How to Set Folder Permissions in PowerShell
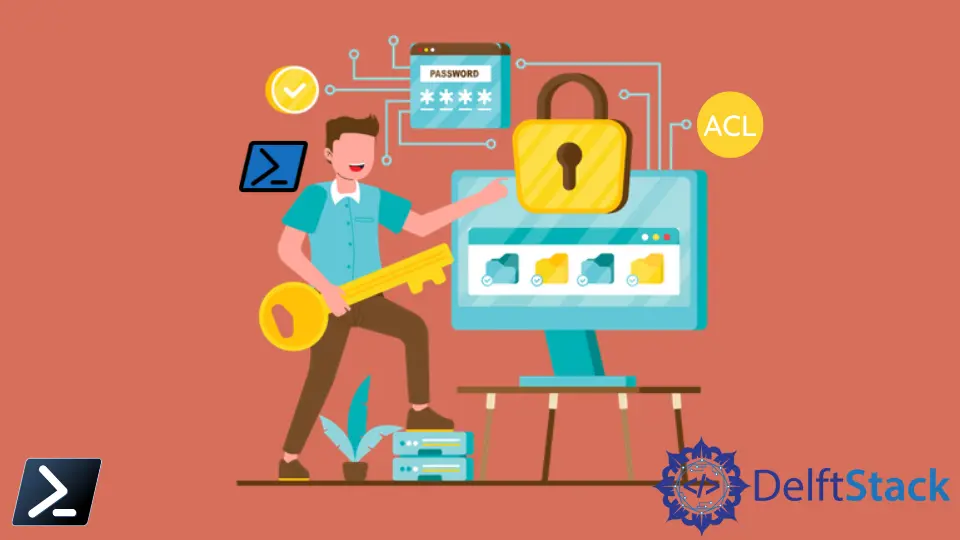
An ACL (access control list) represents users’ permissions and user groups for accessing a file or resource. It is an ordered list of access control entries (ACEs).
When adding the access control entries, you can define the access rights allowed or denied and set user and group permissions on the folder. Using the GUI of File Explorer, you can easily set or change the folder’s permissions in Windows.
In this tutorial, you will learn to set folder permissions using PowerShell.
Use the Set-Acl
Cmdlet to Set Folder Permissions in PowerShell
The ACLs store the information in a security depositor. The security descriptor has two ACL types: system ACL (SACL) and discretionary ACL (DACL).
Each ACE in DACL contains three types of information:
- A security identifier (SID): An SID determines to whom the ACE applies. It can be a single user or a group of users.
- A set of access rights: An access right is the right to perform a particular operation on the object. For more details, see Access Rights.
- Whether a set of access rights is allowed or denied.
The Set-Acl
changes the security descriptor of a specified file or resource. It applies the security descriptor supplied as the value of the -AclObject
parameter.
The following example adds the new ACL rule to the existing permission on the folder C:\pc\computing
.
$acl = Get-Acl -Path "C:\pc\computing"
$ace = New-Object System.Security.Accesscontrol.FileSystemAccessRule ("testuser", "Read", "Allow")
$acl.AddAccessRule($ace)
Set-Acl -Path "C:\pc\computer" -AclObject $acl
The first command gets the existing ACL rules of the C:\pc\computing
. The second command creates a new FileSystemAccessRule
to apply to the folder.
The third command adds the new ACL rule to the existing permissions on the folder. The fourth command uses Set-Acl
to apply the new ACL to the folder.
You can view the ACLs of the folder using the following command.
Get-Acl C:\pc\computing | Format-List
Output:
Path : Microsoft.PowerShell.Core\FileSystem::C:\pc\computing
Owner : DelftStack\rhntm
Group : DelftStack\rhntm
Access : DelftStack\testuser Allow Read, Synchronize
DelftStack\rhntm Allow FullControl
Audit :
Sddl : O:S-1-5-21-1715350875-4262369108-2050631134-1001G:S-1-5-21-1715350875-4262369108-2050631134-1001D:AI(A;;FR;;;S-1-5-21-1715350875-4262369108-2050631134-1003)(A;OICIID;FA;;;S-1-5-21-1715350875-4262
369108-2050631134-1001)
As you can see, the user testuser
has read permission on the folder now. You can set permissions on a large number of folders and files using scripts easily and quickly.
Also, read the article on how to Recursively Set Permissions on Folders Using PowerShell.