PHP 抽象类
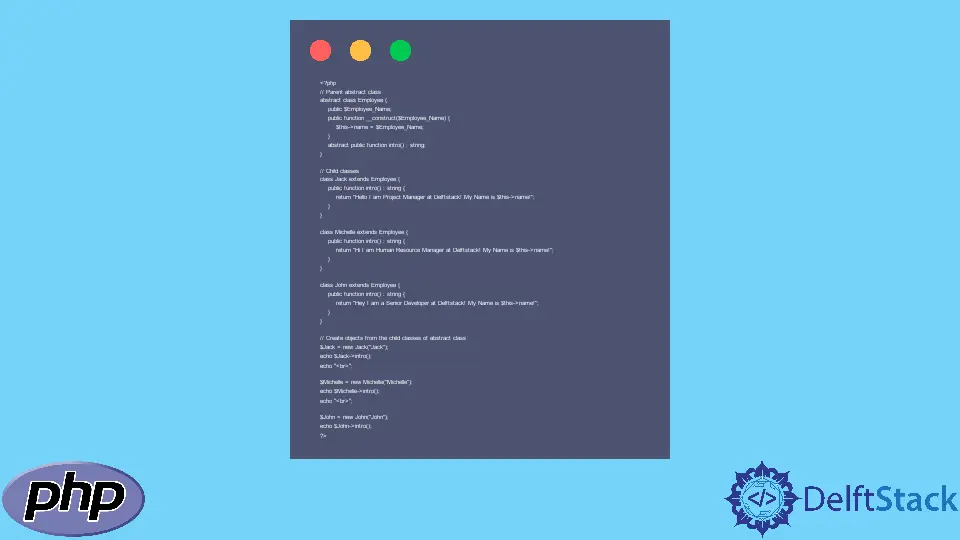
抽象类是具有至少一种抽象方法的类。本教程演示如何在 PHP 中创建和使用抽象类。
PHP 抽象类
在 PHP 中,抽象类是使用 abstract
关键字声明的,这与 C++ 不同。抽象类应该至少包含一个没有任何实际代码的抽象方法;此方法只有用 abstract
关键字声明的名称和参数。
抽象类的目的是提供一种模板来继承并强制继承类实现抽象方法。抽象类介于纯接口和普通类之间。
接口也可以是抽象类的特殊情况,其中每个方法都是抽象的。抽象类永远不能被实例化,如果任何类包含一个抽象方法,则该类必须是抽象的。
抽象类的语法是:
<?php
abstract class Demo
{
abstract function Method_Name(Parameters);
}
?>
此语法使用抽象方法 Method_Name
创建一个抽象类。
让我们尝试一个 PHP 中抽象类的示例:
<?php
// Parent abstract class
abstract class Employee {
public $Employee_Name;
public function __construct($Employee_Name) {
$this->name = $Employee_Name;
}
abstract public function intro() : string;
}
// Child classes
class Jack extends Employee {
public function intro() : string {
return "Hello I am Project Manager at Delftstack! My Name is $this->name!";
}
}
class Michelle extends Employee {
public function intro() : string {
return "Hi I am Human Resource Manager at Delftstack! My Name is $this->name!";
}
}
class John extends Employee {
public function intro() : string {
return "Hey I am a Senior Developer at Delftstack! My Name is $this->name!";
}
}
// Create objects from the child classes of abstract class
$Jack = new Jack("Jack");
echo $Jack->intro();
echo "<br>";
$Michelle = new Michelle("Michelle");
echo $Michelle->intro();
echo "<br>";
$John = new John("John");
echo $John->intro();
?>
Jack、Michelle 和 John 是从抽象类 Employee
继承的,这意味着他们可以使用公共 Employee_Name
属性和 Employee
抽象类的公共 __construct
方法,只是因为继承。但是 intro()
是抽象方法,这就是它在所有类中定义的原因。
查看代码的输出:
Hello I am Project Manager at Delftstack! My Name is Jack!
Hi I am Human Resource Manager at Delftstack! My Name is Michelle!
Hey I am a Senior Developer at Delftstack! My Name is John!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook