How to Parse Datetime by ParseExact in PowerShell
-
Use the
ParseExact
Method to ParseDateTime
in PowerShell -
Use the Explicit Type Conversion to Parse
DateTime
in PowerShell - Conclusion
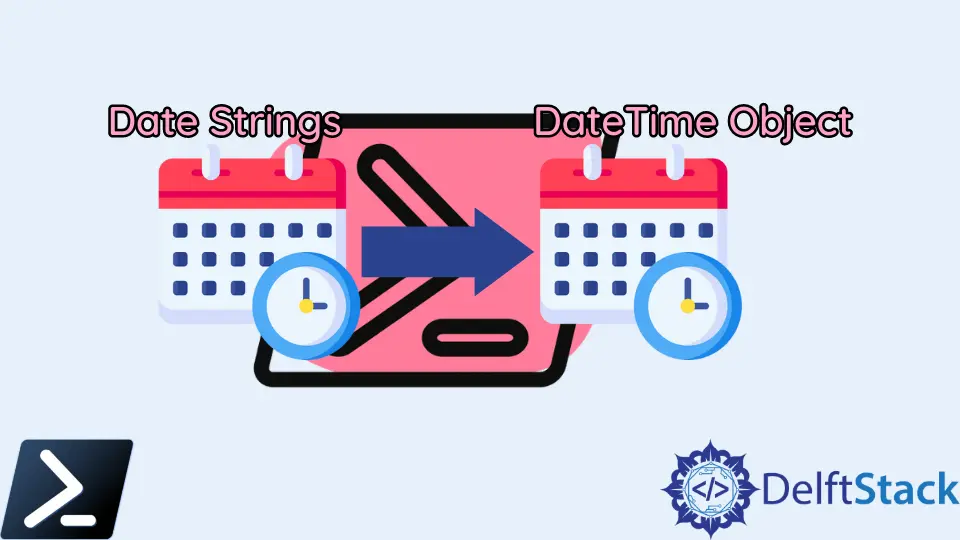
While working with dates on PowerShell, there are times when you will need to convert the date string to a DateTime
object. You cannot use date strings to perform DateTime
operations; you will need the DateTime
object.
This conversion is crucial because date strings by themselves are not sufficient for performing DateTime
operations. Our tutorial is designed to bridge this gap by providing comprehensive guidance on how to parse and convert strings to the DateTime
format in PowerShell.
Use the ParseExact
Method to Parse DateTime
in PowerShell
The ParseExact
method of the DateTime
class converts the date and time string to the DateTime
format. The format of a date and time string pattern must match the specified format of the DateTime
object.
The syntax for ParseExact
is as follows:
[datetime]::ParseExact(string, format, provider)
Parameters:
string
: The date and time in string format that you want to convert.format
: The exact format specifier that defines the expected format of the string.provider
: An object that supplies culture-specific formatting information. This can benull
if using the current culture.
Simple Date Parsing
The following example converts the date string to a DateTime
object using the ParseExact
method.
$dateString = '24-Jan-2024'
$format = 'dd-MMM-yyyy'
$culture = $null # Using the current culture
# Parsing the date
$parsedDate = [datetime]::ParseExact($dateString, $format, $culture)
# Displaying the result
Write-Host "Parsed Date: $parsedDate"
In this code, we first define a date string 24-Jan-2024
, which represents a date in a day-month-year format with an abbreviated month name. We specify this format by the dd-MMM-yyyy
format specifier.
The $culture
variable is set to $null
, which tells PowerShell to use the current culture setting of the system for parsing the date.
The core function here is [datetime]::ParseExact($dateString, $format, $culture)
, which parses the $dateString
according to the $format
we specified. After parsing, the string is converted into a DateTime
object, which we store in the $parsedDate
variable.
Finally, we use Write-Host
to display the parsed date. This is a simple yet effective demonstration of using ParseExact
in a real-world scenario.
Output:
This output shows the DateTime
object that has been created from the string, including the time set to midnight (00:00:00
) by default, as no time was specified in the input string.
Using a Specific Culture
Using a specific culture with ParseExact
is essential when dealing with date strings that are formatted according to the conventions of a culture different from the current system setting. This approach prevents misinterpretation of dates, such as confusing the month and day in MM/dd/yyyy
and dd/MM/yyyy
formats.
Here is a simple example:
$dateString = '23/01/2024'
$format = 'dd/MM/yyyy'
$culture = [Globalization.CultureInfo]::CreateSpecificCulture('fr-FR')
# Parsing the date
$parsedDate = [datetime]::ParseExact($dateString, $format, $culture)
# Displaying the result
Write-Host "Parsed Date: $parsedDate"
In this code snippet, we aim to parse a date string that follows the French date format dd/MM/yyyy
. We first define the date string 23/01/2024
.
Notice that the format dd/MM/yyyy
could easily be confused with the MM/dd/yyyy
format used in the United States. To correctly interpret the date as the 23rd of January, not the 1st of March, we specify the French culture ('fr-FR')
.
The $culture
variable is instantiated using [Globalization.CultureInfo]::CreateSpecificCulture('fr-FR')
, which prepares the cultural context for our parsing operation. We then call [datetime]::ParseExact($dateString, $format, $culture)
to parse the date string.
Finally, we use Write-Host
to display the parsed date, which illustrates how the input string is correctly interpreted in the context of the specified culture.
Output:
This output demonstrates the successful parsing of the date string in the context of French culture. The date is correctly understood as the 23rd of January 2024, and not misinterpreted due to different month-day ordering.
Parsing With Time
In PowerShell, efficiently handling dates and times is a crucial skill, especially when it comes to parsing strings that include both date and time components. The ParseExact
method of the DateTime
class is adept at interpreting such strings, provided that the format is specified precisely.
Let’s parse a datetime string that includes both date and time:
$dateTimeString = '2024-01-23 14:30'
$format = 'yyyy-MM-dd HH:mm'
$provider = $null # Opting for the invariant culture
# Parsing the datetime
$parsedDateTime = [datetime]::ParseExact($dateTimeString, $format, $provider)
# Displaying the result
Write-Host "Parsed DateTime: $parsedDateTime"
In our example, we handle a datetime string 2024-01-23 14:30
, formatted in an international standard (year-month-day and 24-hour time). We meticulously set the $format
to yyyy-MM-dd HH:mm
to align with this structure.
We choose $null
for the $provider
, signifying our use of the invariant culture. This approach is typical when the datetime format is standard and not culturally dependent.
The pivotal moment is when we execute [datetime]::ParseExact($dateTimeString, $format, $provider)
, transforming the string into a DateTime
object that embodies both the date and the time from the string.
Output:
This result effectively illustrates that our input string has been accurately parsed into a DateTime
object, capturing both date and time components.
Use the Explicit Type Conversion to Parse DateTime
in PowerShell
You can also cast the string of a date and time to the DateTime
format in PowerShell.
Using this syntax, you can cast a string to the DateTime
object.
[DateTime]string
The following example converts the string representation of a date and time to the DateTime
object with the cast expression.
$strDate = "2022-06-11 09:22:40"
[DateTime]$strDate
In our PowerShell code snippet, we assign the string "2022-06-11 09:22:40"
to the variable $strDate
. This string represents a date and time in a standard format.
Following this, we perform a type conversion to a DateTime
object using [DateTime]$strDate
.
Output:
With the DateTime
object, you should be able to perform any DateTime
operations. We hope this tutorial helps you understand how to convert strings to the DateTime
format in PowerShell.
Conclusion
Throughout this tutorial, we’ve explored the diverse capabilities of PowerShell in handling DateTime
conversions, focusing on the ParseExact
method and its versatility in parsing strings into DateTime
objects. We’ve demonstrated how to handle simple date formats, incorporate specific cultural contexts, and parse strings that include both date and time components.
Additionally, we delved into the straightforward yet effective technique of explicit type conversion, providing a quick method for standard date formats. Our examples have shown the importance of understanding and correctly applying different parsing methods to ensure accurate and efficient date-time manipulation in PowerShell scripts.