在 PHP 中获取类名
- 在 PHP 中使用类名解析来获取类名
-
在 PHP 中使用
__CLASS__
常量获取类名 -
在 PHP 中使用
get_class()
函数获取类名 - PHP 中使用反射类获取类名
-
在 PHP 中在对象上使用
::class
获取类名
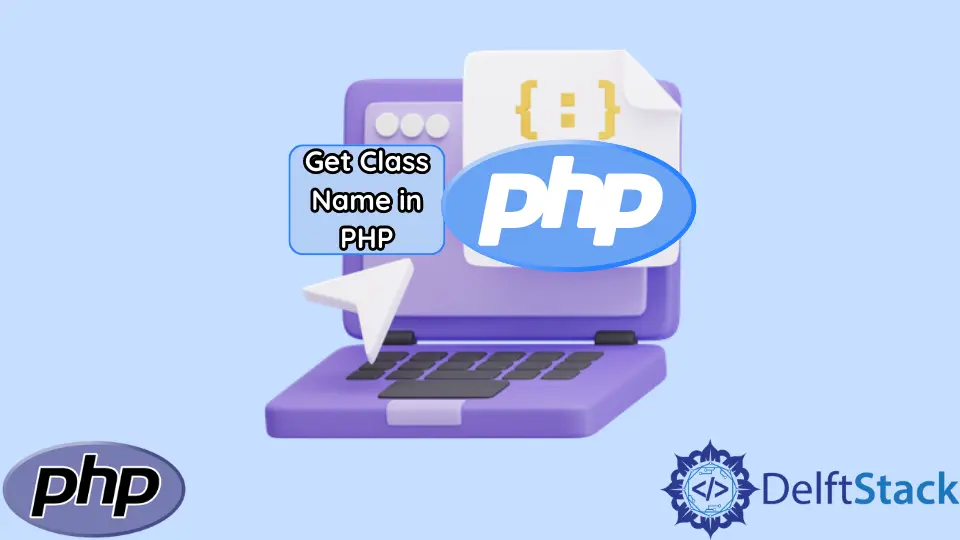
本教程将讨论如何通过类名解析、PHP __CLASS__
常量和 get_class()
方法获取 PHP 类名。你将了解其在类内外的类名解析中的用法。
在 PHP 中使用类名解析来获取类名
当你的代码中有命名空间时,你可以通过类名解析获得类名。结果是一个完全合格的类名 (FQCN)。
此功能在 PHP 中以 ClassName::class
的形式提供。当你的 PHP 中有命名空间时,它很有用。
在下面的代码示例中,通过 ClassName::class
的类名解析将返回关联类的类名:
<?php
namespace YourNameSpace;
// Define a class
class HelloClassName {}
// Get the class name from ::class
echo HelloClassName::class;
?>
输出:
YourNameSpace\HelloClassName
如果你想在类方法中使用这个特性,你可以通过静态方法获取类名。你将把它写成 static::class
。
下一个代码示例展示了如何在类方法中获取类名。
<?php
namespace YourNameSpace;
// Define the class name
class HelloClassName {
/**
* Define a function that returns
* the class name via static::class
*/
public function getClassName() {
return static::class;
}
}
// Create a new object
$hello_class_name = new HelloClassName();
// Get the class name
echo $hello_class_name->getClassName();
?>
输出:
YourNameSpace\HelloClassName
在 PHP 中使用 __CLASS__
常量获取类名
__CLASS__
常量是 PHP 预定义常量的一部分。你可以在类中使用它来获取类名。
以下代码将通过 __CLASS__
常量获取类名。
<?php
// Define the class
class Hello {
// This function will return the
// class name
function HelloName (){
return __CLASS__;
}
}
// Create a new object
$hello_object= new Hello();
// Get the class name
echo $hello_object->HelloName();
?>
输出:
Hello
在 PHP 中使用 get_class()
函数获取类名
PHP 提供了一个 get_class()
函数。该函数将返回类名。你可以在过程和面向对象的编程中使用它。首先,我们来看看程序风格。
下一个代码块定义了一个具有单个函数的类。当参数是 this
关键字时,类中的函数将返回类名。
要获取类名,请从类中创建一个对象;然后,将对象的名称传递给 get_class()
。
<?php
// Define a class
class Hello {
function class_name() {
echo "The class name is: ", get_class($this);
}
}
// Create a new object
$new_hello_object = new Hello();
// Get the class name
$new_hello_object->class_name();
// Also, you can get the class name
// via an external call
// echo get_class($new_hello_object);
?>
输出:
The class name is: Hello
首先,对于 OOP 风格,你可以从静态类返回 get_class()
函数。
<?php
// Define a class
class Hello {
public static function class_name() {
return get_class();
}
}
// Get the class name
$class_name = Hello::class_name();
echo $class_name;
?>
输出:
Hello
此方法有其局限性,因为当你扩展类时,扩展类将返回父类的名称,而不是扩展类。为了解决这个问题,你可以使用 get_call_class()
。
get_call class()
函数依赖于 Late Static Binding。使用此函数,PHP 将返回调用类的名称。它解决了扩展类返回其父类的名称而不是自己的名称的情况。
<?php
// Define a class
class Hello {
// A static function that returns the
// name of the class that calls it
public static function called_class_name() {
return get_called_class();
}
// This function will return the
// defining class name
public static function get_defining_class() {
return get_class();
}
}
class ExtendHello extends Hello {
}
$hello_class_name = Hello::called_class_name();
$extended_class_name = ExtendHello::called_class_name();
$extend_hello_parent_class = ExtendHello::get_defining_class();
echo "Hello class name: " . $hello_class_name . "\n";
echo "Extended Hello class name: " . $extended_class_name . "\n";
echo "Extended Hello parent class name: " . $extend_hello_parent_class . "\n";
?>
输出:
Hello class name: Hello
Extended Hello class name: ExtendHello
Extended Hello parent class name: Hello
PHP 中使用反射类获取类名
反射类是在 PHP 中获取类名的简洁方法。你创建一个类;在这个类中,创建一个返回新反射类的函数。
Reflection 类的参数应该设置为 $this
。之后,你可以通过反射类可用的 getShortName()
方法获取类名。
<?php
// Define a class
class Hello {
// A function that returns the class
// name via reflection
public function HelloClassName() {
return (new \ReflectionClass($this))->getShortName();
}
}
// Create a new class name
$hello_class_name = new Hello();
// Get the class name
echo $hello_class_name->HelloClassName();
?>
输出:
Hello
在 PHP 中在对象上使用 ::class
获取类名
::class
功能适用于 PHP 8 之前的类。从 PHP 开始,当你从类创建对象时,你可以使用 ::class
从创建的对象中获取类名。
你将在以下代码块中找到一个示例。
<?php
namespace YourNameSpace;
// define the class
class HelloClassName {
}
// Create a new object
$hello_class_name = new HelloClassName();
// Get the class name from the object
// via ::class
echo $hello_class_name::class;
?>
输出:
YourNameSpace\HelloClassName
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn