How to Find the Date of a Day of the Week in PHP
- Get the Date for a Numerical Day of the Week in PHP
- Get the Day of the Week From a Date String in PHP
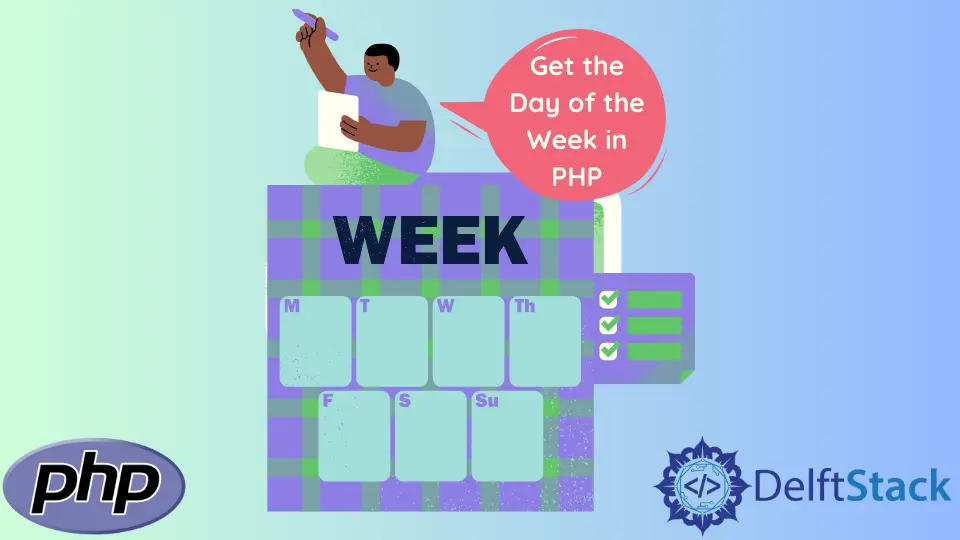
This article teaches you how to get the date of a numerical day of the week using PHP. We base our solution on assigning numbers 0 to 6 to weekdays from Sunday to Saturday.
We’ll use PHP date
and strtotime
functions for our calculations.
Get the Date for a Numerical Day of the Week in PHP
To get the date for a number, we’ll select an initial date from a week. The date will have the format yyyy-mm-dd
, and then we select a number between 0 and 6.
By doing this, we’ll know that any date PHP returns for the number are in the same week as the initial date. Afterward, we use PHP to get the date using our selected number and the initial date.
The PHP code will use the following algorithm:
-
Define the initial date.
-
Choose a number between 0 and 6.
-
Ensure the number falls between 0 and 6.
-
Convert our number to a day of the week using PHP
date
andstrtotime
. -
Convert the day of the week to our date in the format of
yyyy-mm-dd
. We will use thedate()
function and its argument are:Y-m-d
- Unix timestamp of the day of the week from Step 4 subtracted from our chosen number.
- Unix timestamp of our initial date.
The following is the implementation of this algorithm:
<?php
$initial_date = '2022-06-22';
$day = 0;
if ($day >= 0 and $day <= 6) {
$day_of_the_week = date('w', strtotime($initial_date));
$the_date = date('Y-m-d', strtotime(($day - $day_of_the_week). ' day', strtotime($initial_date)));
echo($the_date);
} else {
echo 'You have entered an invalid day value. Check the "$day" variable and try again';
}
?>
Output:
2022-06-19
Get the Day of the Week From a Date String in PHP
When you have the date in the format yyyy-mm-dd
, you can find the day it falls on, e.g., Monday or Sunday. Since we already have a date (from the last section), we can modify our code to print the day name.
The modifications will let us print the full textual representation of the day from the date. We’ll use the date()
function, and we’ll pass the following as its arguments:
- The letter
l
(letter el) - Unix timestamp of our date
The following is the modified code along with the changes from the previous list:
<?php
$the_date = "2022-06-19"; // The date from the previous section
$name_of_the_day = date('l', strtotime($the_date));
echo "The date " . $the_date . " falls on a " . $name_of_the_day;
?>
Output:
The date 2022-06-19 falls on a Sunday
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn