FullyQualifiedErrorId : PathNotFound,Microsoft.PowerShell.Commands.GetItemCommand
Conclusion
These approaches provide distinct ways to check the presence of folders in PowerShell, and you can modify them to meet your specific requirements. These techniques are not only applicable to folders but can also be used to check for the existence of files within your system.
We hope this article gave you an idea of checking if a folder exists in PowerShell.