PHP Abstract Class
- What is an Abstract Class in PHP?
- Creating an Abstract Class
- Using Abstract Classes
- Best Practices for Abstract Classes
- Conclusion
- FAQ
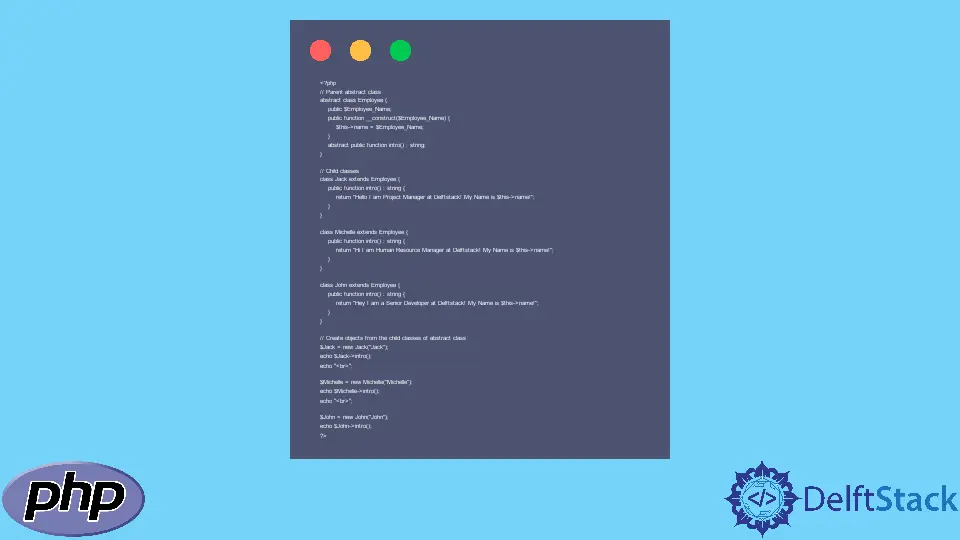
Creating robust and maintainable code is a fundamental aspect of software development. One powerful feature of PHP that aids in this endeavor is the abstract class.
In this tutorial, we will explore how to create and use abstract classes in PHP. By the end of this article, you will understand the purpose of abstract classes, how they differ from regular classes, and the best practices for implementing them in your projects. Whether you are a beginner or an experienced developer, this guide will provide valuable insights into abstract classes in PHP.
What is an Abstract Class in PHP?
An abstract class in PHP serves as a blueprint for other classes. It cannot be instantiated on its own, meaning you cannot create an object directly from it. Instead, abstract classes are intended to be extended by other classes, which must implement the abstract methods defined within the abstract class. This structure allows developers to define a common interface and shared functionality while enforcing specific behaviors in derived classes.
Characteristics of Abstract Classes
- Cannot be instantiated: You cannot create an object from an abstract class directly.
- Can contain abstract methods: These are methods without a body that must be implemented in derived classes.
- Can have concrete methods: Abstract classes can also contain fully defined methods that can be inherited by subclasses.
Creating an Abstract Class
To create an abstract class in PHP, you use the abstract
keyword. Here’s a simple example to illustrate this concept.
<?php
abstract class Animal {
abstract protected function makeSound();
public function sleep() {
return "Sleeping...";
}
}
class Dog extends Animal {
protected function makeSound() {
return "Bark!";
}
}
class Cat extends Animal {
protected function makeSound() {
return "Meow!";
}
}
?>
In this example, we define an abstract class Animal
with an abstract method makeSound
. The sleep
method is a concrete method that can be inherited. The Dog
and Cat
classes extend Animal
and implement the makeSound
method.
Output:
Bark!
Meow!
When you create instances of Dog
and Cat
, you can invoke their makeSound
methods, demonstrating how abstract classes enforce a structure while allowing flexibility in implementation.
Using Abstract Classes
Abstract classes are particularly useful in scenarios where you want to ensure that certain methods are implemented in all subclasses. This is often seen in frameworks and libraries, where a common interface is required.
Example of Using Abstract Classes
Let’s delve deeper into how you can use abstract classes in a more practical scenario. Suppose you are building a simple application that manages different types of vehicles.
<?php
abstract class Vehicle {
abstract protected function startEngine();
public function stopEngine() {
return "Engine stopped.";
}
}
class Car extends Vehicle {
protected function startEngine() {
return "Car engine started.";
}
}
class Motorcycle extends Vehicle {
protected function startEngine() {
return "Motorcycle engine started.";
}
}
$car = new Car();
$motorcycle = new Motorcycle();
echo $car->startEngine();
echo $motorcycle->startEngine();
?>
In this example, the abstract class Vehicle
defines the abstract method startEngine
, which must be implemented by any class that extends it. The Car
and Motorcycle
classes provide their own implementations of startEngine
.
Output:
Car engine started.
Motorcycle engine started.
This structure ensures that any new type of vehicle added in the future will also need to implement the startEngine
method, thus maintaining consistency across your application.
Best Practices for Abstract Classes
When working with abstract classes, there are several best practices to keep in mind:
-
Use Abstract Classes for Shared Behavior: If multiple classes share common functionality, consider using an abstract class to encapsulate that behavior.
-
Keep Abstract Methods to a Minimum: Only define abstract methods that are essential for subclasses. This keeps your abstract class clean and focused.
-
Document Your Code: Since abstract classes serve as a blueprint for other classes, documenting their purpose and usage is crucial for maintainability.
-
Favor Composition Over Inheritance: While inheritance is powerful, sometimes composition is a better approach. Use abstract classes when it makes sense, but also consider other design patterns.
By adhering to these best practices, you can create a more organized and maintainable codebase.
Conclusion
Abstract classes in PHP are a powerful tool for developers, allowing for the creation of flexible and maintainable code. By defining a common interface and enforcing specific behaviors, abstract classes help streamline the development process. As you incorporate abstract classes into your projects, remember to follow best practices to maximize their effectiveness. With a solid understanding of abstract classes, you can enhance the architecture of your PHP applications and improve code reusability.
FAQ
-
What is an abstract class in PHP?
An abstract class is a class that cannot be instantiated on its own and is meant to be extended by other classes. -
Can an abstract class have concrete methods?
Yes, an abstract class can contain both abstract methods (without a body) and concrete methods (with a body). -
How do you define an abstract method?
An abstract method is defined using theabstract
keyword and does not have a body.
-
Can you instantiate an abstract class?
No, you cannot create an object directly from an abstract class. -
What is the purpose of using abstract classes?
Abstract classes allow developers to define a common interface and enforce specific behaviors in derived classes, promoting code reusability and maintainability.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook