How to Move Files and Folders Using PowerShell
- Check if a File or Folder Exists Using PowerShell
- Creating Files and Folders Using PowerShell
- Moving Files and Folders Using PowerShell
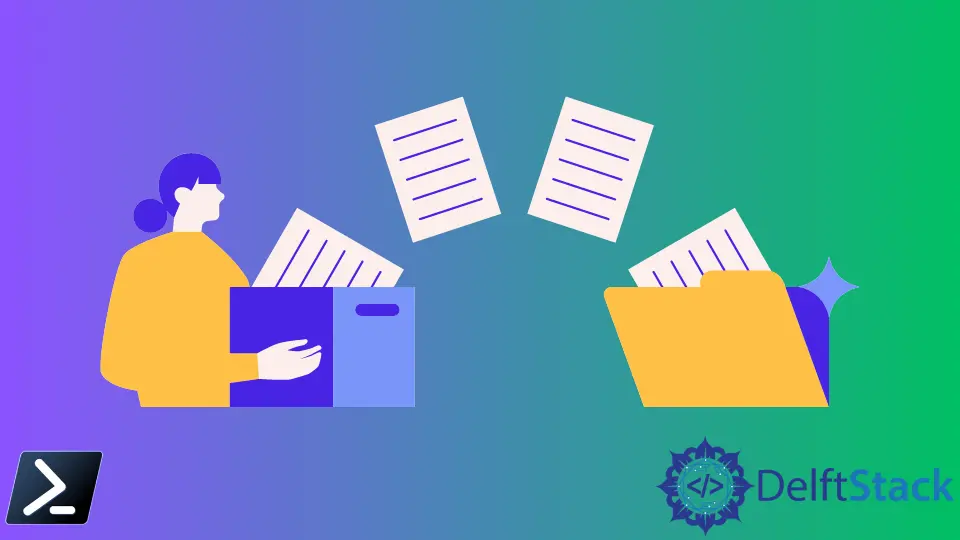
Moving files and folders is a fundamental operation when using the Windows operating system, or perhaps any operating system. We can transfer files or folders one by one or in bulk by simply dragging and dropping them on our screen, but we may need to consider scripting if we only need to move many particular files (like files with specific file formats).
This article will discuss several essential cmdlets when moving files and directories using PowerShell scripting for bulk remediation.
Check if a File or Folder Exists Using PowerShell
The Test-Path
command is a native PowerShell built-in cmdlet that returns True
or False
depending on whether a file or a directory path exists. We can use the Test-Path
PowerShell command if we need to query if a single file exists.
To demonstrate, create a file (test.txt)
in your current working directory or pick any other existing file. Once we have selected our test file, run the command below, replacing the path (C:\Temp\PS) and file name (test.txt)
.
The Test-Path
command will return a Boolean value when executed.
Command:
Test-Path C:\Temp\PS\test.txt
In the above example, the command returns a True
value if the file (test.txt)
exists. Otherwise, we will see a False
value displayed on the console.
Creating Files and Folders Using PowerShell
The Windows PowerShell New-Item
command creates both files and directories. Below are two examples of using the Windows PowerShell New-Item
cmdlet to create a file and a folder.
Command:
# Create a Folder
New-Item -ItemType 'Directory' -Name 'C:\Temp\PS'
# Create a File
New-Item -ItemType 'File' -Name 'Test.txt' -Path '.\PS'
Moving Files and Folders Using PowerShell
The PowerShell move file command, Move-Item
cmdlet, works to move single files or directories and offers filtering capabilities to help quickly transfer content from one location to the next.
In the below example, we move the previously created file, TestFile.txt
, to the parent directory. Using the syntax of ..
to signify the parent directory from the current location, use the Windows PowerShell Move-Item
command to move the file.
Command:
Move-Item -Path '.\TestFile.txt' -Destination '..'
We can not only move files but can also move folders using the Move-Item
command.
First, we need to specify the value of the -Path
parameter to the folder that we need to move. Notice that we have removed the file extension from the -Path
parameter.
Command:
Move-Item -Path '.\PSScripts` -Destination '..'
Perhaps we want to transfer our files and folders to a folder that is not the direct parent of our working directory. Then, we can supply the -Destination
parameter with a custom directory.
Command:
Move-Item -Path '.\PSScripts` -Destination 'C:\Temp'
If the destination folder supplied in the -Destination
parameter does not exist, the script throws an error. The caveat when using the Move-Item
cmdlet is that it doesn’t create a new directory if the destination directory does not exist.
We can use our previously mentioned Test-Path
and New-Item
commands to fix this. In the below script block, we will check the destination path first to see if it exists.
If the folder doesn’t exist, then the script creates it first. Once created, it will now initiate the file or folder transfer.
Code:
$current_folder = "C:\Temp\PS"
$new_folder = "C:\PS\PSScripts"
if (Test-Path -Path $new_folder) {
Move-Item -Path $current_folder -Destination $new_folder
}
else {
New-Item -ItemType 'Directory' -Name $new_folder
Move-Item -Path $current_folder -Destination $new_folder
}
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn