MATLAB sscanf() Function
-
Understanding the
sscanf()
Function in MATLAB -
Extract Values From a String Using the
sscanf()
Function in MATLAB - Handling Different Data Types
- Error Handling
- Practical Applications
- Conclusion
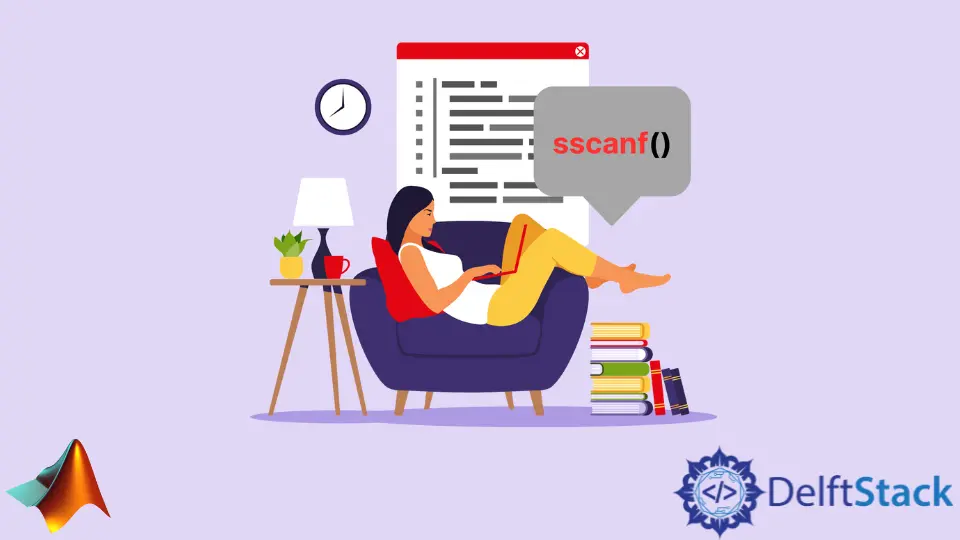
MATLAB, a powerful numerical computing environment, provides a rich set of functions for data manipulation and processing. Among these, the sscanf()
function stands out as a versatile tool for parsing formatted input.
In this tutorial, we will delve into the intricacies of sscanf()
, exploring its syntax, capabilities, and practical applications.
Understanding the sscanf()
Function in MATLAB
The sscanf()
function in MATLAB is designed to scan and extract data from a formatted string. Its name, which stands for "string scan formatted"
, reflects its purpose: it parses a string according to a specified format, extracting data into variables for further use.
Syntax:
[output] = sscanf(input, format)
Here’s what each part of the syntax represents:
output
: This is a variable or an array of variables that will store the extracted data. The number of variables should match the number of conversions specified in the format string.input
: This is the string from which the data will be extracted.format
: This is a string that specifies the format of the input string. It contains format specifiers that define how the data should be interpreted.
Format specifiers are placeholders in the format string that indicate the type and format of the data to be extracted. Some common format specifiers include:
%d
: Integer%f
: Floating-point number%s
: String%c
: Character
These specifiers can also include additional information, such as field width, precision, and more, to customize the parsing process.
Let’s consider a practical example to illustrate how sscanf()
works:
str = 'Age: 30, Height: 175.5, Weight: 70.2';
[age, height, weight] = sscanf(str, 'Age: %d, Height: %f, Weight: %f');
In this example, we have a string str
containing information about age, height, and weight. We use sscanf()
with a format string that matches the pattern in str
.
The extracted values are stored in the variables age
, height
, and weight
.
Extract Values From a String Using the sscanf()
Function in MATLAB
The sscanf()
function scans a string according to a given format in MATLAB. For example, if the given string contains numbers and we want to separate them from each other and save them in a matrix, we can use the sscanf()
function.
The first argument of the sscanf()
function is the input string, and the second argument is the format that we want to use to scan the string, like %d
for numeric values. The third argument is optional and defines the size for the scanning of the string, and it is useful when we do not want to scan the entire string.
If we don’t specify the size, the function will scan the whole string. The sscanf()
function returns four output arguments.
The first output argument is the matrix, which will contain the result of the scanning of the string. The second output argument is optional and returns the number of elements the function has successfully scanned.
The third optional output argument returns the error message that occurred during the scanning of the string. The fourth argument is also optional, and it returns the following index of the position at which the sscanf()
stopped the scanning process.
For example, let’s create a string containing multiple floating-point numbers and separate them using the sscanf()
function in MATLAB.
Code:
clc
clear
My_S = "3.1 2.2 5.2";
num = sscanf(My_S,'%f')
Output:
num =
3.1000
2.2000
5.2000
In the above code, the clc
command is used to clear the MATLAB command window, and the clear
command is used to clean the workspace. As we see in the output, all the floating-point numbers have been separated and saved inside a matrix.
Now, let’s define another string that contains characters and numbers and try to separate the numbers.
Code:
clc
clear
My_S = "3.1 2.2 hello 5.2";
[num, count, msg, index] = sscanf(My_S,'%f')
Output:
num =
3.1000
2.2000
count = 2
msg = sscanf: format failed to match
index = 9
The above code defined four output arguments to store all the outputs from the sscanf()
function. In the above result, the num
variable contains two elements only, but as we can see, there are four numbers in the given string.
The reason is returned in the msg
variable, which says format failed to match
. We set in the above code that we want to scan the floating-point numbers using the %f
symbol, so when the function moves to the non-floating point number, which is the character h
, it stops there.
It returned the error message along with the index, at which it stopped scanning the string, which is 9
in the output.
We can also define the size as the third input argument inside the sscanf()
function. The size is related to the count in the above output; if we define the size as 1
, the function will only read the first element of the string.
We can also define the string as m*n
in the case of a matrix. This is useful if we only want to read a given matrix’s specific row or column.
We can use different kinds of formats to scan the given string, like %d
for signed integer or base 10 numbers, %u
for unsigned integer or base 10 numbers, %o
for octal or base 8 numbers, %x
for hexadecimal or base 16 numbers, and %f
for floating-point numbers.
Handling Different Data Types
One of the strengths of sscanf()
lies in its ability to handle different data types within a single string. For instance, if a string contains both integers and floating-point numbers, sscanf()
can be configured to extract them appropriately.
Error Handling
sscanf()
returns the number of successful conversions. This can be used for error checking to ensure that the expected data was extracted.
If the return value doesn’t match the number of conversions specified in the format string, it indicates a parsing error.
Practical Applications
The sscanf()
function finds extensive use in various domains. Some common applications include:
-
Data Parsing from Files: It can be used to extract specific data from files with known formats, such as log files, CSV files, and more.
-
Text Processing: It is useful for extracting structured information from text data, such as extracting values from sentences or paragraphs.
-
Signal Processing: In applications involving signal processing,
sscanf()
can be used to parse data packets and extract relevant information. -
Custom Data Formats: When dealing with custom data formats,
sscanf()
provides a flexible tool for data extraction.
Conclusion
In conclusion, MATLAB’s sscanf()
function is a powerful tool for extracting structured data from formatted strings. Its versatility and ability to handle different data types within a single string make it a valuable asset in various domains, from data parsing in files to signal processing tasks.
The provided examples demonstrate how sscanf()
can efficiently parse strings, extracting numeric values and handling different formats. Moreover, the function’s error-handling capabilities ensure that unexpected data or formatting issues can be addressed effectively.
By mastering sscanf()
, MATLAB users can streamline data processing tasks, making it an indispensable tool in their toolkit. Whether it’s processing log files, extracting information from text data, or handling custom data formats, sscanf()
proves to be a versatile and invaluable function.