PHP での文字列の置換
Minahil Noor
2020年12月21日
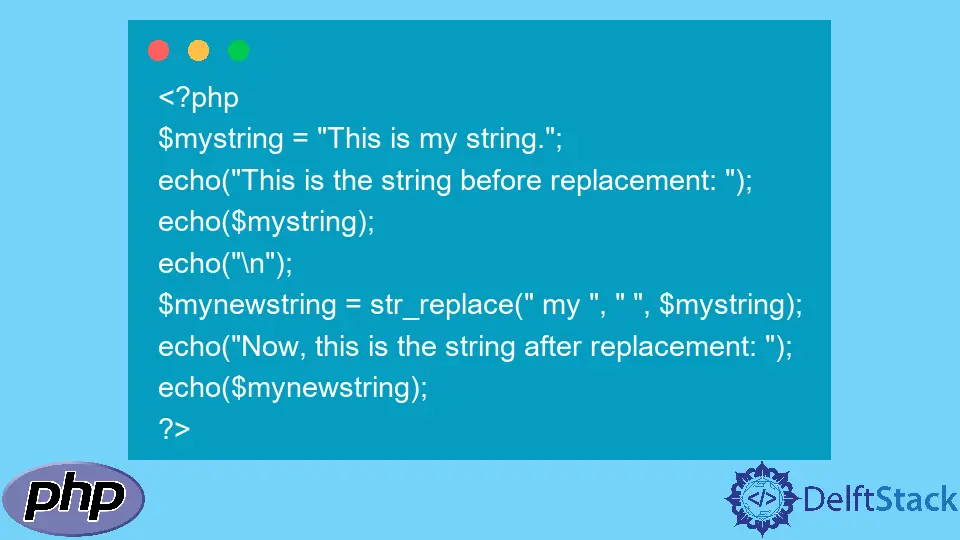
この記事では、PHP で文字列の一部を置換する方法を紹介します。
PHP で文字列の一部を置換するには str_replace()
関数を使用する
PHP では、文字列の一部を置換するための特殊な関数として str_replace()
があります。この関数は、与えられた部分文字列を検索し、与えられた値に置き換えます。この関数を使用するための正しい構文は以下の通りです。
str_replace($search, $replace, $subject, $count);
関数 str_replace()
には 4つのパラメータしかありません。パラメータの詳細は以下の通りです。
パラメータの詳細は以下の通りです。 | 説明 | |
---|---|---|
$search |
強制的 | 与えられた文字列または配列の中で検索したい文字列または配列です。そして、この $search の文字列または配列は、与えられた $replace パラメータで置き換えられます。 |
$replace |
強制的 | これは $search の位置に配置される文字列または配列です。 |
$subject |
強制的 | これは部分文字列が検索され、置換される文字列または配列です。 |
$count |
任意 | 与えられた場合、実行された置換をカウントします。 |
この関数は、置換された文字列または配列を返します。以下のプログラムは、str_replace()
関数を用いて PHP で文字列の一部を置換する方法を示しています。
<?php
$mystring = "This is my string.";
echo("This is the string before replacement: ");
echo($mystring);
echo("\n");
$mynewstring = str_replace(" my ", " ", $mystring);
echo("Now, this is the string after replacement: ");
echo($mynewstring);
?>
出力:
This is the string before replacement: This is my string.
Now, this is the string after replacement: This is string.
この関数は置換された文字列を返します。
パラメータ $count
を渡すと、置換された文字列の数をカウントします。
<?php
$mystring = "This is my string.";
echo("This is the string before replacement: ");
echo($mystring);
echo("\n");
$mynewstring = str_replace(" my ", " ", $mystring, $count);
echo("Now, this is the string after replacement: ");
echo($mynewstring);
echo("\n");
echo("The number of replacements is: ");
echo($count);
?>
出力:
This is the string before replacement: This is my string.
Now, this is the string after replacement: This is string.
The number of replacements is: 1
出力は、この関数が 1つの置換を行ったことを示しています。これは、$search
文字列が渡された文字列の中に一度だけ出現したことを意味します。