How to Iterate Through Matrix in MATLAB
- Iterate Through a Matrix in MATLAB Using Linear Indexing
-
Iterate Through a Matrix in MATLAB Using the
arrayfun()
Function -
Iterate Through a Matrix in MATLAB Using the
cellfun()
Function -
Iterate Through a Matrix in MATLAB Using Nested
for
Loops -
Iterate Through a Matrix in MATLAB Using a
while
Loop - Conclusion
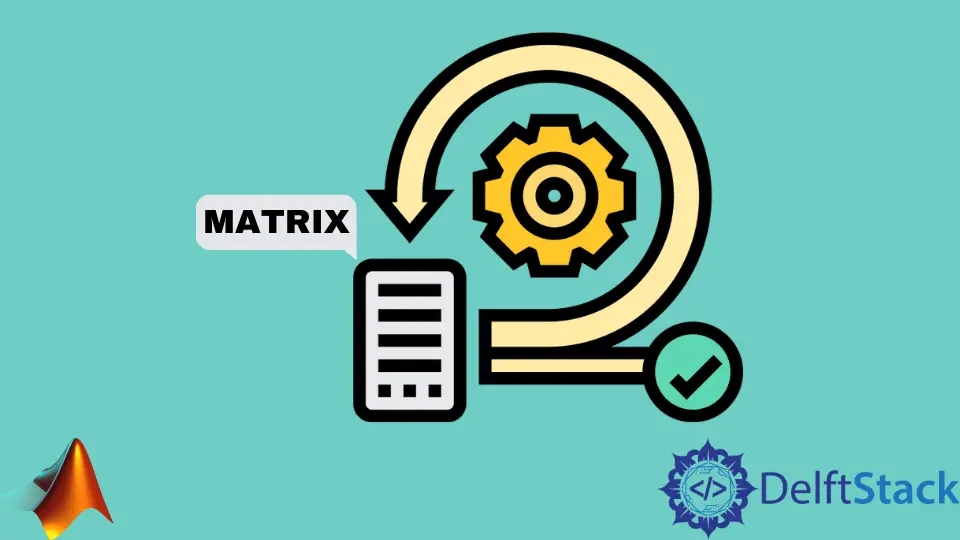
Matrices are at the core of MATLAB, serving as a fundamental data structure for a multitude of applications, from scientific computing to data analysis. Efficiently traversing and manipulating matrices is an important skill for MATLAB users, and the language provides various techniques for matrix iteration.
In this article, we will delve into the different methods available, exploring the syntax, functionality, and optimal use cases for each.
Iterate Through a Matrix in MATLAB Using Linear Indexing
One efficient approach for iterating through matrices in MATLAB is using linear indexing. This method simplifies the iteration process, allowing you to access matrix elements using a single index.
The syntax for linear indexing involves treating a matrix as a one-dimensional array and accessing its elements using a single index. The linear_index
corresponds to the position of the element when the matrix is linearly traversed.
element = matrix(linear_index);
Consider a matrix A
with dimensions m x n
. The linear index k
corresponds to the position of an element in a one-dimensional representation of the matrix.
The relationship between the linear index and the row (i
) and column (j
) indices is given by:
linear_index = (i - 1) * n + j;
This relationship allows MATLAB to navigate through the matrix using a single loop instead of nested loops for rows and columns.
Example: Iterating Through a Matrix Using Linear Indexing
Let’s implement linear indexing with a practical example. Consider the following matrix:
m = [2 6 1; 17 19 18];
Now, let’s iterate through this matrix using linear indexing:
m = [2 6 1; 17 19 18];
for i = 1:numel(m)
disp(m(i));
end
In the above code, we start by defining a matrix m
. The numel()
function is then used to determine the total number of elements in the matrix.
The subsequent loop iterates through each element using linear indexing (m(i)
) and displays it on the command window using disp()
.
This code employs a single loop, thanks to linear indexing, simplifying the iteration process. The loop iterates through the matrix elements in a sequential order dictated by linear indexing.
Code Output:
2
17
6
19
1
18
In this output, each matrix element is displayed in the order dictated by linear indexing.
Iterate Through a Matrix in MATLAB Using the arrayfun()
Function
While linear indexing provides a concise way to iterate through matrices, MATLAB offers additional functionality through functions like arrayfun()
.
The arrayfun()
function is designed to apply a specified function to each element of an array or matrix. The syntax is as follows:
output = arrayfun(function_handle, matrix);
Here, function_handle
represents the function to be applied, and matrix
is the input matrix. The result is stored in the variable output
.
arrayfun()
iterates through each element of the matrix, applying the specified function to each element individually. The function handle provided can be an anonymous function or a predefined function.
Example: Iterating Through a Matrix Using the arrayfun()
Function
Let’s illustrate the use of arrayfun()
with a practical example. Suppose we have a matrix B
:
B = [0 90; 180 270];
Now, let’s apply the cosine
function to each element of this matrix using arrayfun()
:
B = [0 90; 180 270];
output = arrayfun(@(x) cos(x), B);
In this example, we apply the cosine
function to each element of the matrix by invoking arrayfun()
with the function handle @(x) cosd(x)
, where x
represents the input element. The anonymous function cosd(x)
calculates the cosine of the input angle x
in degrees.
As the arrayfun()
iterates through each element of the matrix B
, it applies the specified function to every individual element. The results are then stored in the variable output
, creating a new matrix where each entry corresponds to the cosine of the corresponding element in the original matrix.
Code Output:
output =
1.0000 -0.4481
-0.5985 0.9844
The output displays the result of applying the cosine function to each element of the matrix B
. The values in the output
matrix correspond to the cosine of the angles in degrees present in the original matrix B
.
Iterate Through a Matrix in MATLAB Using the cellfun()
Function
Beyond linear indexing and the versatile arrayfun()
function, MATLAB offers the cellfun()
function for iterating through matrices, particularly when dealing with cell arrays.
The cellfun()
function is designed to apply a specified function to each element of a cell array. The syntax is as follows:
output = cellfun(function_handle, cell_array);
Here, function_handle
represents the function to be applied, and cell_array
is the input cell array. The result is stored in the variable output
.
Similar to arrayfun()
, cellfun()
iterates through each element of the cell array, applying the specified function to each element individually. The function handle provided can be an anonymous function or a predefined function.
Example: Iterating Through a Matrix Using the cellfun()
Function
Let’s illustrate the use of cellfun()
with a practical example. Suppose we have a cell array C
:
C = {0, 90, 180, 270};
Now, let’s apply the sine function to each element of this cell array using cellfun()
:
C = {0, 90, 180, 270};
output = cellfun(@(x) sin(x), C);
disp('output =');
disp(output);
Here, we apply the sine
function to each element of the cell array C
by utilizing cellfun()
with the function handle @(x) sind(x)
, where x
represents the input element. The anonymous function sind(x)
calculates the sine of the input angle x
in degrees.
As cellfun()
iterates through each element of the cell array C
, it applies the specified function to each individual element.
The results are then stored in the variable output
. It creates a new cell array where each entry corresponds to the sine of the corresponding element in the original cell array.
Code Output:
output =
0 0.8940 -0.8012 -0.1760
The output displays the result of applying the sine
function to each element of the cell array C
. The values in the output
cell array correspond to the sine of the angles in degrees present in the original cell array C
.
Iterate Through a Matrix in MATLAB Using Nested for
Loops
While linear indexing, arrayfun()
, and cellfun()
provide efficient ways to iterate through matrices, the classic approach of using nested for
loops is also widely employed in MATLAB.
The syntax for nested for
loops involves having an outer loop control the rows and an inner loop control the columns. The structure looks like this:
% Nested for loops syntax
for i = 1:rows
for j = 1:columns
% Code to be executed for each element (i, j)
end
end
Here, rows
and columns
represent the dimensions of the matrix.
The outer loop (for i = 1:rows
) runs through each row of the matrix, and for each row, the inner loop (for j = 1:columns
) iterates through the columns. This way, every element of the matrix is accessed and processed.
Example: Iterating Through a Matrix Using Nested for
Loops
Let’s illustrate the use of nested for
loops with a practical example. Suppose we have a 3x3 matrix D
:
D = magic(3);
Now, let’s iterate through this matrix using nested for
loops:
D = magic(3);
[rows, columns] = size(D);
for i = 1:rows
for j = 1:columns
element = D(i, j);
disp(element);
end
end
In this example, we extract the dimensions of the matrix using size(D)
, obtaining the values for rows
(number of rows) and columns
(number of columns). The outer loop, controlled by for i = 1:rows
, iterates through each row of the matrix.
Within the outer loop, the inner loop, governed by for j = 1:columns
, iterates through each column of the current row. This structure ensures that each element of the matrix is accessed and processed.
The current element is obtained using indexing (D(i, j)
), and the disp(element)
statement prints each element to the command window. This nested loop process repeats for every element in the matrix, providing a systematic traversal through each row and column.
Code Output:
8
1
6
3
5
7
4
9
2
The output displays the elements of the matrix D
in the order determined by nested for
loops. The sequence matches the linear traversal of the matrix, highlighting how nested for
loops efficiently iterate through each element of a matrix in MATLAB.
Iterate Through a Matrix in MATLAB Using a while
Loop
Iterating through a matrix using a while
loop offers an alternative approach to the more commonly used for
loops. While for
loops are suitable for cases where the number of iterations is known beforehand, while
loops are advantageous when the termination condition is based on a dynamic criterion.
The syntax for a while
loop involves specifying a condition that, when true
, allows the loop to continue iterating. The structure looks like this:
% While loop syntax
while condition
% Code to be executed
end
The loop will continue iterating as long as the specified condition remains true
.
In the context of matrix iteration, a while
loop can be used to traverse the matrix until a specific condition is met. This condition can be related to the current position in the matrix or a specific value within the matrix.
Example: Iterating Through a Matrix Using a while
Loop
Let’s illustrate the use of a while
loop with a practical example. Suppose we have a 3x3 matrix E
:
E = magic(3);
Now, let’s iterate through this matrix using a while
loop until we reach the last element:
E = magic(3);
[m, n] = size(E);
i = 1;
j = 1;
while i <= m
while j <= n
element = E(i, j);
disp(element);
j = j + 1;
end
j = 1;
i = i + 1;
end
Here, we obtain the dimensions m
(rows) and n
(columns) by extracting the size of the matrix using size(E)
. The while
loop is initiated with the loop variables i
and j
set to 1, representing the starting position in the matrix.
The outer while
loop controls the rows, while the inner while
loop controls the columns. Within the inner loop, the current element is accessed using indexing (E(i, j)
), and the disp(element)
statement prints each element to the command window.
The inner loop increments the column index (j
) until it reaches the end of the row. After completing a row, the column index is reset to 1, and the outer loop increments the row index (i
).
This process continues until the entire matrix is traversed.
Code Output:
8
1
6
3
5
7
4
9
2
The output displays the elements of the matrix E
in the order determined by the while
loop. The sequence matches the linear traversal of the matrix, showcasing how a while
loop can efficiently iterate through each element of a matrix in MATLAB.
While while
loops offer flexibility in termination conditions, it’s important to ensure that the loop terminates to prevent infinite iterations.
Conclusion
MATLAB provides a variety of methods for iterating through matrices, each catering to different needs and preferences. We explored several techniques, including concise linear indexing, the arrayfun()
and cellfun()
functions, the classic nested for
loops, and the while
loop.
Whether your preference is for simplicity, efficiency, or flexibility, MATLAB offers a solution for matrix iteration that suits your programming style. By understanding and mastering these various techniques, you can optimize your code for different scenarios, ensuring both flexibility and performance in your MATLAB applications.