How to Delete Files Older Than X Days Using PowerShell
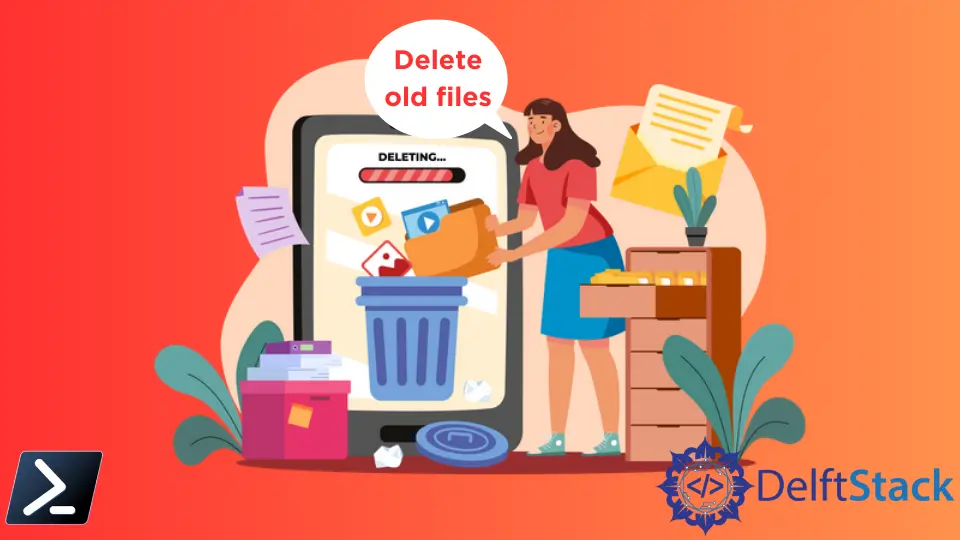
PowerShell supports the handling of different file operations in the system. You can perform various file tasks such as create
, copy
, move
, rename
, edit
, delete
, and view
files in PowerShell.
Many files remain unused for a long time in the system. Some files might be no longer required and need to be deleted after certain days.
You will have to delete the files manually, checking the date created from the File Explorer. It may cost you a lot of time when there are many files to be deleted.
Instead, you can use PowerShell commands to automate the file deletion task, saving you a lot of time.
This tutorial will teach you to delete files older than X days using PowerShell.
Use Remove-Item
Cmdlet to Delete Files Older Than X Days Using PowerShell
The Remove-Item
cmdlet deletes one or more items from the specified location. Multiple providers support it.
You can use this cmdlet to remove different types of items, including files, folders, registry keys, variables, aliases, and functions. It does not return any output.
For example, we have a file C:\New\test.txt
.
Get-Item "C:\New\test.txt"
Output:
Directory: C:\New
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 2/1/2022 11:39 AM 80 test.txt
Now, we will delete it using Remove-Item
.
Remove-Item "C:\New\test.txt"
Get-Item "C:\New\test.txt"
Output:
As you can see, the file path is not found because the file is deleted.
Get-Item : Cannot find path 'C:\New\test.txt' because it does not exist.
At line:1 char:1
+ Get-Item C:\New\test.txt
+ ~~~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : ObjectNotFound: (C:\New\test.txt:String) [Get-Item], ItemNotFoundException
+ FullyQualifiedErrorId : PathNotFound,Microsoft.PowerShell.Commands.GetItemCommand
You cannot directly pipe a path to this cmdlet. But, you can pipe a string that contains a path.
Now, let’s see how you can delete files older than X days using Remove-Item
.
Here, we have a directory C:\test_folder1
, and we will show you how to delete all files older than X days in that directory.
The following command gets all the files in the C:\test_folder1
directory.
Get-ChildItem "C:\test_folder1" -File
The above command output is piped to the command below, which filters the files by the creation time less than X days from the current date.
where CreationTime -LT (Get-Date).AddDays(-15)
After that, it is piped to the Remove-Item
cmdlet to be deleted.
The final code to delete files older than X days should be like this:
Get-ChildItem "C:\test_folder1" | where CreationTime -LT (Get-Date).AddDays(-15) | Remove-Item
You can also use the LastWriteTime
of a file to remove the files having the last write time of X days older.
Get-ChildItem "C:\test_folder1" | where LastWriteTime -LT (Get-Date).AddDays(-15) | Remove-Item
If you want to delete all files at the directory, including sub-directories, you have to use the -Recurse
parameter.
Get-ChildItem "C:\test_folder1" -Recurse | where LastWriteTime -LT (Get-Date).AddDays(-15) | Remove-Item
The -Force
parameter allows you to delete files like hidden or read-only.
Get-ChildItem "C:\test_folder1" | where CreationTime -LT (Get-Date).AddDays(-15) | Remove-Item -Force
You can verify the deleted files in the file explorer or from the PowerShell console using the Get-ChildItem
cmdlet.
Get-ChildItem "C:\test_folder1" -Recurse
Output:
All files older than 15 days are deleted.
Directory: C:\test_folder1
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----- 2/23/2022 11:11 PM New folder
-a---- 2/23/2022 10:29 PM 0 books.txt
-a---- 2/23/2022 10:29 PM 0 hello.txt
Directory: C:\test_folder1\New folder
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 2/23/2022 10:26 PM 0 cars.txt
Using PowerShell cmdlets, you can easily find and delete files older than x
days in the specified directory.