How to Use Kronecker Delta in MATLAB
- The Kronecker Delta in MATLAB
- Use Kronecker Delta to Compare Two Symbolic Variables in MATLAB
- Use Kronecker Delta to Compare Symbolic Variable with Zero in MATLAB
- Use Kronecker Delta to Compare Vector of Numbers with Symbolic Variable in MATLAB
- Use Kronecker Delta to Compare Two Matrices in MATLAB
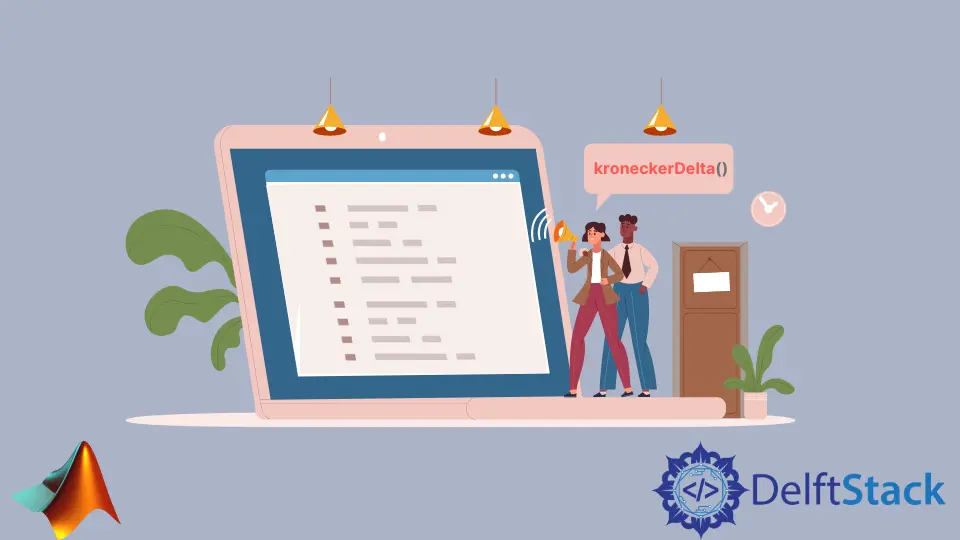
This tutorial demonstrates how to use the kroneckerDelta()
method in MATLAB.
The Kronecker Delta in MATLAB
The Kronecker delta, named after Leopold Kronecker, is a function in mathematics used for two variables: non-negative integers.
MATLAB has a built-in function for the Kronecker delta, kroneckerDelta()
, which has a similar use.
The kroneckerDelta()
method compares and checks for the equality of two variables in MATLAB. It returns 1
or 0
as true or false, respectively.
Use Kronecker Delta to Compare Two Symbolic Variables in MATLAB
Let us test the equality of two equal variables using kroneckerDelta()
in MATLAB.
Example:
syms a b
a = b;
kroneckerDelta(a,b)
syms c d
kroneckerDelta(c,d)
Output:
ans =
1
ans =
kroneckerDelta(c - d, 0)
The code above checks for the equality of variables using kroneckerDelta()
for two equal and two unequal variables.
a
is equal to b
, which is why the kroneckerDelta()
method returns 1
, which means true.
The method kroneckerDelta()
cannot decide if the c
equals d
. It returns the function call with undecidable input.
Use Kronecker Delta to Compare Symbolic Variable with Zero in MATLAB
The method kroneckerDelta()
doesn’t accept the variable type double zero. Hence, we can not directly put 0
.
We use sym
to convert the 0
to an object.
Example:
syms a
a = sym(0);
kroneckerDelta(a)
Output:
ans =
1
Remember that kroneckerDelta(a)
is equal to kroneckerDelta(a,0)
, which means it will compare a
with 0
, which should return 1
.
Use Kronecker Delta to Compare Vector of Numbers with Symbolic Variable in MATLAB
Let’s compare a vector of the number to a symbolic variable. The variable will be compared with each member of the vector.
Example:
Vec = 1:5
syms a
a = sym(5)
answer = kroneckerDelta(Vec,a)
Output:
Vec =
1 2 3 4 5
a =
5
answer =
[ 0, 0, 0, 0, 1]
Use Kronecker Delta to Compare Two Matrices in MATLAB
Both matrices should have equal sizes to compare using kroneckerDelta()
.
Let’s compare two matrices using the kroneckerDelta()
method.
Example:
syms a
X = [a+3 a+1 a+2;a-2 a-1 a]
Y = [a+3 a a+2;a-1 a-1 a]
Answer = kroneckerDelta(X,Y)
Output:
X =
[ a + 3, a + 1, a + 2]
[ a - 2, a - 1, a]
Y =
[ a + 3, a, a + 2]
[ a - 1, a - 1, a]
Answer =
[ 1, 0, 1]
[ 0, 1, 1]
The code above will compare each member of matrix X
with each Y
member in the same position and returns a matrix with the results.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook