How to Concatenate Strings in PHP
- Use the Concatenation Operator to Concatenation Strings in PHP
- Use the Concatenation Assignment Operator to Concatenate Strings in PHP
-
Use the
sprintf()
Function to Concatenate Strings in PHP
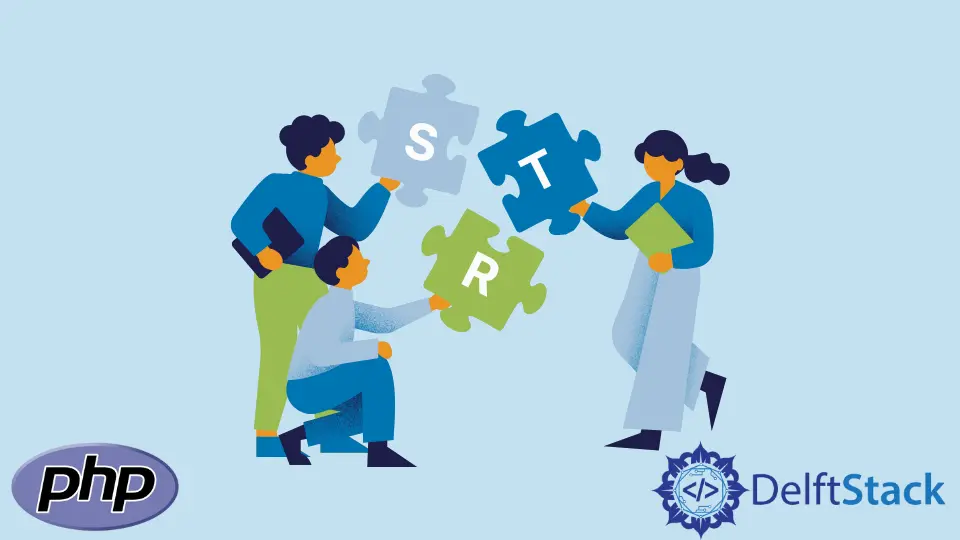
This article will introduce different methods to perform string concatenation in PHP.
Use the Concatenation Operator to Concatenation Strings in PHP
The process of joining two strings together is called the concatenation process. In PHP, we can achieve this by using the concatenation operator. The concatenation operator is .
. The correct syntax to use this operator is as follows.
$finalString = $string1 . $string2;
The details of these variables are as follows.
Variables | Description |
---|---|
$finalString |
It is the string in which we will store the concatenated strings. |
$string1 |
It is the string that we want to concatenate with the other string. |
$string2 |
It is the string that we want to concatenate with the first string. |
The program below shows how we can use the concatenation operator to combine two strings.
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string.";
$finalString = $mystring1 . $mystring2;
echo($finalString);
?>
Output:
This is the first string. This is the second string.
Likewise, we can use this operator to combine multiple strings.
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string. ";
$mystring3 = "This is the third string. ";
$mystring4 = "This is the fourth string. ";
$mystring5 = "This is the fifth string.";
$finalString = $mystring1 . $mystring2 . $mystring3 . $mystring4 . $mystring5;
echo($finalString);
?>
Output:
This is the first string. This is the second string. This is the third string. This is the fourth string. This is the fifth string.
Use the Concatenation Assignment Operator to Concatenate Strings in PHP
In PHP, we can also use the concatenation assignment operator to concatenate strings. The concatenation assignment operator is .=
. The difference between .=
and .
is that the concatenation assignment operator .=
appends the string on the right side. The correct syntax to use this operator is as follows.
$string1 .= $string2;
The details of these variables are as follows.
Variables | Description |
---|---|
$string1 |
It is the string with which we want to append a new string on the right side. |
$string2 |
It is the string that we want to concatenate with the first string. |
The program below shows how we can use the concatenation assignment operator to combine two strings.
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string.";
$mystring1 .= $mystring2;
echo($mystring1);
?>
Output:
This is the first string. This is the second string.
Likewise, we can use this operator to combine multiple strings.
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string. ";
$mystring3 = "This is the third string. ";
$mystring4 = "This is the fourth string. ";
$mystring5 = "This is the fifth string.";
$mystring1 .= $mystring2 .= $mystring3 .= $mystring4 .= $mystring5;
echo($mystring1);
?>
Output:
This is the first string. This is the second string. This is the third string. This is the fourth string. This is the fifth string.
Use the sprintf()
Function to Concatenate Strings in PHP
In PHP, we can also use the sprintf()
function to concatenate strings. This function gives several formatting patterns to format strings. We can use this formatting to combine two strings. The correct syntax to use this function is as follows.
sprintf($formatString, $string1, $string2, ..., $stringN)
The function sprintf()
accepts N+1 parameters. The detail of its parameters is as follows.
Parameters | Description | |
---|---|---|
$formatString |
mandatory | The format will be applied to the given string or strings. |
$string1 , $string2 , $stringN |
mandatory | It is the string we want to format. At least one string is mandatory. |
The function returns the formatted string. We will use the format %s %s
to combine two strings. The program that combines two strings is as follows:
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string";
$finalString = sprintf("%s %s", $mystring1, $mystring2);
echo($finalString);
?>
Output:
This is the first string. This is the second string.