How to Loop Through Vector in MATLAB
-
Loop Through a Vector Using a
for
Loop in MATLAB -
Loop Through a Vector Using a
while
Loop in MATLAB - Loop Through a Vector Using Vectorized Operations in MATLAB
-
Loop Through a Vector Using
arrayfun
andcellfun
in MATLAB - Conclusion
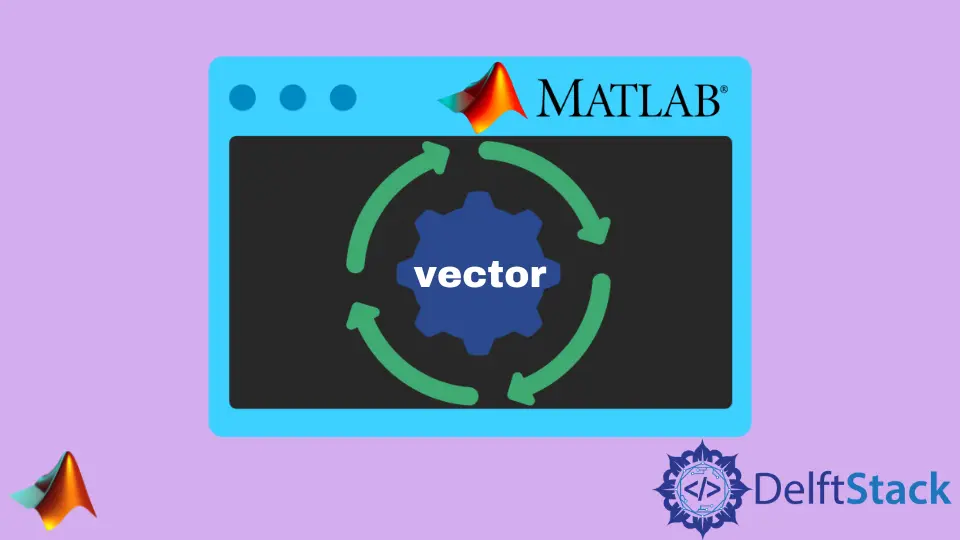
When working with vectors in MATLAB, the need to iterate through each element arises frequently for tasks such as analysis, manipulation, or display. In this article, we’ll explore various methods to loop through a vector in MATLAB, providing insights into the strengths and use cases of each approach.
Loop Through a Vector Using a for
Loop in MATLAB
One common and straightforward method to iterate through each element of a vector is by using a for
loop.
The for
loop in MATLAB is a fundamental control structure that allows you to execute a sequence of statements multiple times. When applied to vectors, it becomes a powerful tool for iterating through each element of the vector and performing operations accordingly.
Code Example:
vector = [1, 2, 3, 4, 5];
for i = 1:length(vector)
disp(vector(i));
end
Output:
1
2
3
4
5
In this example, we start by defining a vector named vector
with arbitrary values. The for
loop is then initiated with this syntax: for i = 1:length(vector)
.
Here, i
is the loop variable that takes on each integer value from 1
to the length of the vector (length(vector)
).
Within the loop, the disp
function is used to display the value of the current element in the vector, accessed by indexing with vector(i)
. As the loop iterates, i
increments, and the corresponding elements of the vector are displayed one by one.
This process continues until the loop completes iteration through all elements of the vector. The loop variable i
acts as an index, ensuring that each element is processed during each iteration.
Loop Through a Vector Using a while
Loop in MATLAB
While the for
loop is a common choice for iterating through vectors in MATLAB, an alternative approach involves using a while
loop.
The while
loop in MATLAB provides a flexible way to iterate through a vector by incrementing an index until a specific condition becomes false. This condition is often based on the length of the vector or other logical criteria.
Unlike the for
loop, which iterates a predetermined number of times, the while
loop continues until a specified condition is no longer true.
Code Example:
vector = [1, 2, 3, 4, 5];
i = 1;
while i <= length(vector)
disp(vector(i));
i = i + 1;
end
Output:
1
2
3
4
5
In this example, we begin by defining a vector named vector
with arbitrary values. To implement the while
loop, we initialize an index variable i
to 1
outside the loop.
The condition while i <= length(vector)
ensures that the loop continues executing as long as the index i
is less than or equal to the length of the vector.
Within the loop, the disp
function is used to display the value of the current element in the vector, accessed by indexing with vector(i)
.
After displaying the current element, the index i
is incremented by 1
(i = i + 1
). This step is crucial to prevent an infinite loop and to ensure that the loop progresses to the next element in the vector.
The loop continues iterating until the condition i <= length(vector)
is no longer true, indicating that we have reached the end of the vector.
Loop Through a Vector Using Vectorized Operations in MATLAB
In MATLAB, vectorized operations stand out as a powerful and efficient way to process entire vectors without explicit looping. Unlike traditional for
or while
loops, vectorized operations leverage MATLAB’s built-in capabilities to perform element-wise operations on entire vectors or matrices, eliminating the need for explicit loops.
This approach is not only more concise but often results in faster and more efficient code execution. Vectorized operations are particularly advantageous when the operations on each element of the vector can be expressed in a single line.
Code Example:
vector = [1, 2, 3, 4, 5];
disp(vector);
Output:
1 2 3 4 5
In this example, we begin by defining a vector named vector
with arbitrary values. Instead of using a loop to iterate through the vector, we employ a vectorized operation to display the entire vector at once.
The disp
function is applied directly to the vector, and MATLAB automatically handles the element-wise display.
This approach is particularly effective for operations that can be performed on each element independently. In the case of displaying a vector, the disp
function acts on the entire vector, and MATLAB internally applies the function to each element, resulting in a concise and efficient code snippet.
Loop Through a Vector Using arrayfun
and cellfun
in MATLAB
The arrayfun
function is another approach we can use to streamline the process of applying a specified function to each element of a regular array, eliminating the need for explicit loops.
It takes a function handle and applies it to each element of the array, returning the results in a new array. This approach aligns with MATLAB’s philosophy of vectorized operations, contributing to cleaner and more readable code.
Code Example:
vector = [1, 2, 3, 4, 5];
arrayfun(@disp, vector);
Output:
1
2
3
4
5
Here, we start by defining a vector named vector
with arbitrary values. Instead of resorting to a conventional loop, we employ arrayfun
to streamline the process.
The syntax arrayfun(@disp, vector)
indicates that we want to apply the disp
function to each element of the vector.
The @disp
syntax denotes a function handle, essentially telling arrayfun
to use the disp
function as the operation to perform on each element. The function is applied element-wise to the entire vector.
As a result, each element is displayed individually, replicating the effect of a loop without the need for explicit indexing or iteration.
arrayfun
proves to be especially valuable when dealing with more complex operations. You can substitute @disp
with any function that takes a single input and customize your vectorized operations accordingly.
Conclusion
In conclusion, the choice of iteration method in MATLAB depends on the specific task and coding preferences. While traditional loops offer explicit control, vectorized operations and arrayfun
provide concise and efficient alternatives.
Understanding these methods equips MATLAB users to navigate vectors with flexibility and efficiency.