How to Compare Folders in PowerShell
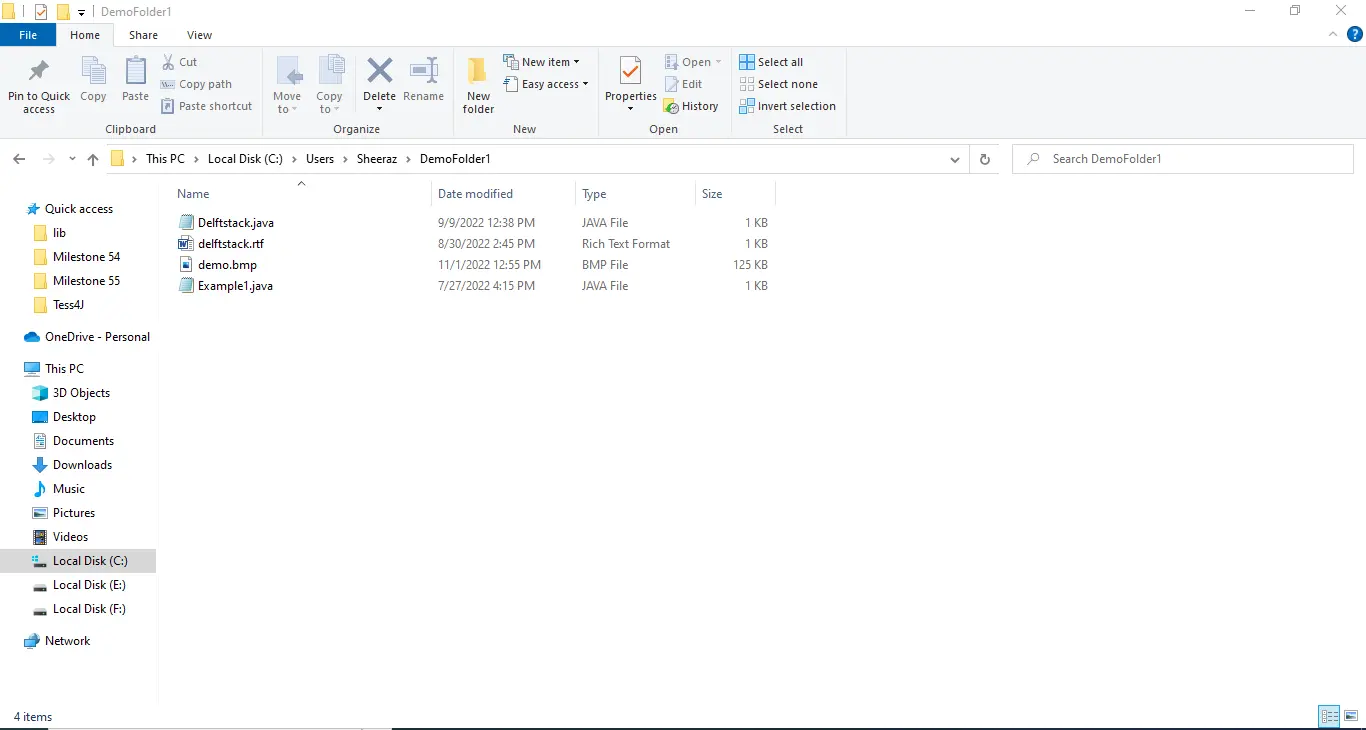
This tutorial demonstrates how to compare two folders using PowerShell.
PowerShell Compare Folders
Comparing two folders in PowerShell is an easy step-by-step process. We can use the Compare-Object
method of PowerShell to compare the contents of the given folders.
See the stepwise process for the given two folders:
Get the Contents of the Folders
The first step is to get the contents of the given folder. First, run the following commands to get the children of the folders:
$SourceFolder = Get-ChildItem -Path C:\Users\Sheeraz\DemoFolder1
$DestinationFolder = Get-ChildItem -Path C:\Users\Sheeraz\DemoFolder2
The above command will get the contents of the folder. And to show the contents, call the declared variable:
$SourceFolder
The output for the source folder content is:
Directory: C:\Users\Sheeraz\DemoFolder1
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 9/9/2022 12:38 PM 399 Delftstack.java
-a---- 8/30/2022 2:45 PM 51 delftstack.rtf
-a---- 11/1/2022 12:55 PM 127286 demo.bmp
-a---- 7/27/2022 4:15 PM 318 Example1.java
$DestinationFolder
The output for the destination folder content is:
Directory: C:\Users\Sheeraz\DemoFolder2
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 9/20/2022 5:16 PM 68 composer.json
-a---- 9/20/2022 5:16 PM 4774 composer.lock
-a---- 9/20/2022 4:11 PM 2801573 composer.phar
-a---- 8/30/2022 2:45 PM 51 delftstack - Copy.rtf
-a---- 9/9/2022 12:38 PM 399 Delftstack.java
-a---- 8/30/2022 2:45 PM 51 delftstack.rtf
-a---- 11/1/2022 12:55 PM 127286 demo - Copy.bmp
-a---- 11/1/2022 12:55 PM 127286 demo.bmp
-a---- 7/27/2022 4:15 PM 318 Example1.java
Calculate the Number of Files and the Length of the Given Folders
This is an optional step where we count the number of files with particular extensions in each folder and calculate the size of each folder. Run the following commands.
Count the number of files with a particular extension in each folder:
($DestinationFolder | where { $_.Extension -eq '.java' }).count
The above command will count the number of files with the extension .java
in the destination folder. The output we will obtain for our folder is 2.
Similarly, in the source folder:
($SourceFolder | where { $_.Extension -eq '.java' }).count
The above command will count the files in the source folder with the extension .java
. The output we will obtain for our folder is 2.
Now to calculate the size of the given folder, run the following commands:
($DestinationFolder | Measure-Object -Property length -Sum).Sum
($SourceFolder | Measure-Object -Property length -Sum).Sum
The above commands will calculate the size of each given folder. The output for our folders is as follows:
3061806
128054
Compare the Given Folders by Name
We will first compare the folders by their content name, which does not compare the content inside the files. See the command:
Compare-Object -ReferenceObject $SourceFolder.Name -DifferenceObject $DestinationFolder.Name
The above command will compare the folder content by their names and show the different files in both folders. See the output:
InputObject SideIndicator
----------- -------------
composer.json =>
composer.lock =>
composer.phar =>
delftstack - Copy.rtf =>
demo - Copy.bmp =>
It shows that these five files are different from the source folder by name.
Compare the Given Folders by Files Content
In the final step, we will compare the folder by the content in their files. To perform this operation, first, we need to convert the files in folders to hash
.
The hash
command is easy to use. For example:
Get-FileHash 'Delftstack.java'
The above command will get the hash
for the file Delftstack.java.
See the output:
Algorithm Hash Path
--------- ---- ----
SHA256 FACF7DD67442E2EF84460669E007DA40D17D82E4448D9DBC7E575D25D6012D30 C:\Users\Sheeraz\Delftstack.java
To get the hash
for each file in the folder, we need to run the foreach
loop. See the commands:
$SourceFolder = Get-ChildItem -Path C:\Users\Sheeraz\DemoFolder1 | foreach { Get-FileHash -Path $_.FullName }
$DestinationFolder = Get-ChildItem -Path C:\Users\Sheeraz\DemoFolder2 | foreach { Get-FileHash -Path $_.FullName }
The above commands will get the hash
for each file in both folders. To show them, we need to run the following commands:
$DestinationFolder.Hash
$SourceFolder.Hash
The output for the destination folder is:
BBBFED20764B6B3FAE56DF485778F5F27918B63008B0EBCAF1342CA8ADC3EADF
23D197F445E3264BF230128A7AFB9BF0E65209249D51C4AD70F51F44DF2D83C5
8FE98A01050C92CC6812B8EAD3BD5B6E0BCDC575CE7A93B242BDE497A31D7732
3D543438B2036F4AFF2CBCD9527FDB00CB249CF6D4D01E3FC2E5DA1C693EC76C
FACF7DD67442E2EF84460669E007DA40D17D82E4448D9DBC7E575D25D6012D30
3D543438B2036F4AFF2CBCD9527FDB00CB249CF6D4D01E3FC2E5DA1C693EC76C
DE35F58F1AF9A75902844BA13D5821EB99957B79555330EFF8C9F974C2481932
DE35F58F1AF9A75902844BA13D5821EB99957B79555330EFF8C9F974C2481932
CCCDDAFD5EDB60F6CDB4E20CFE9FEBE74AB15D28AD0E465B56D23247F33B3315
The output for the source folder is:
FACF7DD67442E2EF84460669E007DA40D17D82E4448D9DBC7E575D25D6012D30
3D543438B2036F4AFF2CBCD9527FDB00CB249CF6D4D01E3FC2E5DA1C693EC76C
DE35F58F1AF9A75902844BA13D5821EB99957B79555330EFF8C9F974C2481932
CCCDDAFD5EDB60F6CDB4E20CFE9FEBE74AB15D28AD0E465B56D23247F33B3315
Now we can compare the content of both folders by their hash
value. See the command:
Compare-Object -ReferenceObject $SourceFolder.Hash -DifferenceObject $DestinationFolder.Hash
The above command will compare the content of two folders by their hash
and output the hash
for different content. See the output:
InputObject SideIndicator
----------- -------------
BBBFED20764B6B3FAE56DF485778F5F27918B63008B0EBCAF1342CA8ADC3EADF =>
23D197F445E3264BF230128A7AFB9BF0E65209249D51C4AD70F51F44DF2D83C5 =>
8FE98A01050C92CC6812B8EAD3BD5B6E0BCDC575CE7A93B242BDE497A31D7732 =>
3D543438B2036F4AFF2CBCD9527FDB00CB249CF6D4D01E3FC2E5DA1C693EC76C =>
DE35F58F1AF9A75902844BA13D5821EB99957B79555330EFF8C9F974C2481932 =>
As we cannot see which file has the different content, to show the name of the files, run the following command:
(Compare-Object -ReferenceObject $SourceFolder -DifferenceObject $DestinationFolder -Property hash -PassThru).Path
The above command will show the full path to the files containing different content in both folders. See the output:
C:\Users\Sheeraz\DemoFolder2\composer.json
C:\Users\Sheeraz\DemoFolder2\composer.lock
C:\Users\Sheeraz\DemoFolder2\composer.phar
C:\Users\Sheeraz\DemoFolder2\delftstack.rtf
C:\Users\Sheeraz\DemoFolder2\demo.bmp
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook