How to Flip Image in MATLAB
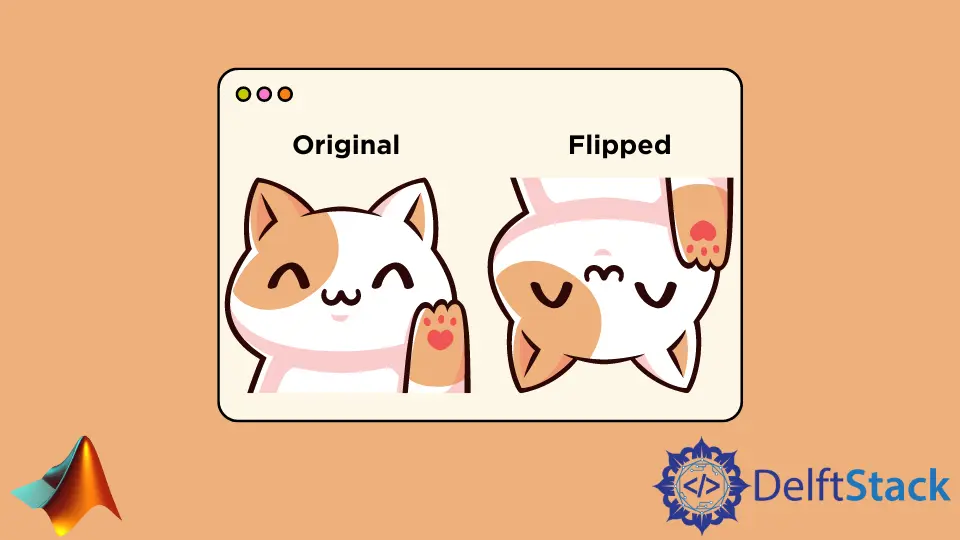
This tutorial will discuss flipping an image vertically using the flip()
function in Matlab.
Flip an Image Using the flip()
Function in MATLAB
Images are composed of pixels. For example, if you have a 200 by 200-pixel image, meaning there are 200 pixels on the vertical axis and 200 pixels on the horizontal axis. We have to read and store the image in Matlab using the imread()
function. It will be stored in a matrix, and each element of the matrix is the value of each pixel of the image. To vertically flip the image, we need to change the position of pixels. For example, the last row of the matrix will become the first row and the first who will become the last row, and so on. We can use the Matlab built-in function flip()
to flip an image or a matrix. For example, let’s flip an image using the flip()
function in Matlab and show them on the same plot using the subplot()
and imshow()
function. See the code below.
img = imread('cat.jpg');
img2 = flip(img);
subplot(1,2,1)
imshow(img)
title('Original Image')
subplot(1,2,2)
imshow(img2)
title('Flipped Image')
Output:
As you can see in the above output, the image is flipped vertically. If we want to flip the image horizontally, we have to add a second argument in the flip()
function, the dimension of the matrix-like flip(img, 2)
. For example, in the above output, we have flipped the matrix column-wise that why the image is vertically flipped, but if we want to flip the image horizontally, we have to flip the matrix row-wise. In Matlab, the first dimension of a matrix is its column and the second dimension is its rows. So to flip the image, we have to pass the dimension as the second argument in the flip()
function. By default, the flip()
function will flip the matrix using the first dimension, which is the column. Some images also have a third dimension which contains the colors in the picture. If we flip the third dimension, the image won’t flip, but the colors of the image will be flipped or changed.
Flip an Image Using Matrix Manipulation in MATLAB
We can also flip a matrix using Matrix manipulation in Matlab. For example, if we want to flip an image using Matrix manipulation instead of the flip()
function, we have to change the flip(img)
function in the above code with the code img(end:-1:1,:,:)
. In this code, we have changed the position of the column entries, and the other two dimensions will remain the same. We have used the colon as the second and third argument so that the second and third dimensions remains the same. We can also change the second dimension. For example, let’s flip the above image vertically as well as horizontally using matrix manipulation in Matlab. See the code below.
img = imread('cat.jpg');
img2 = img(end:-1:1,end:-1:1,:);
subplot(1,2,1)
imshow(img)
title('Original Image')
subplot(1,2,2)
imshow(img2)
title('Flipped Image')
Output:
As you can see in the above output, the image is flipped vertically as well as horizontally. We can only flip one dimension at a time using the flip()
function, but we can flip multiple dimensions using matrix manipulation. We can also flip a matrix using a loop in Matlab.