PHP での文字列連結
Minahil Noor
2023年1月30日
PHP
PHP String
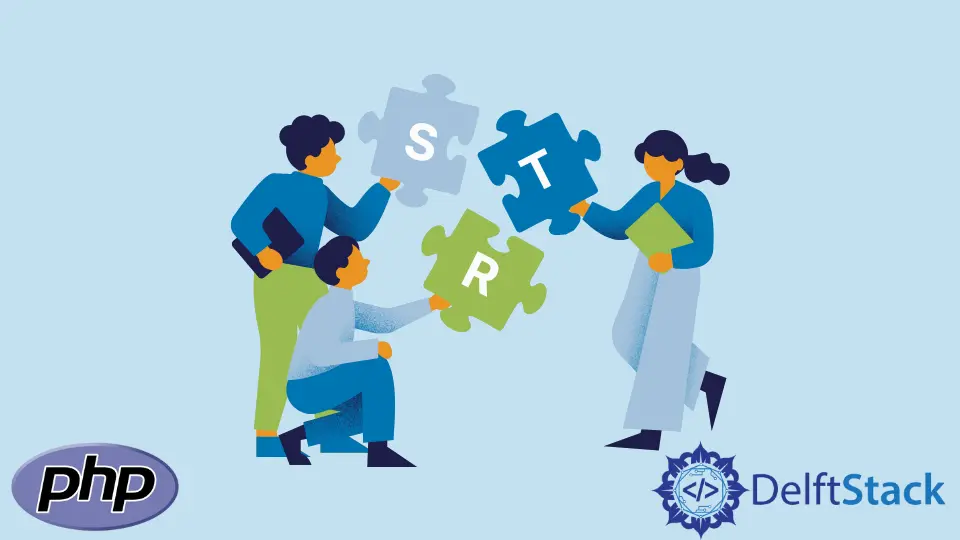
この記事では、PHP で文字列連結を行うためのさまざまな方法を紹介します。
PHP で文字列連結演算子を使用して文字列を連結する
2つの文字列を結合する処理を連結処理といいます。PHP では、連結演算子を使用することでこれを実現します。連結演算子は .
です。この演算子を使用するための正しい構文は以下の通りです。
$finalString = $string1 . $string2;
これらの変数の詳細は以下の通りです。
変数の内容 | 説明 |
---|---|
$finalString |
これは連結された文字列を格納する文字列です。 |
$string1 |
他の文字列と連結したい文字列です。 |
$string2 |
最初の文字列と連結させたい文字列です。 |
以下のプログラムは、コンカチネーション演算子を使って 2つの文字列を結合する方法を示しています。
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string.";
$finalString = $mystring1 . $mystring2;
echo($finalString);
?>
出力:
This is the first string. This is the second string.
同様に、この演算子を使って複数の文字列を結合することができます。
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string. ";
$mystring3 = "This is the third string. ";
$mystring4 = "This is the fourth string. ";
$mystring5 = "This is the fifth string.";
$finalString = $mystring1 . $mystring2 . $mystring3 . $mystring4 . $mystring5;
echo($finalString);
?>
出力:
This is the first string. This is the second string. This is the third string. This is the fourth string. This is the fifth string.
PHP で文字列を連結するために連結代入演算子を使用する
PHP では、連結代入演算子を使って文字列を連結することもできます。連結代入演算子は .=
です。.=
と .
の違いは、連結代入演算子 .=
が文字列を右側に追加する点です。この演算子を使用する正しい構文は以下の通りです。
$string1 .= $string2;
これらの変数の詳細は以下の通りです。
変数 | 説明 |
---|---|
$string1 |
右側に新しい文字列を追加したい文字列です。 |
$string2 |
最初の文字列と連結したい文字列です。 |
以下のプログラムは、連結代入演算子を使って 2つの文字列を連結する方法を示しています。
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string.";
$mystring1 .= $mystring2;
echo($mystring1);
?>
出力:
This is the first string. This is the second string.
同様に、この演算子を使って複数の文字列を結合することができます。
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string. ";
$mystring3 = "This is the third string. ";
$mystring4 = "This is the fourth string. ";
$mystring5 = "This is the fifth string.";
$mystring1 .= $mystring2 .= $mystring3 .= $mystring4 .= $mystring5;
echo($mystring1);
?>
出力:
This is the first string. This is the second string. This is the third string. This is the fourth string. This is the fifth string.
PHP で文字列を連結するには sprintf()
関数を使用する
PHP では、文字列を連結するために sprintf()
関数を使用することもできます。この関数は、文字列をフォーマットするためのいくつかのフォーマットパターンを提供しています。この書式を使って、2つの文字列を連結することができます。この関数を使用するための正しい構文は以下の通りです。
sprintf($formatString, $string1, $string2, ..., $stringN)
関数 sprintf()
は N+1 個のパラメータを受け取ります。パラメータの詳細は以下の通りです。
パラメータ | 説明 | |
---|---|---|
$formatString |
強制的 | フォーマットは、与えられた文字列または文字列に適用されます。 |
$string1 、$string2 、$stringN |
強制的 | フォーマットしたい文字列です。少なくとも 1つの文字列は必須です。 |
この関数はフォーマットされた文字列を返します。2つの文字列を結合するには、%s %s
という書式を用います。2つの文字列を結合するプログラムは以下の通りです。
<?php
$mystring1 = "This is the first string. ";
$mystring2 = "This is the second string";
$finalString = sprintf("%s %s", $mystring1, $mystring2);
echo($finalString);
?>
出力:
This is the first string. This is the second string.
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe