How to Count Items in a Folder With PowerShell
-
Use the
Measure-Object
Cmdlet to Count Items in a Folder With PowerShell -
Use the
Get-ChildItem
and theCount
Property to Count Items in a Folder With PowerShell -
Use the
foreach
Loop to Count Items in a Folder With PowerShell - Conclusion
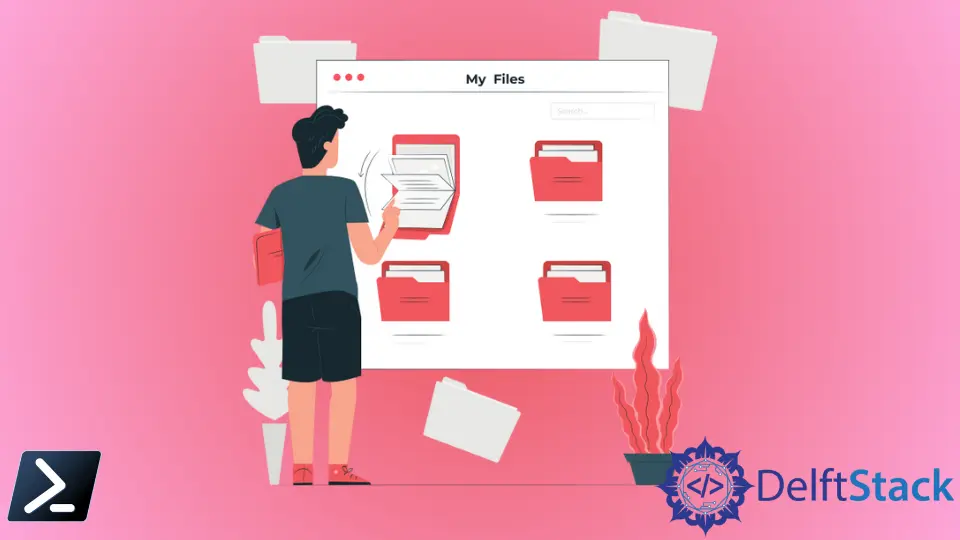
PowerShell supports different file and folder operations, such as creating, copying, moving, removing, and listing files and folders. Sometimes, you might need to know how many files and folders are present in the specific folder.
PowerShell offers several methods to accomplish this task, each with its advantages and use cases. One such method is using the Measure-Object
cmdlet, which provides a straightforward way to count items by measuring their properties.
This article explores how to utilize the Measure-Object
cmdlet to count items in a folder in PowerShell, along with other alternative methods for comparison.
Use the Measure-Object
Cmdlet to Count Items in a Folder With PowerShell
The Measure-Object
cmdlet calculates the property values of certain types of objects. You can use this cmdlet to count objects.
It also calculates the Average, Sum, Minimum, Maximum, and Standard Deviation of numeric values. You can count the number of string objects’ lines, words, and characters.
The following command counts the number of files and folders present in the path
directory. It counts all files and folders on the first level of the folder tree.
(Get-ChildItem 'path' | Measure-Object).Count
In this example, we’re using Get-ChildItem
to retrieve all items within the folder located at "path"
. This collection of items is then piped into Measure-Object
, which calculates various properties.
Since we’re interested in counting the items, we access the Count
property of the resulting object, providing us with the total count of items in the folder.
Output:
The Count
property only displays the count values.
The Count
property, as its name suggests, simply displays the count values obtained from the measurement.
For instance, if you wish to count only files within a directory, you can utilize the -File
parameter with Get-ChildItem
. This parameter instructs PowerShell to retrieve a list of files exclusively.
(Get-ChildItem -File 'path' | Measure-Object).Count
This command calculates the count of files present in the directory path
. The output, in this case, is 11
, indicating the total number of files found.
Output:
Similarly, if you want to count only folders, you can employ the -Directory
parameter:
(Get-ChildItem -Directory 'path' | Measure-Object).Count
In this example, PowerShell counts only the folders within the specified directory, returning a count of 6
.
Output:
The -Recurse
parameter in Get-ChildItem
gets the items recursively in the specified location. You can use the -Recurse
parameter to count files and folders recursively.
(Get-ChildItem -Recurse 'path' | Measure-Object).Count
This command counts all files and folders within the specified location, including those in sub-folders. The output, in this case, is 12082
, indicating the total count of items found recursively.
Output:
Use the Get-ChildItem
and the Count
Property to Count Items in a Folder With PowerShell
Counting items within a folder is a fundamental operation in PowerShell scripting, especially when managing files and directories. One straightforward approach to achieve this is by utilizing the Get-ChildItem
cmdlet along with the Count
property.
(Get-ChildItem -Path "path").Count
In this example, we’re using Get-ChildItem
to fetch all items within the folder located at "path"
. The collection of items returned by Get-ChildItem
is then piped to the .Count
property, which provides us with the total count of items in the folder.
Output:
Use the foreach
Loop to Count Items in a Folder With PowerShell
Counting items within a folder is a fundamental operation in PowerShell scripting, especially when managing files and directories. One straightforward approach to achieve this is by utilizing the Get-ChildItem
cmdlet along with the Count
property.
$folderPath = "path"
$count = 0
foreach ($item in (Get-ChildItem -Path $folderPath)) {
$count++
}
$count
In this example, we first define the $folderPath
variable to store the path of the folder we want to count items in. We initialize a counter variable, $count
, to zero.
Then, using a foreach
loop, we iterate through each item in the folder obtained by Get-ChildItem
. For each item encountered, we increment the $count
variable.
After the loop completes, we output the final count of items stored in $count
.
Output:
Conclusion
Counting items in a folder with PowerShell is a fundamental task for many scripting scenarios, from basic file management to complex automation workflows. By leveraging PowerShell’s versatile cmdlets like Measure-Object
and Get-ChildItem
and control structures like foreach
loops, scripters can efficiently determine the number of items within a folder.
Each method offers its advantages, allowing scripters to choose the approach that best suits their specific requirements and preferences. With the knowledge gained from this article, PowerShell users can confidently handle file and folder counting tasks in their scripting endeavors.