How to Get Class Name in PHP
- Use Class Name Resolution to Get Class Name in PHP
-
Use
__CLASS__
Constant to Get Class Name in PHP -
Use
get_class()
Function to Get Class Name in PHP - Use Reflection Class to Get Class Name in PHP
-
Use
::class
on Object to Get Class Name in PHP
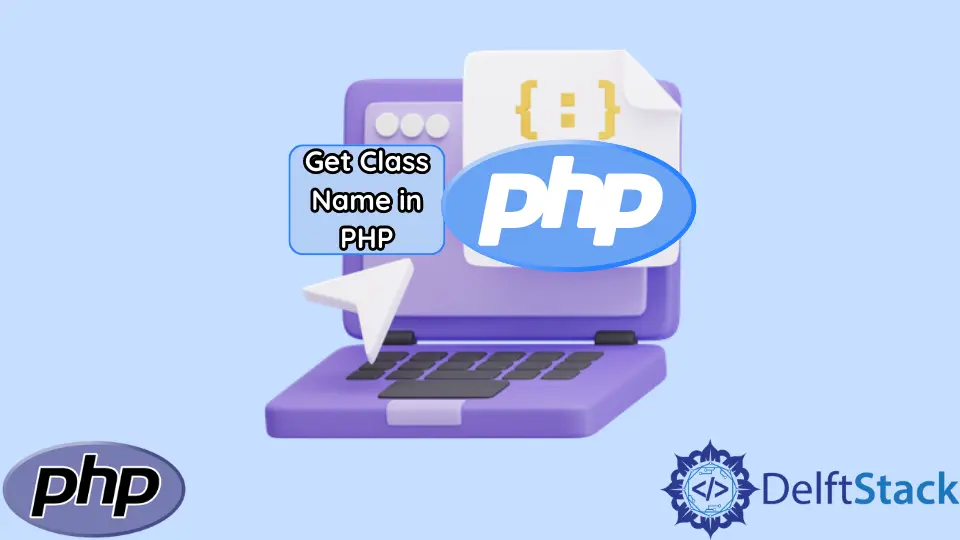
This tutorial will discuss how to get a PHP class name with class name resolution, PHP __CLASS__
constant, and the get_class()
method. You will learn its usage for the class name resolution in and out of a class.
Use Class Name Resolution to Get Class Name in PHP
You can get a class name via class name resolution when you have a namespace in your code. The result is a Fully Qualified Class Name (FQCN).
This feature is available in PHP as ClassName::class
. It is useful when you have a namespace in your PHP.
In the following code example, the class name resolution via ClassName::class
will return the class name of the associated class:
<?php
namespace YourNameSpace;
// Define a class
class HelloClassName {}
// Get the class name from ::class
echo HelloClassName::class;
?>
Output:
YourNameSpace\HelloClassName
If you want to use this feature in a class method, you can get the class name via the static method. You’ll write this as static::class
.
The next code example shows how to get a class name in a class method.
<?php
namespace YourNameSpace;
// Define the class name
class HelloClassName {
/**
* Define a function that returns
* the class name via static::class
*/
public function getClassName() {
return static::class;
}
}
// Create a new object
$hello_class_name = new HelloClassName();
// Get the class name
echo $hello_class_name->getClassName();
?>
Output:
YourNameSpace\HelloClassName
Use __CLASS__
Constant to Get Class Name in PHP
The __CLASS__
constant is part of the PHP predefined constant. You can use it within a class to get a class name.
The following code will get the class name via the __CLASS__
constant.
<?php
// Define the class
class Hello {
// This function will return the
// class name
function HelloName (){
return __CLASS__;
}
}
// Create a new object
$hello_object= new Hello();
// Get the class name
echo $hello_object->HelloName();
?>
Output:
Hello
Use get_class()
Function to Get Class Name in PHP
PHP provides a get_class()
function. This function will return the class name. You can use it in procedural and object-oriented programming. First, we’ll take a look at the procedural style.
The next code block defines a class with a single function. The function within the class will return the class name when its argument is the this
keyword.
To get the class name, you create an object from the class; then, you pass the object’s name to get_class()
.
<?php
// Define a class
class Hello {
function class_name() {
echo "The class name is: ", get_class($this);
}
}
// Create a new object
$new_hello_object = new Hello();
// Get the class name
$new_hello_object->class_name();
// Also, you can get the class name
// via an external call
// echo get_class($new_hello_object);
?>
Output:
The class name is: Hello
At first, for the OOP style, you can return the get_class()
function from a static class.
<?php
// Define a class
class Hello {
public static function class_name() {
return get_class();
}
}
// Get the class name
$class_name = Hello::class_name();
echo $class_name;
?>
Output:
Hello
This method has its limitations because when you extend the class, the extended class will return the name of the parent class and not the extended class. To solve this, you can use get_called_class()
.
The get_called class()
function relies on Late Static Binding. With this function, PHP will return the name of the calling class. It solves the situation where the extended class returns the name of its parent class rather than its own.
<?php
// Define a class
class Hello {
// A static function that returns the
// name of the class that calls it
public static function called_class_name() {
return get_called_class();
}
// This function will return the
// defining class name
public static function get_defining_class() {
return get_class();
}
}
class ExtendHello extends Hello {
}
$hello_class_name = Hello::called_class_name();
$extended_class_name = ExtendHello::called_class_name();
$extend_hello_parent_class = ExtendHello::get_defining_class();
echo "Hello class name: " . $hello_class_name . "\n";
echo "Extended Hello class name: " . $extended_class_name . "\n";
echo "Extended Hello parent class name: " . $extend_hello_parent_class . "\n";
?>
Output:
Hello class name: Hello
Extended Hello class name: ExtendHello
Extended Hello parent class name: Hello
Use Reflection Class to Get Class Name in PHP
Reflection Class is a concise way to get a class name in PHP. You create a class; within this class, create a function that returns a new Reflection class.
The Reflection class should have its argument set to $this
. Afterward, you can get the class name via the getShortName()
method available with the Reflection class.
<?php
// Define a class
class Hello {
// A function that returns the class
// name via reflection
public function HelloClassName() {
return (new \ReflectionClass($this))->getShortName();
}
}
// Create a new class name
$hello_class_name = new Hello();
// Get the class name
echo $hello_class_name->HelloClassName();
?>
Output:
Hello
Use ::class
on Object to Get Class Name in PHP
The ::class
feature worked on classes before PHP 8. Since PHP, when you create an object from a class, you can get the class name from the created object with ::class
.
You’ll find an example in the following code block.
<?php
namespace YourNameSpace;
// define the class
class HelloClassName {
}
// Create a new object
$hello_class_name = new HelloClassName();
// Get the class name from the object
// via ::class
echo $hello_class_name::class;
?>
Output:
YourNameSpace\HelloClassName
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn