How to Get Date and Time Using PowerShell
- Get Date and Time in PowerShell
- Date Arithmetic Using DateTime Methods in PowerShell
- Compare Dates in PowerShell
- Convert Strings to DateTime Object in PowerShell
-
DateTime
Methods in PowerShell -
Format
DateTime
Objects in PowerShell
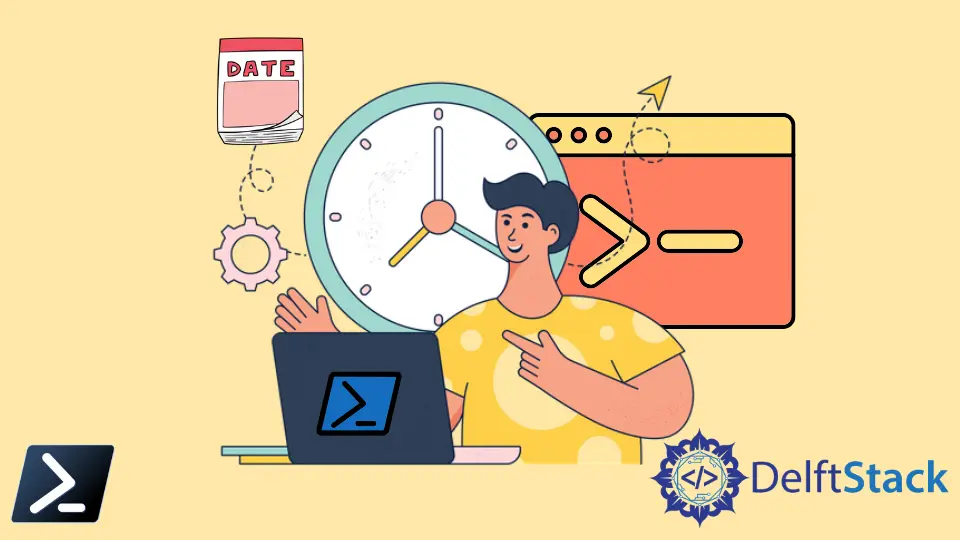
PowerShell can do many things, and, like any suitable programming language, it can do just about anything we want with date and time. Using the Windows PowerShell Get-Date
cmdlet and other techniques, you can find today’s date, tomorrow’s date, format dates, etc.
This article will explain how we can get the current date and time and how the DateTime
object works in PowerShell.
Get Date and Time in PowerShell
One of the ways to discover the current date and time with Windows PowerShell is using the Get-Date
command. This cmdlet displays the current date and time.
Get-Date
Output:
Wednesday, 9 March 2022 8:14:34 pm
By default, the Get-Date
command looks like it only returns the current date and time, but, in reality, it’s producing a lot more information. To find this information, pipe the Format-List
cmdlet output below.
Get-Date | Format-List
Output:
DisplayHint : DateTime
Date : 08/03/2022 12:00:00 am
Day : 8
DayOfWeek : Wednesday
DayOfYear : 68
Hour : 20
Kind : Local
Millisecond : 671
Minute : 18
Month : 3
Second : 1
Ticks : 637824538816714508
TimeOfDay : 20:18:01.6714508
Year : 2022
DateTime : Wednesday, 9 March 2022 8:18:01 pm
You can also use the Get-Member
command to find all the object properties by running the Get-Date | Get-Member
command.
If we check the type of object Get-Date | Get-Member
returns, you’ll notice a System.DateTime
object type. This class exposes all of the properties and methods we see.
We can discover its object type by using the below command.
(Get-Date).GetType().FullName
Output:
System.DateTime
Once we see all available properties, we can reference them with dot(.)
notation, as shown below.
(Get-Date).Year
(Get-Date).DayOfWeek
(Get-Date).Month
(Get-Date).DayOfYear
Output:
2022
Wednesday
3
68
Date Arithmetic Using DateTime Methods in PowerShell
The System.DateTime
object that Get-Date
returns have various methods you can invoke to add or remove chunks of time. For example, if we run Get-Date | Get-Member
, we will see different techniques that start with Add
.
Get-Date | Get-Member | where { $_.Name -like "Add*" }
Output:
TypeName: System.DateTime
Name MemberType Definition
---- ---------- ----------
Add Method datetime Add(timespan value)
AddDays Method datetime AddDays(double value)
AddHours Method datetime AddHours(double value)
AddMilliseconds Method datetime AddMilliseconds(double value)
AddMinutes Method datetime AddMinutes(double value)
AddMonths Method datetime AddMonths(int months)
AddSeconds Method datetime AddSeconds(double value)
AddTicks Method datetime AddTicks(long value)
AddYears Method datetime AddYears(int value)
You’ll see a few invoking these methods and their output in the following commands.
#Adding 8 days to the current date
(Get-Date).AddDays(8)
#Adding 3 hours to the current time
(Get-Date).AddHours(3)
#Adding five years to the current date
(Get-Date).AddYears(5)
#Subtracting 7 days from the current date using a negative number.
(Get-Date).AddDays(-7)
Output:
Thursday, 17 March 2022 8:28:57 pm
Wednesday, 9 March 2022 11:28:57 pm
Tuesday, 9 March 2027 8:28:57 pm
Tuesday, 1 March 2022 8:28:57 pm
Compare Dates in PowerShell
You can also compare dates using standard PowerShell operators. For example, PowerShell knows when a date is “less than” (earlier than) or “greater than” (later than) another date.
To compare dates, create two DateTime
objects using Get-Date
or perhaps by casting strings with [DateTime]
and then using standard PowerShell operators like -lt
or -gt
. We can see a simple example of comparing dates below.
#Declaring the date
$Date1 = (Get-Date -Month 10 -Day 14 -Year 2021)
$Date2 = Get-Date
$Date1 -lt $Date2
Output:
True
Convert Strings to DateTime Object in PowerShell
To convert a string variable to a DateTime
object, preface the string (or variable) with the [DateTime]
data type. When we do this, Windows PowerShell tries to interpret the string as a date and time and then provide all of the properties and methods available on this object type.
$Date = "2020-09-07T13:35:08.4780000Z"
[DateTime]$Date
Output:
Monday, 7 September 2020 5:35:08 pm
DateTime
Methods in PowerShell
One way to manipulate how a DateTime
object is displayed in the console is by using several methods on the DateTime
object. The DateTime
object has four methods to manipulate the formatting.
ToLongDateString()
ToShortDateString()
ToLongTimeString()
ToShortTimeString()
Let’s see an examples using the ToShortDateString()
and ToShortTimeString()
methods.
[DateTime]$Date = $Date
$date.ToShortDateString() + " " + $date.ToShortTimeString()
Output:
9/7/2020 7:05 PM
Format DateTime
Objects in PowerShell
To change the date and time format of a DateTime
object, use the Get-Date
command to generate the object and the -Format
parameter to change the layout. The -Format
parameter accepts a string of characters, representing how a date/time string should look.
Get-Date -Format "dddd dd-MM-yyyy HH:mm K"
Output:
Wednesday 09-03-2022 21:21 +04:00
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn