How to Resize Image in MATLAB
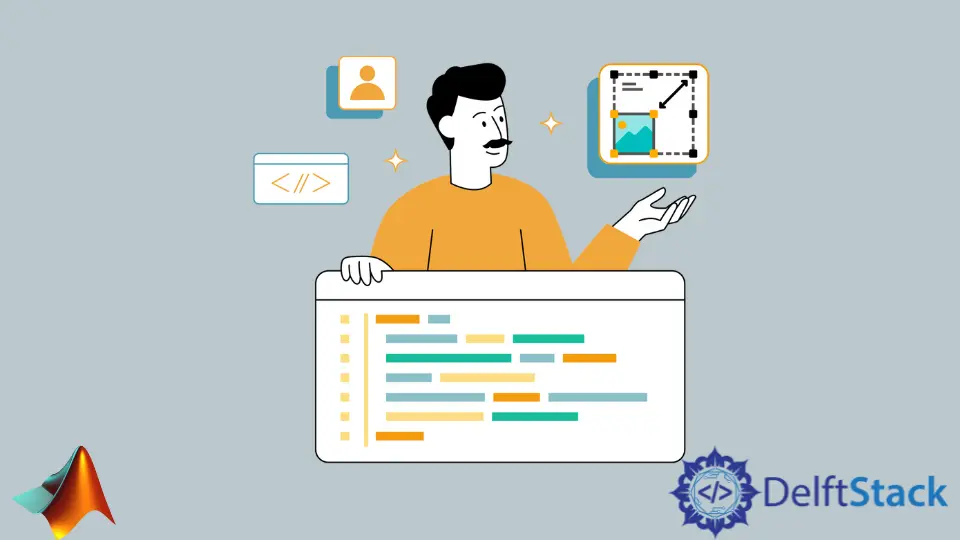
Resizing images is a fundamental task in image processing, whether you’re preparing images for analysis, visualization, or presentation. MATLAB, a widely used platform for numerical computing and data analysis, provides powerful tools for image manipulation, including resizing.
In this article, we’ll explore how to resize images in MATLAB using the built-in function imresize()
and discuss various techniques and considerations.
Resize Images in MATLAB Using the imresize()
Function
The imresize()
function provides a convenient way to adjust image dimensions in MATLAB. This function allows users to adjust the size of images while preserving their aspect ratio and content integrity.
The imresize()
function provides flexibility in specifying the desired dimensions of the output image, allowing users to resize images to a specific size or scale them by a certain factor.
The basic syntax of the imresize()
function is:
B = imresize(A, scale)
Where:
A
is the input image.scale
is the scaling factor. Ifscale
is less than 1, it will resize the image to a smaller size; ifscale
is greater than 1, it will resize the image to a larger size.
Additionally, there are several other syntaxes of the imresize()
function, allowing you to specify the output size, interpolation method, and other parameters.
Here’s a more detailed breakdown of the syntax options:
B = imresize(A, [M N])
B = imresize(A, [M N], method)
B = imresize(A, [M N], method, 'OutputSize', 'crop')
B = imresize(A, [M N], method, 'OutputSize', 'scale')
B = imresize(___, Name, Value)
Where:
[M N]
specifies the output size as a two-element vector[rows cols]
.method
is an optional argument specifying the interpolation method. Possible values include:'nearest'
: Nearest-neighbor interpolation.'bilinear'
: Bilinear interpolation.'bicubic'
: Bicubic interpolation.'box'
: Box interpolation.'triangle'
: Triangle interpolation.'lanczos2'
: Lanczos-2 interpolation.'lanczos3'
: Lanczos-3 interpolation.
'OutputSize', 'crop'
specifies that the output image should be cropped to the specified size.'OutputSize', 'scale'
specifies that the output image should be scaled to fit within the specified size while preserving the aspect ratio.Name, Value
pairs allow you to specify additional parameters such as'Antialiasing'
,'Colormap'
, etc.
The imresize()
function works by interpolating pixel values from the original image to create a new image with the desired dimensions. When enlarging an image, it generates new pixels by interpolating values from neighboring pixels. Conversely, when reducing image size, it removes pixels based on the specified scale factor.
You can type help imresize
in the MATLAB command window for more detailed information on the imresize()
function and its syntax.
Now, let’s walk through some examples demonstrating how to resize images using the imresize()
function in MATLAB.
Example 1: Uniform Resizing Using the imresize()
Function
In this example, we’ll resize an image uniformly by a scale factor of 2.
% Load original image
original_image = imread('cat1.jpg');
% Resize the image
resized_image = imresize(original_image, 10);
% Display original and resized images
figure
imshow(original_image)
title('Original Image')
figure
imshow(resized_image)
title('Resized Image')
Here, we demonstrate uniform resizing of an image by a scale factor of 2
using the imresize()
function.
Initially, we load the original image using imread()
and assign it to the variable original_image
. Then, we resize the image using imresize(original_image, 2)
to increase its dimensions by a factor of 2
.
This creates a new image, resized_image
, with doubled width and height compared to the original. Finally, we display both the original and resized images side by side using imshow()
within a figure with two subplots.
The imshow()
function is used to display each image separately. This example showcases how imresize()
uniformly scales the dimensions of an image.
Output:
Example 2: Resizing to Specific Dimensions Using the imresize()
Function
In this example, we’ll resize an image to specific dimensions, such as 300x300 pixels.
% Load original image
original_image = imread('cat1.jpg');
% Resize the image to specific dimensions
resized_image = imresize(original_image, [300, 300]);
% Display original and resized images
figure
imshow(original_image)
title('Original Image')
figure
imshow(resized_image)
title('Resized Image')
In this example, we demonstrate resizing an image to specific dimensions, in this case, 300x300 pixels.
Similar to Example 1 above, we start by loading the original image using imread()
and store it in the variable original_image
. Next, we use imresize(original_image, [300, 300])
to resize the image to the specified dimensions.
This results in a new image, resized_image
, with dimensions of 300x300 pixels. We then display both the original and resized images side by side in separate figures, similar to Example 1.
This example illustrates how imresize()
can be used to resize images to precise dimensions.
Output:
Example 3: Resizing an Indexed Image Using the imresize()
Function
In this example, we’ll resize an indexed image while preserving its colormap.
% Read indexed image
[indexed_image, colormap] = imread("corn.tif");
% Resize the indexed image
[resized_indexed_image, new_colormap] = imresize(indexed_image, colormap, 2);
% Display original and resized indexed images
figure
imshow(indexed_image, colormap)
title('Original Indexed Image and Colormap')
figure
imshow(resized_indexed_image, new_colormap)
title('Resized Indexed Image and Optimized Colormap')
Here, we demonstrate resizing an indexed image while preserving its colormap.
First, we read the indexed image and its corresponding colormap using imread()
and assign them to the variables indexed_image
and colormap
, respectively. Then, we use imresize(indexed_image, colormap, 2)
to resize the indexed image by a scale factor of 2
while maintaining its colormap.
This results in a new resized indexed image, resized_indexed_image
, and a new colormap, new_colormap
. We display both the original indexed image and the resized indexed image side by side in separate figures.
This example highlights how imresize()
can handle indexed images with colormaps.
Output:
Example 4: Resizing an Image Using the imresize()
Function With Interpolation Methods
In this example, we’ll resize an image while specifying different interpolation methods.
% Load original image
original_image = imread('cat1.jpg');
% Resize the image using different interpolation methods
resized_nearest = imresize(original_image, 0.2, 'nearest');
resized_bilinear = imresize(original_image, 0.3, 'bilinear');
resized_bicubic = imresize(original_image, 0.4, 'bicubic');
% Display original and resized images with different interpolation methods
figure
imshow(original_image)
title('Original Image')
figure
imshow(resized_nearest)
title('Resized (Nearest)')
figure
imshow(resized_bilinear)
title('Resized (Bilinear)')
figure
imshow(resized_bicubic)
title('Resized (Bicubic)')
Here, we show resizing an image using different interpolation methods: nearest neighbor, bilinear, and bicubic.
We start by loading the original image using imread()
and store it in the variable original_image
. Then, we resize the image three times using imresize()
with different interpolation methods: 'nearest'
, 'bilinear'
, and 'bicubic'
.
This creates three resized images: resized_nearest
, resized_bilinear
, and resized_bicubic
, each using a different interpolation method. Finally, we display the original image and the three resized images side by side in separate figures.
Each plot is labeled with the corresponding interpolation method. This example demonstrates how to specify different interpolation methods with imresize()
to achieve varying degrees of image quality and smoothness.
Output:
Example 5: Resizing an RGB Image Using the imresize()
Function
In this example, we’ll resize an RGB image using the imresize()
function in MATLAB.
% Load original RGB image
original_image = imread('peppers.png');
% Resize the RGB image to 50% of its original size
resized_image = imresize(original_image, 0.5);
% Display original and resized images
figure
imshow(original_image)
title('Original RGB Image')
figure
imshow(resized_image)
title('Resized RGB Image (50% of Original Size)')
In this code snippet, we begin by loading the original RGB image named peppers.png
into the variable original_image
using the imread()
function.
Next, we proceed to resize the original RGB image to 50% of its original size. We accomplish this by calling the imresize()
function with two arguments: the original image variable original_image
and the scaling factor 0.5
.
This scaling factor reduces the dimensions of the image by half, effectively resizing it to half of its original width and height. The resulting resized image is stored in the variable resized_image
.
Finally, we display both the original and resized images for comparison purposes. We create two separate figures using the figure
function to accommodate each image.
You can adjust the scaling factor as needed to resize the image to a different size. Additionally, you can experiment with other interpolation methods supported by the imresize()
function for resizing RGB images, such as 'nearest'
, 'bilinear'
, or 'bicubic'
.
Output:
Conclusion
In conclusion, resizing an image in MATLAB is a straightforward process facilitated by the imresize()
function. This function allows us to adjust the dimensions of an image according to our specific requirements, whether it be scaling the image by a certain factor, resizing it to fit within specified dimensions, or applying custom interpolation methods.
By simply loading the original image and applying the desired resizing operation using imresize()
, we can efficiently manipulate image sizes to suit our needs. Additionally, MATLAB provides various options for interpolation methods, aspect ratio preservation, and batch processing, enabling flexibility and customization in resizing images.
Whether working with grayscale, RGB, or indexed images, MATLAB offers a versatile toolkit for image resizing tasks, making it a powerful tool for image processing and analysis. With the ability to resize images seamlessly, MATLAB empowers users to effectively manage and manipulate image data for a wide range of applications, from computer vision and machine learning to scientific research and digital imaging.