How to Convert a String to Datetime in PowerShell
-
Use
ParseExact
to Convert String toDateTime
in PowerShell -
Use the Explicit Conversion to Convert a String to
DateTime
in PowerShell -
Use
Get-Date
Cmdlet to Convert a String toDateTime
in PowerShell -
Use
Parse
to Convert a String toDateTime
in PowerShell - Conclusion
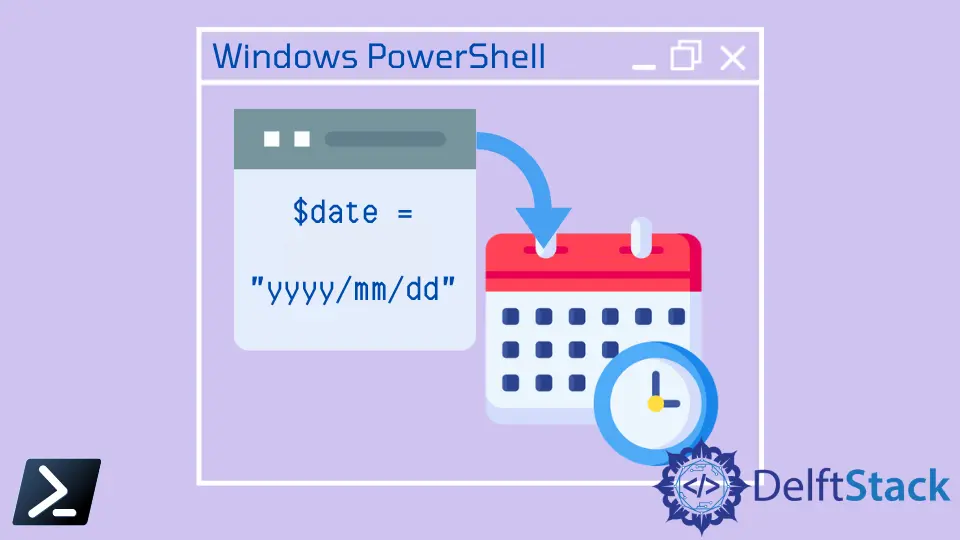
Converting string representations of dates and times to DateTime
objects is a common task in PowerShell scripting, often encountered when dealing with data from diverse sources or formats. In this technical guide, we explore various methods to achieve this task, each offering its unique advantages.
From the precision of the ParseExact
method to the simplicity of the explicit [DateTime]
conversion and the versatility of the Get-Date
cmdlet, we cover multiple approaches to suit different scenarios and preferences. Additionally, we delve into the powerful Parse
method, providing detailed explanations, syntax, and practical code examples to facilitate a comprehensive understanding of each method.
Use ParseExact
to Convert String to DateTime
in PowerShell
Converting a string representation of a date and time to a DateTime
object is a common task in PowerShell scripting, especially when dealing with data from various sources or formats. One approach to accomplish this is by using the ParseExact
method, which allows you to specify the exact format of the input string, providing more control over the conversion process.
$dateString = "2024-02-05"
$date = [datetime]::ParseExact($dateString, "yyyy-MM-dd", $null)
$date
In our provided PowerShell code snippet, we’re utilizing the ParseExact
method to convert a string representation of a date, "2024-02-05"
, into a DateTime
object. By specifying the exact format of the input string as "yyyy-MM-dd"
, we ensure precise conversion, unaffected by system date settings or cultural variations.
The resultant DateTime
object, stored in the $date
variable, accurately represents the parsed date, enabling seamless integration into subsequent operations. When we print $date
, we obtain the expected output, confirming the successful conversion.
Output:
Use the Explicit Conversion to Convert a String to DateTime
in PowerShell
The explicit [DateTime]
method in PowerShell is a type accelerator that allows for direct conversion of a string to a DateTime
object. When used, it instructs PowerShell to interpret the provided string as a DateTime
value without the need for additional parsing or formatting instructions.
This method is particularly useful when the input string follows a standard date format, such as ISO 8601
(e.g., "YYYY-MM-DD"
), as it simplifies the conversion process and enhances code readability.
$dateString = "2024-02-05"
[DateTime]$date = $dateString
$date
In this provided PowerShell code snippet, we are using the explicit [DateTime]
method to convert the string "2024-02-05"
to a DateTime
object. By simply assigning the string variable $dateString
to the variable $date
with the [DateTime]
type accelerator, PowerShell automatically converts the string to a DateTime
object.
When we print $date
, we obtain the expected output, confirming the successful conversion.
Output:
Use Get-Date
Cmdlet to Convert a String to DateTime
in PowerShell
In PowerShell, the Get-Date
cmdlet serves not only to fetch the current date and time but also to convert a string representation of a date and time into a DateTime
object. This flexibility makes Get-Date
a handy tool for handling date-related operations in PowerShell scripts.
When provided with a string argument, Get-Date
automatically attempts to parse it into a DateTime
object, making it convenient for converting date strings of various formats into a standardized DateTime
format.
$dateString = "2024-02-05"
[DateTime]$date = $dateString
$date
In the given code snippet, we have a string variable $dateString
containing the date in the format "YYYY-MM-DD"
. Utilizing the Get-Date
cmdlet, we convert this string into a DateTime
object and assign it to the variable $date
.
We rely on the Get-Date
cmdlet to automatically interpret the string $dateString
as a date and parse it into the corresponding DateTime
object.
Output:
Use Parse
to Convert a String to DateTime
in PowerShell
In PowerShell, the Parse
method is a powerful tool for converting a string representation of a date and time into a DateTime
object. This method provides precise control over the parsing process, allowing the user to specify the exact format of the input string.
By defining the format explicitly, the Parse
method ensures accurate conversion, regardless of the system’s regional settings or cultural variations.
$dateString = "2024-02-05"
$date = [datetime]::Parse($dateString)
$date
In this example, we demonstrate how to use the Parse
method to convert a string representation of a date into a DateTime
object in PowerShell. By invoking the Parse
method on the [datetime]
type accelerator, we parse the input string $dateString
and store the result in the variable $date
.
Output:
Conclusion
PowerShell offers several methods for converting strings to DateTime
objects, each catering to different requirements and preferences. Whether you need precise control over formatting with ParseExact
, simplicity with explicit [DateTime]
conversion, automatic parsing with Get-Date
, or fine-grained control with Parse
, PowerShell provides the flexibility and functionality necessary for efficient date and time manipulation.
By mastering these methods and understanding their nuances, PowerShell users can streamline their scripts and handle date-related tasks effectively, enhancing productivity and ensuring accurate data processing.