MATLAB Image Low Pass Filter
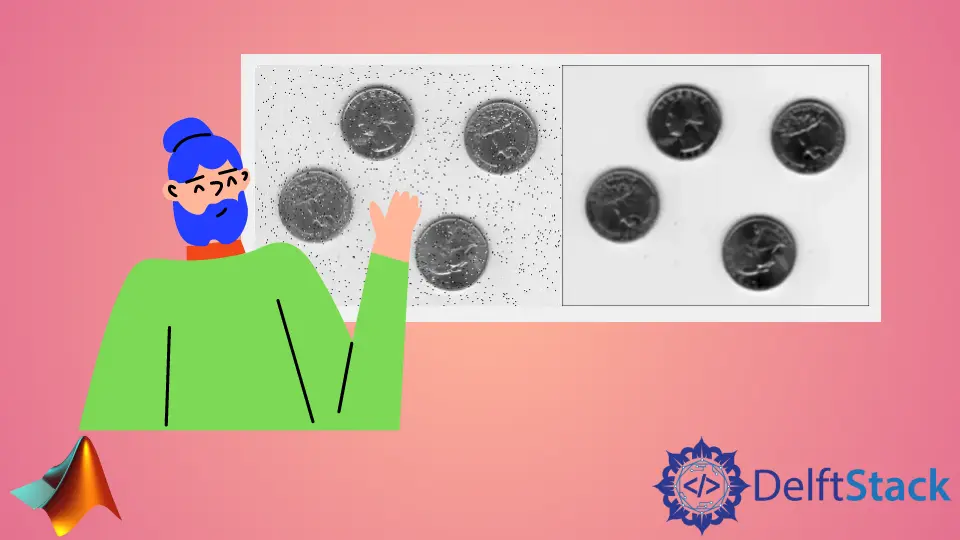
This tutorial will discuss creating a 2D low pass filter for an image using the fspecial()
function in MATLAB.
Smooth an Image Using Low Pass Filter in MATLAB
There are two frequency levels present in an image. The edges and noise in an image represent high-frequency components, and the smooth areas represent low-frequency components.
The high-frequency components have a high-intensity level or high contrast, and the low-frequency components have a low-intensity level or low contrast.
Suppose an image has sharp edges or noise, and we want to smooth it. In that case, we have to create a low pass filter that will allow the low-frequency components or pixels to pass and stop the high-frequency components or pixels, lowering the intensity level of pixels in the image.
Suppose an image has smooth or low contrast areas, and we want to sharpen it. In that case, we have to create a high pass filter that will allow the high-frequency components or pixels to pass and stop the low-frequency components or pixels, increasing the intensity level of pixels in the image.
There are different kinds of filters that we can use to make a low or high-pass filter.
The fspecial()
function of MATLAB can be used to make a 2D low or high pass filter. After creating a filter, we can apply it to the given image using the imfilter()
or filter2()
function.
The fspecial()
function has different syntaxes depending on various filters. The available fspecial()
filters and their syntaxes are shown below.
Filter_object = fspecial(type)
Filter_object = fspecial('average',hsize)
Filter_object = fspecial('disk',radius)
Filter_object = fspecial('gaussian',hsize,sigma)
Filter_object = fspecial('laplacian',alpha)
Filter_object = fspecial('log',hsize,sigma)
Filter_object = fspecial('motion',len,theta)
Filter_object = fspecial('prewitt')
Filter_object = fspecial('sobel')
The first syntax is the basic syntax of the fspecial()
function, and the six filters after the first syntax are low pass filters used to smooth an image, and the last two filters are high pass filters used to sharpen the edges present in the given image. We have to pass the type of the filter or filter name to make a filter of that specific type.
Some filters also have optional arguments, but if we only pass the filter name, the function will use default values for optional arguments. We can also set the optional arguments of filters using their specific syntax, which is shown after the first syntax.
For example, in the case of averaging filter, we can set the filter size, which can be a positive integer or a vector of two elements, using the second syntax listed above.
If we set the hsize
as a positive integer or scaler, the filter will be a square matrix. If we set the hsize
as a vector of two elements, the first element will represent the number of rows, and the second element will represent the number of columns of the filter matrix.
The third syntax is used to create a circular averaging filter or disk filter according to the given radius, which can be a positive integer, and by default, its value is set to 5. The fourth syntax is used to create a Gaussian filter with the size hsize
and sigma
, which represents the standard deviation, and by default, its value is set to 0.5
.
We can also use the imgaussfilt()
and imgaussfilt3()
functions in MATLAB to create and apply a Gaussian filter to a given image. The fifth syntax is used to create a laplacian
filter according to alpha
, which sets the shape of Laplacian, and its default value is 0.2
.
The sixth syntax is used to create a Laplacian filter of gaussian filer according to the filter size hsize
and sigma
, representing standard deviation.
The seventh syntax is used to create a motion filter to smooth an image that contains linear motion of the camera according to the length of the motion specified by len
, which has a default value of 9, and the angle of motion is in degrees specified by theta
. The angle will be in the counterclockwise direction, and its default value is 0.
The eighth syntax creates a high pass filter to enhance the intensity level of horizontal edges using a vertical gradient. If we want to create a filter to enhance the vertical edges, we can take the transpose of the output filter matrix.
The last syntax is the same as the second last syntax; the only difference is that the last syntax is used to create a filter using the smoothing effect. For example, let’s use the fspecial()
function to create the averaging filter to remove salt and pepper noise present in an image.
See the code below.
clc
clear
Input_image = imread('eight.tif');
Noisy_image = imnoise(Input_image,'salt & pepper',0.03);
h = fspecial('average');
Smoothed_image = filter2(h, Input_image);
imshowpair(Noisy_image,Smoothed_image,'montage')
Output:
In the above code, we used the imnoise()
function to put salt and pepper noise in the given image, and we used the filter2()
function to apply the averaging filter to the noisy image. We use the imshowpair()
function to show the noisy and smoothed images for comparison.
We can see in the above output that the right-side image is smoothed using the averaging filter. We can apply different filters to an image to check the result.
We can also use the imfilter()
function to apply a filter on a given image. The difference between filter2()
and imfilter()
is their algorithm to apply the filter on the given image.
imfilter()
uses double precision and floating-point arithmetic to compute the value of pixels of the output image, and filter2()
takes the 2D convolution of the given image and the filter matrix, which will be rotated by 180 degrees to find the output pixel values. We can try both these functions and compare their result.
We can also set some options of the imfilter()
function like the padding options for input array, size of output matrix, and convolution options. We can also set the shape of the output filtered data in case of the filter2()
function, which is set to same
by default, but we can also set it to full
for full 2D filtered data and valid
for data which is computed without using the zero-padded edges.
Check this link for more details about the filter2()
function. Check this link for more details about the imfilter()
function.