How to Append Data to File Using PowerShell
-
the
Add-Content
Basic Syntax in PowerShell -
Use the
Add-Content
to Append Text to File in PowerShell - Use the (`n) Operator to Append Data on New Line in PowerShell
-
Use the
Add-Content
Command to Add Content of One File to Another File in PowerShell -
Use the
Add-Content
Command to Append Data to Read-Only File in PowerShell
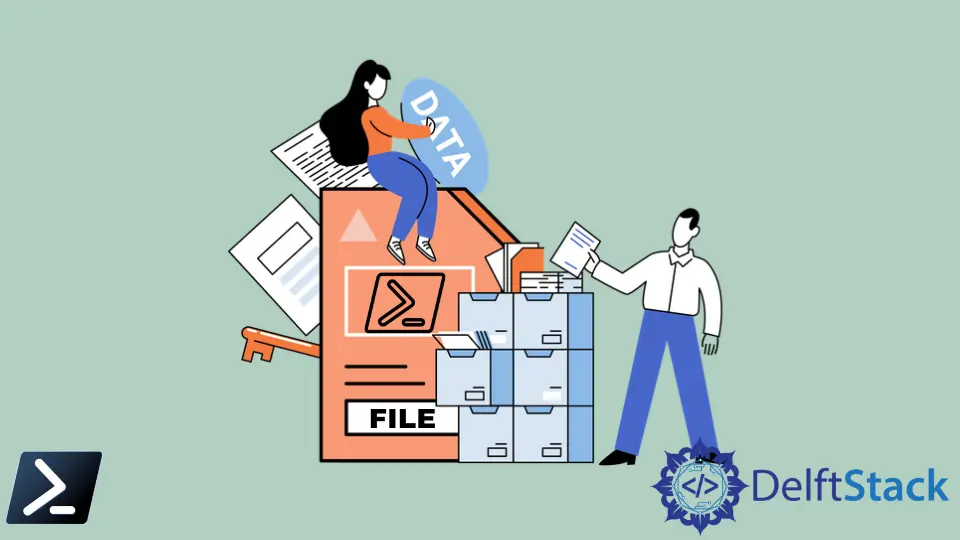
The Add-Content
command in PowerShell adds content to a file. We can specify content in the command or Get-Content
to get the file’s content for appending.
The Add-Content
cmdlet appends text to the file or appends a string to the file.
the Add-Content
Basic Syntax in PowerShell
The Add-Content
cmdlet in Windows PowerShell appends content to file and appends text to file.
Add-Content
[-Path] <string[]>
[-Value] <Object[]>
[-PassThru]
[-Filter <string>]
[-Include <string[]>]
[-Exclude <string[]>]
[-Force]
[-Credential <pscredential>]
[-WhatIf]
[-Confirm]
[-NoNewline]
[-Encoding <Encoding>]
[-AsByteStream]
[-Stream <string>]
[<CommonParameters>]
Use the Add-Content
to Append Text to File in PowerShell
For example, you have the Get-DateFile.txt
text file in the directory.
Create a new file with Get-DateFile.txt
, and add some test data.
Get-DataFile.txt:
Example illustration about appending text to
Add-Content -Path .\Get-DateFile.txt "End of file"
The Add-Content
cmdlet appends the End of file
string to the end of the file specified by the -Path
parameter in the current directory.
Output:
Example illustration about appending text to End of file
Use the (`n) Operator to Append Data on New Line in PowerShell
To append data to a file on a new line, use the new line operator (`n).
Add-Content -Path .\Get-DateFile.txt "`nSecond line starts here..."
Output:
Example illustration about appending text to End of file
Second line starts hereā¦
Use the Add-Content
Command to Add Content of One File to Another File in PowerShell
The Add-Content
command will append one file’s content to another.
It will read and assign file contents to a variable and write content to the destination file.
The Add-Content
command will create a new file if the file doesn’t exist while adding the text to the file.
# Read file contents to variable
$sourceFileContent = Get-Content -Path .\GetFileProperties.txt
# This line will append the content of one file to another file
# If the file does not exist, the Add-Content command will create a new file
Add-Content -Path .\Destination_File.txt -Value $sourceFileContent
To append the content of one file to another file, the Get-Content
cmdlet in PowerShell gets the file’s content specified by the Path
parameter.
Then, it reads the file’s contents and stores them in a variable $sourceFileContent
.
Add-Content
cmdlet in PowerShell appends the source file’s content specified in the -Value
parameter.
The Add-Content
command will create a new file if the file doesn’t exist and copy the content.
Use the Add-Content
Command to Append Data to Read-Only File in PowerShell
# Create a new file
New-Item -Path .\TempROFile.txt -ItemType File
# Set file as read-only
Set-ItemProperty -Path .\TempROFile.txt -Name IsReadOnly -Value $True
# Get file details
Get-ChildItem -Path .\TempROFile.txt
# Appends the line to file
Add-Content -Path .\TempROFile.txt -Value 'End of File' -Force
The first command creates a new file using PowerShell’s New-Item
cmdlet.
The Set-ItemProperty
command in PowerShell is used to set the IsReadOnly
property to true for the specified file.
The Get-ChildItem
command in PowerShell gets specified file details such as Name
, LastWriteTime
, Length
, and mode
.
The Add-Content
cmdlet appends a line to a read-only file specified by the -Path
parameter.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn