How to Replace String in PHP
-
Understanding the
str_replace()
Function - Example 1: Basic String Replacement
- Example 2: Replacing Multiple Substrings
- Example 3: Case Sensitivity in Replacements
- Example 4: Replacing Special Characters
- Conclusion
- FAQ
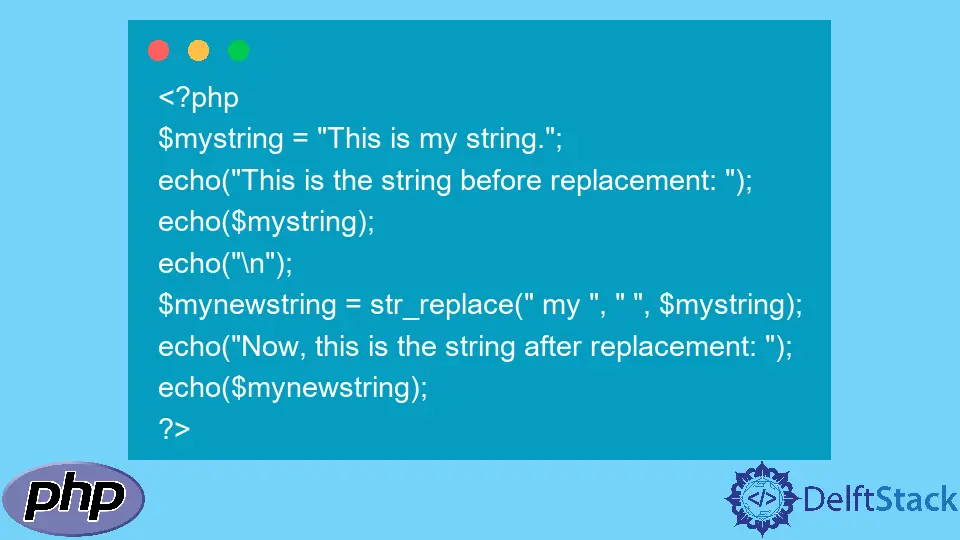
Replacing parts of a string is a common task in programming, and in PHP, it’s both straightforward and efficient. Whether you’re cleaning up user input, formatting data, or simply altering text, knowing how to replace strings will enhance your coding skills.
In this article, we’ll delve into the str_replace()
function, one of PHP’s most powerful tools for string manipulation. We’ll explore how to use it effectively with practical examples that you can apply in your projects. By the end of this guide, you’ll have a solid understanding of how to replace strings in PHP, making your code cleaner and more efficient.
Understanding the str_replace()
Function
The str_replace()
function in PHP is designed to search for specific substrings within a string and replace them with new content. This function is particularly useful for tasks like sanitizing user input or modifying text for display. The syntax for str_replace()
is as follows:
str_replace($search, $replace, $subject);
- $search: The substring you want to find.
- $replace: The substring you want to use as a replacement.
- $subject: The original string where the replacement will occur.
Let’s look at some examples to see how this works in practice.
Example 1: Basic String Replacement
Here’s a simple example that demonstrates how to replace a word in a string.
<?php
$originalString = "Hello World!";
$search = "World";
$replace = "PHP";
$newString = str_replace($search, $replace, $originalString);
echo $newString;
?>
Output:
Hello PHP!
In this example, we start with the string “Hello World!” and replace “World” with “PHP”. The result is “Hello PHP!”. This basic use case showcases how easy it is to change specific parts of a string using str_replace()
.
Example 2: Replacing Multiple Substrings
The str_replace()
function can also handle multiple replacements at once. This feature is particularly useful when you need to make several changes in a single pass.
<?php
$originalString = "I love PHP. PHP is great!";
$search = array("PHP", "great");
$replace = array("Python", "awesome");
$newString = str_replace($search, $replace, $originalString);
echo $newString;
?>
Output:
I love Python. Python is awesome!
Here, we replaced both “PHP” with “Python” and “great” with “awesome”. The function takes arrays for both the search and replace parameters, allowing for efficient batch processing. This is particularly beneficial when dealing with large datasets or user inputs.
Example 3: Case Sensitivity in Replacements
PHP’s str_replace()
is case-sensitive. If you need to replace a substring regardless of its case, you should use str_ireplace()
, which performs a case-insensitive replacement.
<?php
$originalString = "Hello World!";
$search = "world";
$replace = "PHP";
$newString = str_ireplace($search, $replace, $originalString);
echo $newString;
?>
Output:
Hello PHP!
In this case, “world” is replaced by “PHP” even though the original string has “World” with an uppercase “W”. Using str_ireplace()
is a great way to ensure that your replacements are not affected by letter casing, making your code more robust.
Example 4: Replacing Special Characters
Sometimes, you may encounter strings with special characters that need to be replaced. The str_replace()
function can handle these cases effectively.
<?php
$originalString = "The price is $100!";
$search = "$";
$replace = "USD ";
$newString = str_replace($search, $replace, $originalString);
echo $newString;
?>
Output:
The price is USD 100!
In this example, we replaced the dollar sign with “USD “. This is particularly useful in applications dealing with currency or special formatting, ensuring that your strings are clear and informative.
Conclusion
Replacing strings in PHP is a simple yet powerful tool that can enhance your programming capabilities. The str_replace()
function, along with its case-insensitive counterpart str_ireplace()
, allows for efficient manipulation of text. Whether you’re cleaning user input, formatting data, or altering strings for display, these functions provide the flexibility and control you need. By mastering string replacement, you can write cleaner, more effective PHP code that meets the demands of modern web development.
FAQ
-
What is the difference between str_replace() and str_ireplace()?
str_replace() is case-sensitive, while str_ireplace() performs case-insensitive replacements. -
Can I replace multiple substrings at once using str_replace()?
Yes, you can pass arrays for both search and replace parameters to replace multiple substrings simultaneously. -
What happens if the substring to be replaced is not found?
If the substring is not found, the original string is returned unchanged. -
Is str_replace() suitable for large strings and datasets?
Yes, str_replace() is efficient and can handle large strings and datasets effectively.
- Can I use str_replace() to replace special characters?
Absolutely! str_replace() can replace any character, including special characters, in a string.