How to Concatenate Files Using PowerShell
-
Use
Get-Content
andSet-Content
to Concatenate Files Using PowerShell -
Use
Add-Content
to Concatenate Files Using PowerShell -
Use
Out-File
to Concatenate Files Using PowerShell - Use Concatenate Operator to Concatenate Files Using PowerShell
- Conclusion
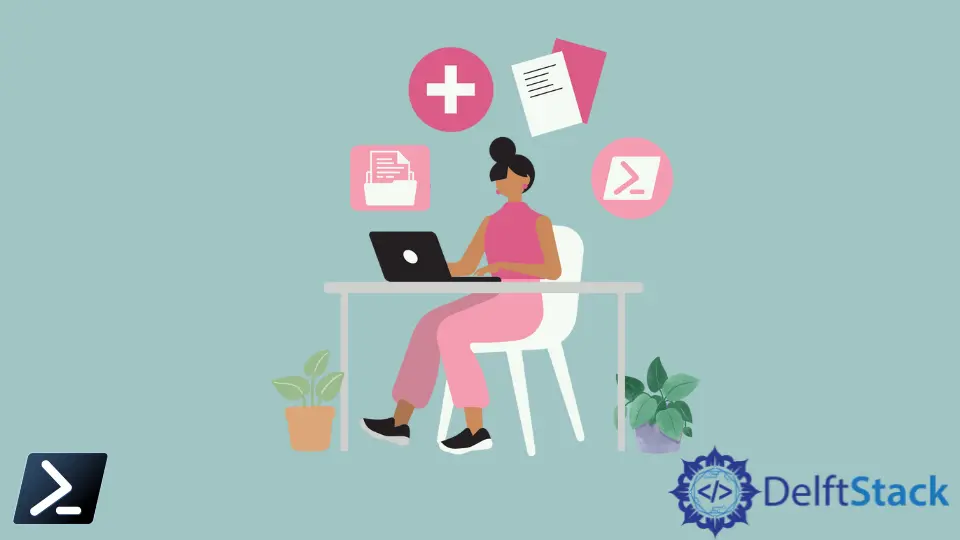
PowerShell allows you to perform different file operations, such as creating, copying, moving, removing, viewing, and renaming files. Another important feature lets you concatenate multiple files into a single file.
In this article, we will explore four methods to easily merge the multiple files’ content into a single file: using Get-Content
and Set-Content
, using the Add-Content
cmdlet, using the Out-File
cmdlet, and using the concatenate operator.
Use Get-Content
and Set-Content
to Concatenate Files Using PowerShell
This method utilizes Get-Content
to read the contents of multiple files (file1.txt
and file2.txt
) and then pipes the combined content to Set-Content
to write it to a new file (concatenated.txt
).
The Set-Content
is a string-processing cmdlet in PowerShell. It writes new content or replaces the content in a file.
file1.txt
:
This is a file1 text file.
file2.txt
:
This is a file2 text file.
Code:
Get-Content file1.txt, file2.txt | Set-Content concatenated.txt
In this command, the Get-Content file1.txt, file2.txt
reads the contents of file1.txt
and file2.txt.
Then, as we see the vertical line |
, this is the pipe operator, which passes the output (contents of file1.txt
and file2.txt
) to the next part of the command.
Lastly, the Set-Content concatenated.txt
command sets the content of the concatenated files into a new file named concatenated.txt
.
Check the content of a concatenated.txt
file using Get-Content
. Use the command sample below.
Get-Content concatenated.txt
The Get-Content
command reads the contents of the specified file and outputs them to the console. If concatenated.txt
is in the current directory, the command will display its content.
Make sure to replace concatenated.txt
with the actual path to your file if it’s not in the current directory.
We will also use Get-Content
in the following methods that will be discussed to check and verify the file content if it works correctly.
Output:
This is a file1 text file.
This is a file2 text file.
It will show the merged content of two files and automate the new line per file.
Use Add-Content
to Concatenate Files Using PowerShell
Here, Get-Content
is used to read the content of each file separately. The Add-Content
cmdlet then appends the content to the specified file (concatenated2.txt
). This process is repeated for each file.
file1.txt
:
This is a file1 text file.
file2.txt
:
This is a file2 text file.
Code:
Get-Content file1.txt | Add-Content -Path concatenated2.txt
Get-Content file2.txt | Add-Content -Path concatenated2.txt
This command reads the contents of file1.txt
and file2.txt
and appends them to the end of the existing concatenated2.txt
file.
The |
(pipe) operator passes the combined content of the two files to the Add-Content
cmdlet.
Note: Make sure the file paths are correct and that you have the necessary permissions to read from
file1.txt
andfile2.txt
and write toconcatenated2.txt
.
Check the content of a concatenated2.txt
file using Get-Content
.
Get-Content concatenated2.txt
Output:
This is a file1 text file.
This is a file2 text file.
It will show the merged content of two files and automate the new line per file.
Use Out-File
to Concatenate Files Using PowerShell
The Out-File
cmdlet sends the output to a file. If the file does not exist, it creates a new file in the specified path.
To concatenate files with Out-File
, you will need to use the Get-Content
cmdlet to get the content of a file. Normally, Get-Content
displays the output in the console.
You have to pipe its output to Out-File
, so it sends the output to a specified file. The following example merges two files’ content into a concatenated3.txt
.
file1.txt
:
This is a file1 text file.
file2.txt
:
This is a file2 text file.
Code:
Get-Content file1.txt, file2.txt | Out-File concatenated3.txt
The command above will overwrite the content of concatenated3.txt
with the combined content of file1.txt
and file2.txt.
If concatenated3.txt
doesn’t exist, it will be created.
To compare with the previous method, Add-Content
appends the existing file while Out-File
overwrites it.
Run the following command to verify the content of a concatenated3.txt
file.
Get-Content concatenated3.txt
Output:
This is a test1 file.
This is a test2 file.
As you can see, the content of both test1.txt
and test2.txt
are copied to concatenated3.txt
.
If you want to concatenate all .txt
files in the directory, you can use *.txt
to select all files with the .txt
extension in the directory.
Get-Content *.txt | Out-File concatenated3.txt
Use Concatenate Operator to Concatenate Files Using PowerShell
In this method, the +
operator concatenates the contents of file1.txt
and file2.txt
before passing them to Set-Content
for writing to the destination file (concatenated4.txt
).
file1.txt
:
This is a file1 text file.
file2.txt
:
This is a file2 text file.
Code:
(Get-Content file1.txt -Raw) + "`r`n" + (Get-Content file2.txt -Raw) | Set-Content concatenated4.txt
The + "`r`n" +
part is used to concatenate strings.
In this case, it adds a carriage return ("r")
and a newline ("n")
character between the content of file1.txt
and file2.txt
. This ensures that the contents of the two files will be on separate lines when combined.
Check the content of a concatenated4.txt
file using Get-Content
.
Get-Content concatenated4.txt
Output:
This is a file1 text file.
This is a file2 text file.
It will show the merged content of two files and is supported by the + "`r`n" +
to add a new line per file.
Conclusion
Concatenating files in PowerShell is a versatile task, and the choice of method depends on your specific requirements.
Whether you prefer the simplicity of the Get-Content
and Set-Content
pipeline, the explicit nature of Add-Content
and Out-File
, or the concise syntax of the concatenate operator, PowerShell provides multiple options to suit your needs.
Understanding these methods empowers you to efficiently manipulate and manage file contents in various scenarios.