PHP 추상 클래스
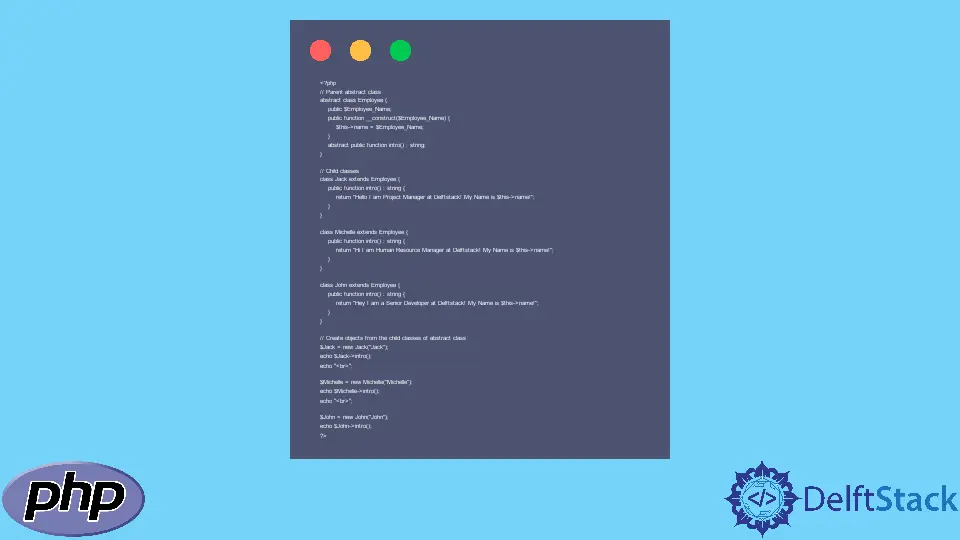
추상 클래스는 하나 이상의 추상 메서드가 있는 클래스입니다. 이 튜토리얼은 PHP에서 추상 클래스를 만들고 사용하는 방법을 보여줍니다.
PHP 추상 클래스
PHP에서 추상 클래스는 C++와 달리 abstract
키워드를 사용하여 선언됩니다. 추상 클래스는 실제 코드가 없는 최소한 하나의 추상 메서드를 포함해야 합니다. 이 메소드에는 abstract
키워드로 선언된 이름과 매개변수만 있습니다.
추상 클래스의 목적은 상속할 템플릿 종류를 제공하고 상속 클래스가 추상 메서드를 구현하도록 하는 것입니다. 추상 클래스는 순수 인터페이스와 일반 클래스 사이의 것입니다.
인터페이스는 모든 메서드가 추상적인 추상 클래스의 특수한 경우일 수도 있습니다. 추상 클래스는 인스턴스화할 수 없으며 클래스에 하나의 추상 메서드가 포함된 경우 해당 클래스는 추상이어야 합니다.
추상 클래스의 구문은 다음과 같습니다.
<?php
abstract class Demo
{
abstract function Method_Name(Parameters);
}
?>
이 구문은 Method_Name
추상 메서드를 사용하여 추상 클래스를 만듭니다.
PHP의 추상 클래스에 대한 예제를 시도해 보겠습니다.
<?php
// Parent abstract class
abstract class Employee {
public $Employee_Name;
public function __construct($Employee_Name) {
$this->name = $Employee_Name;
}
abstract public function intro() : string;
}
// Child classes
class Jack extends Employee {
public function intro() : string {
return "Hello I am Project Manager at Delftstack! My Name is $this->name!";
}
}
class Michelle extends Employee {
public function intro() : string {
return "Hi I am Human Resource Manager at Delftstack! My Name is $this->name!";
}
}
class John extends Employee {
public function intro() : string {
return "Hey I am a Senior Developer at Delftstack! My Name is $this->name!";
}
}
// Create objects from the child classes of abstract class
$Jack = new Jack("Jack");
echo $Jack->intro();
echo "<br>";
$Michelle = new Michelle("Michelle");
echo $Michelle->intro();
echo "<br>";
$John = new John("John");
echo $John->intro();
?>
Jack, Michelle, John은 추상 클래스 Employee
에서 상속받았습니다. 즉, 상속 때문에 Employee
추상 클래스에서 공용 Employee_Name
속성과 공용 __construct
메서드를 사용할 수 있습니다. 그러나 intro()
는 추상 메서드이기 때문에 모든 클래스에서 정의됩니다.
코드에 대한 출력을 참조하십시오.
Hello I am Project Manager at Delftstack! My Name is Jack!
Hi I am Human Resource Manager at Delftstack! My Name is Michelle!
Hey I am a Senior Developer at Delftstack! My Name is John!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook